knn实现手写数字识别c++
时间: 2023-09-03 20:07:15 浏览: 68
对于手写数字识别,K最近邻(K-Nearest Neighbors, KNN)是一种简单而有效的算法。下面是一个用C语言实现KNN手写数字识别的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define K 3 // 设置K值
typedef struct {
int label;
double features[64];
} Sample;
double euclidean_distance(double *a, double *b, int n) {
double sum = 0.0;
for (int i = 0; i < n; i++) {
sum += pow(a[i] - b[i], 2);
}
return sqrt(sum);
}
int knn_predict(Sample *train_set, Sample test_sample, int train_size) {
double distances[train_size];
int labels[K];
// 计算测试样本与所有训练样本之间的距离
for (int i = 0; i < train_size; i++) {
distances[i] = euclidean_distance(train_set[i].features, test_sample.features, 64);
}
// 根据距离排序,获取K个最近邻样本的标签
for (int i = 0; i < K; i++) {
int min_index = 0;
for (int j = 1; j < train_size; j++) {
if (distances[j] < distances[min_index]) {
min_index = j;
}
}
labels[i] = train_set[min_index].label;
distances[min_index] = INFINITY; // 将已选择的样本距离设为无穷大,避免再次选择
}
// 统计最近邻样本中出现次数最多的标签
int counts[10] = {0};
for (int i = 0; i < K; i++) {
counts[labels[i]]++;
}
int max_count = 0;
int predicted_label = 0;
for (int i = 0; i < 10; i++) {
if (counts[i] > max_count) {
max_count = counts[i];
predicted_label = i;
}
}
return predicted_label;
}
int main() {
// 读取训练数据和测试数据
FILE *train_file = fopen("train.txt", "r");
if (train_file == NULL) {
printf("无法打开训练文件\n");
return 1;
}
int train_size;
fscanf(train_file, "%d", &train_size);
Sample *train_set = (Sample *)malloc(train_size * sizeof(Sample));
for (int i = 0; i < train_size; i++) {
fscanf(train_file, "%d", &train_set[i].label);
for (int j = 0; j < 64; j++) {
fscanf(train_file, "%lf", &train_set[i].features[j]);
}
}
fclose(train_file);
FILE *test_file = fopen("test.txt", "r");
if (test_file == NULL) {
printf("无法打开测试文件\n");
return 1;
}
int test_size;
fscanf(test_file, "%d", &test_size);
Sample *test_set = (Sample *)malloc(test_size * sizeof(Sample));
for (int i = 0; i < test_size; i++) {
fscanf(test_file, "%d", &test_set[i].label);
for (int j = 0; j < 64; j++) {
fscanf(test_file, "%lf", &test_set[i].features[j]);
}
}
fclose(test_file);
// 预测并计算准确率
int correct_count = 0;
for (int i = 0; i < test_size; i++) {
int predicted_label = knn_predict(train_set, test_set[i], train_size);
if (predicted_label == test_set[i].label) {
correct_count++;
}
}
double accuracy = (double)correct_count / test_size * 100;
printf("准确率:%lf%%\n", accuracy);
free(train_set);
free(test_set);
return 0;
}
```
以上代码是一个简单的KNN手写数字识别的实现。你需要准备训练数据和测试数据,将其分别保存在"train.txt"和"test.txt"的文本文件中。训练数据和测试数据的格式如下:
```
2000 // 样本数量
5 // 标签
0.1 0.2 ... 0.3 // 特征
...
```
你可以根据实际情况调整K值和特征维度等参数来提高识别精度。希望能对你有所帮助!
相关推荐
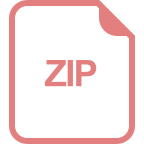














