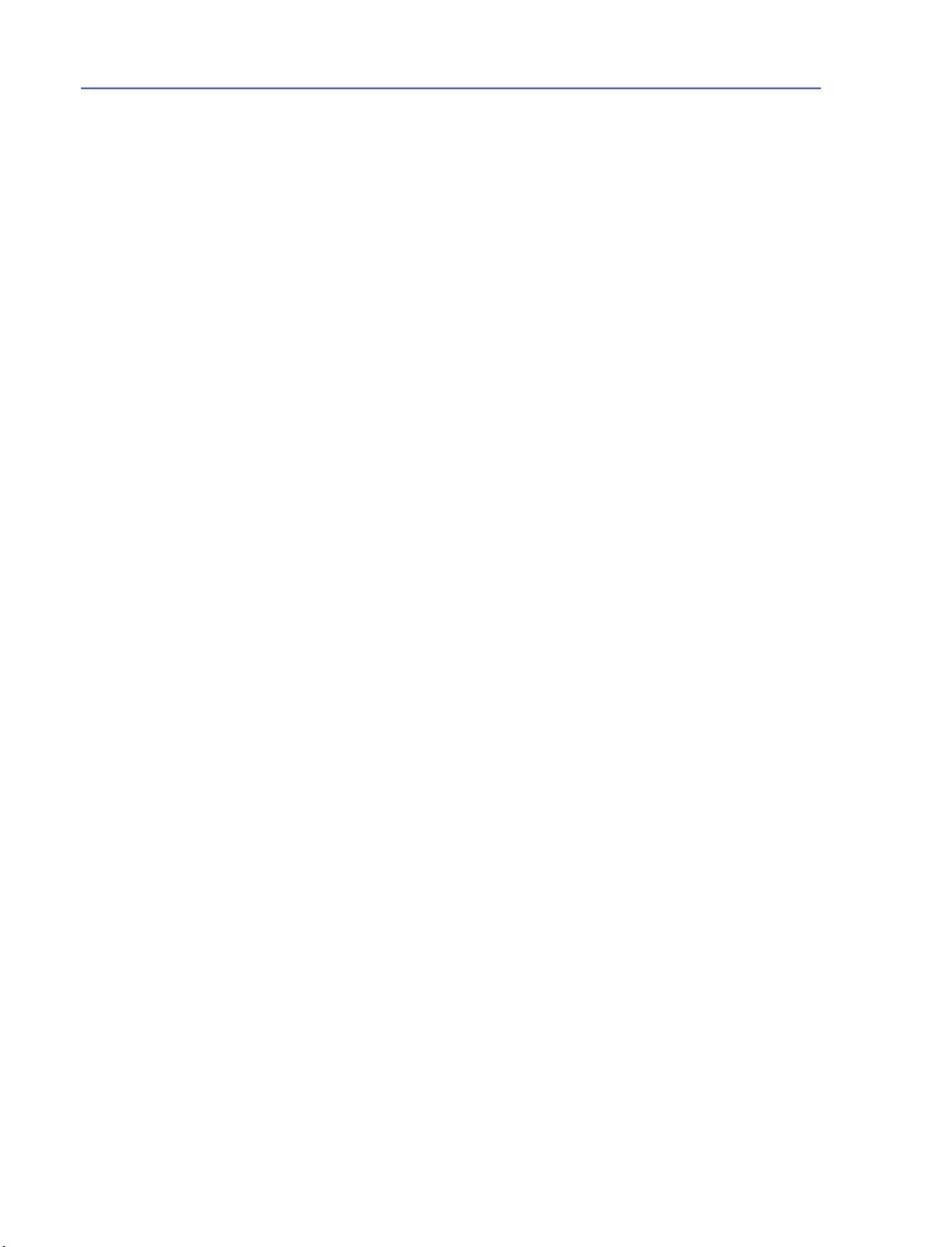
viii
Image Permute . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134
Image Mathematics . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 135
Image Reslice . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137
Iterating through an image . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 138
Chapter 7 Volume Rendering 139
7.1 Historical Note on Supported Data Types. . . . . . . . . . . . . . . . . . . . . . . 140
7.2 A Simple Example . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 140
7.3 Why Multiple Volume Rendering Techniques? . . . . . . . . . . . . . . . . . . 142
7.4 Creating a vtkVolume . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
7.5 Using vtkPiecewiseFunction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
7.6 Using vtkColorTransferFunction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144
7.7 Controlling Color / Opacity with a vtkVolumeProperty. . . . . . . . . . . . 145
7.8 Controlling Shading with a vtkVolumeProperty. . . . . . . . . . . . . . . . . . 147
7.9 Creating a Volume Mapper. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 149
7.10 Cropping a Volume . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 150
7.11 Clipping a Volume . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151
7.12 Controlling the Normal Encoding . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 152
7.13 Volumetric Ray Casting for vtkImageData. . . . . . . . . . . . . . . . . . . . . . 153
7.14 Fixed Point Ray Casting . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
7.15 2D Texture Mapping . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
7.16 3D Texture Mapping . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
7.17 Volumetric Ray Casting for vtkUnstructuredGrid . . . . . . . . . . . . . . . . 157
7.18 ZSweep . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
7.19 Projected Tetrahedra . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159
7.20 Speed vs. Accuracy Trade-offs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159
7.21 Using a vtkLODProp3D to Improve Performance . . . . . . . . . . . . . . . . 161
Chapter 8 Information Visualization 163
8.1 Exploring Relationships in Tabular Data . . . . . . . . . . . . . . . . . . . . . . . 164
Converting a Table to a Graph . . . . . . . . . . . . . . . . . . . . . . . . . . . . 164
Converting a Table to a Tree . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168
8.2 Graph Visualization Techniques . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
Vertex Layout. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 171
Edge Layout . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 172
Converting Layouts to Geometry . . . . . . . . . . . . . . . . . . . . . . . . . . 173
Area Layouts . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 175
8.3 Views and Representations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 176
Selections in Views . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179
8.4 Graph Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 180
Boost Graph Library Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . 182
Creating Graph Algorithms . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 185
The Parallel Boost Graph Library . . . . . . . . . . . . . . . . . . . . . . . . . 186
Multithreaded Graph Library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 186
8.5 Databases. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 187
Connecting to a Database. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 187
Executing Queries . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 188
Queries and Threads. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 189
Reading Results . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 189
Writing Data. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 190
Table Schemata . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 190