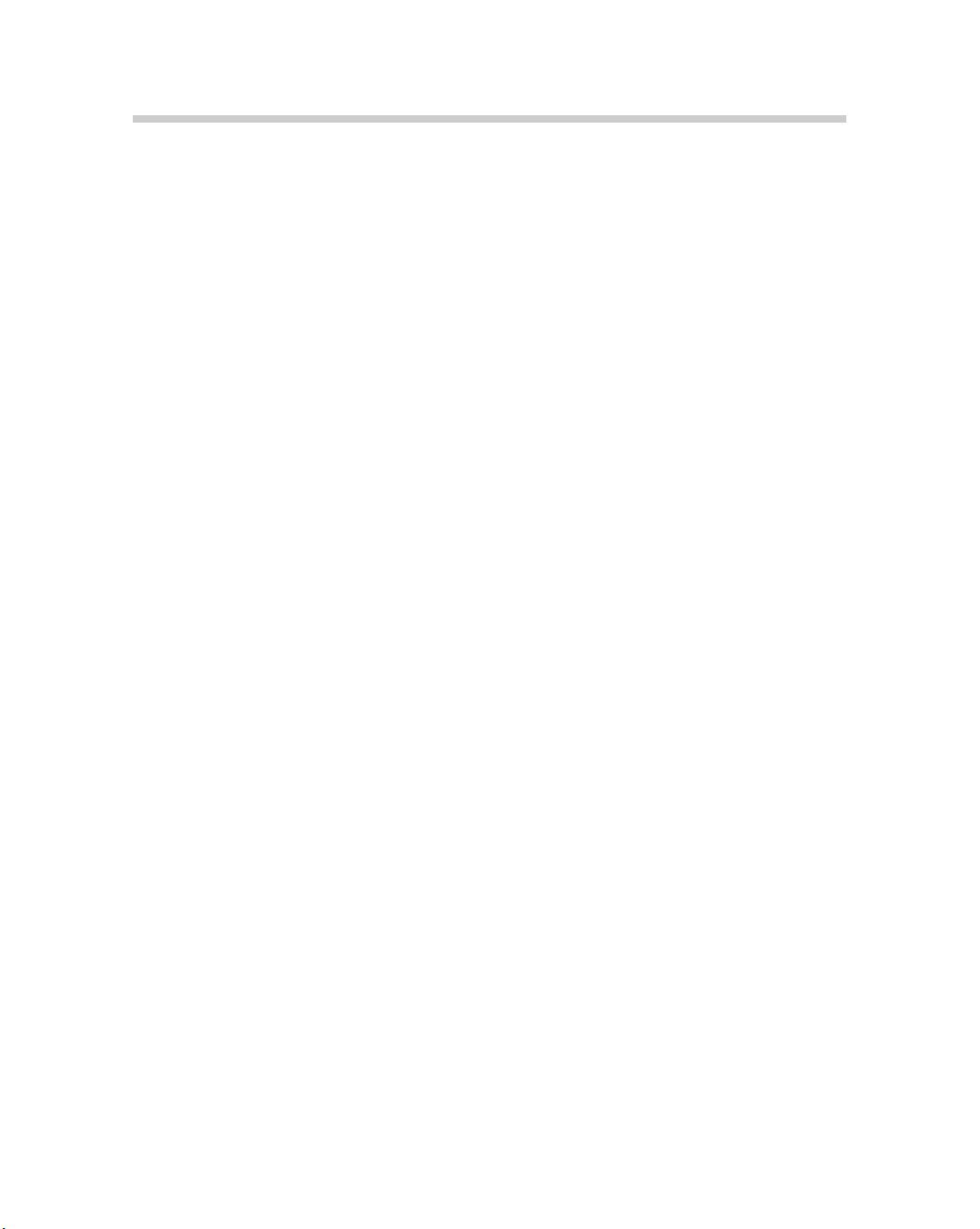
Table of Contents
xv
Pointing with Pointers ................................................................................413
Becoming horribly complex .............................................................413
Pointers to functions .........................................................................418
Pointing a variable to a member function ......................................419
Pointing to static member functions ...............................................422
Referring to References ..............................................................................422
Reference variables ...........................................................................423
Returning a reference from a function ............................................424
Chapter 2: Creating Data Structures. . . . . . . . . . . . . . . . . . . . . . . . . . . .427
Working with Data .......................................................................................427
The great variable roundup ..............................................................427
Expressing variables from either side .............................................429
Casting a spell on your data .............................................................431
Casting safely with C++ ..................................................................... 433
Dynamically casting with dynamic_cast .........................................433
Statically casting with static_cast....................................................437
Structuring Your Data .................................................................................438
Structures as component data types ..............................................439
Equating structures ...........................................................................440
Returning compound data types .....................................................441
Naming Your Space .....................................................................................442
Using variables and part of a namespace .......................................445
Chapter 3: Constructors, Destructors, and Exceptions . . . . . . . . . . . .449
Constructing and Destructing Objects .....................................................449
Overloading constructors .................................................................450
Initializing members ..........................................................................451
Adding a default constructor ...........................................................455
Functional constructors ....................................................................458
Calling one constructor from another ............................................460
Copying instances with copy constructors ....................................461
When constructors go bad: failable constructors? .......................464
Destroying your instances ................................................................465
Virtually inheriting destructors .......................................................466
Programming the Exceptions to the Rule .................................................469
Throwing direct instances ................................................................472
Catching any exception .....................................................................473
Rethrowing an exception ..................................................................474
Chapter 4: Advanced Class Usage . . . . . . . . . . . . . . . . . . . . . . . . . . . . .477
Inherently Inheriting Correctly ..................................................................477
Morphing your inheritance...............................................................477
Adjusting access ................................................................................480
Returning something different, virtually speaking ........................482
Multiple inheritance ..........................................................................486
02_317358-ftoc.indd xv02_317358-ftoc.indd xv 7/22/09 11:15:05 PM7/22/09 11:15:05 PM
More free ebooks : http://fast-file.blogspot.com