c++手写数字识别knn
时间: 2023-08-03 21:39:43 浏览: 119
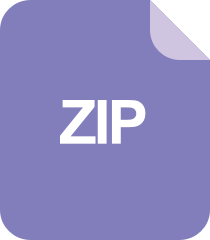
OpenCV和KNN的手写体数字识别项目源码.zip
好的,我可以为您提供一些关于手写数字识别KNN算法的代码实现。
K近邻算法(KNN)是一种基本的机器学习算法,它可以用于分类和回归问题。在数字识别中,KNN可以用于分类问题。以下是实现手写数字识别KNN算法的C++代码示例:
1. 定义一个结构体来表示样本数据。
```c++
struct Sample {
int label; // 标签
vector<int> features; // 特征向量
};
```
2. 定义一个函数来计算两个样本之间的距离。
```c++
double distance(const Sample& a, const Sample& b) {
double dist = 0;
for (int i = 0; i < a.features.size(); i++) {
dist += (a.features[i] - b.features[i]) * (a.features[i] - b.features[i]);
}
return sqrt(dist);
}
```
3. 定义一个函数来预测测试样本的标签。
```c++
int predict(const vector<Sample>& train, const Sample& test, int k) {
vector<pair<double, int>> distances; // 存储距离和标签
for (const auto& sample : train) {
double dist = distance(sample, test);
distances.emplace_back(dist, sample.label);
}
sort(distances.begin(), distances.end()); // 按照距离从小到大排序
unordered_map<int, int> count; // 存储标签和出现次数
for (int i = 0; i < k; i++) {
count[distances[i].second]++;
}
int max_count = 0;
int pred_label = -1;
for (const auto& p : count) {
if (p.second > max_count) {
max_count = p.second;
pred_label = p.first;
}
}
return pred_label;
}
```
4. 加载训练数据和测试数据,并进行预测。
```c++
int main() {
// 加载训练数据
vector<Sample> train;
ifstream train_file("train.txt");
string line;
while (getline(train_file, line)) {
stringstream ss(line);
Sample sample;
ss >> sample.label;
int feature;
while (ss >> feature) {
sample.features.push_back(feature);
}
train.push_back(sample);
}
// 加载测试数据
vector<Sample> test;
ifstream test_file("test.txt");
while (getline(test_file, line)) {
stringstream ss(line);
Sample sample;
ss >> sample.label;
int feature;
while (ss >> feature) {
sample.features.push_back(feature);
}
test.push_back(sample);
}
// 预测测试数据
int k = 5; // K值
int correct_count = 0;
for (const auto& sample : test) {
int pred_label = predict(train, sample, k);
if (pred_label == sample.label) {
correct_count++;
}
}
cout << "Accuracy: " << (double)correct_count / test.size() << endl;
return 0;
}
```
以上就是一个简单的手写数字识别KNN算法的C++实现。需要注意的是,KNN算法的效果很大程度上依赖于选择的特征和K值的大小。希望能对您有所帮助!
阅读全文
相关推荐
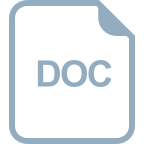
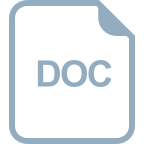

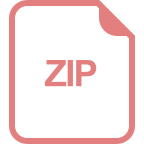
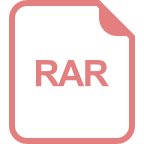
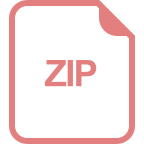
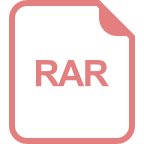
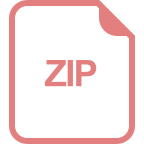
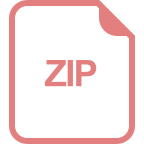
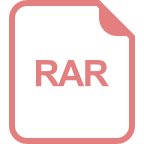
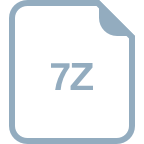
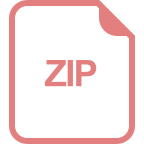
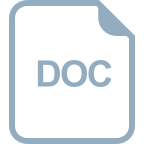



