用JAVA实现diffie-hellman算法
时间: 2023-11-26 14:06:15 浏览: 157
Diffie-Hellman算法是一种密钥交换协议,它可以在不安全的通信信道上协商出一个共享的密钥。下面是用JAVA实现Diffie-Hellman算法的示例代码:
```java
import java.math.BigInteger;
import java.security.SecureRandom;
public class DiffieHellman {
private static final BigInteger P = new BigInteger("FFFFFFFFFFFFFFFFC90FDAA22168C234C4C6628B80DC1CD1"
+ "29024E088A67CC74020BBEA63B139B22514A08798E3404DD"
+ "EF9519B3CD3A431B302B0A6DF25F14374FE1356D6D51C245"
+ "E485B576625E7EC6F44C42E9A637ED6B0BFF5CB6F406B7ED"
+ "EE386BFB5A899FA5AE9F24117C4B1FE649286651ECE65381"
+ "FFFFFFFFFFFFFFFF", 16);
private static final BigInteger G = BigInteger.valueOf(2);
public static void main(String[] args) {
// Alice and Bob generate their private keys
BigInteger a = generatePrivateKey();
BigInteger b = generatePrivateKey();
// Alice and Bob calculate their public keys
BigInteger A = calculatePublicKey(a);
BigInteger B = calculatePublicKey(b);
// Alice and Bob exchange their public keys
BigInteger secretA = calculateSecretKey(a, B);
BigInteger secretB = calculateSecretKey(b, A);
// Check if the shared secrets are equal
if (secretA.equals(secretB)) {
System.out.println("Shared secret: " + secretA);
} else {
System.out.println("Error: shared secrets do not match");
}
}
private static BigInteger generatePrivateKey() {
SecureRandom random = new SecureRandom();
return new BigInteger(256, random);
}
private static BigInteger calculatePublicKey(BigInteger privateKey) {
return G.modPow(privateKey, P);
}
private static BigInteger calculateSecretKey(BigInteger privateKey, BigInteger publicKey) {
return publicKey.modPow(privateKey, P);
}
}
```
该示例代码实现了Diffie-Hellman算法的基本步骤:
1. Alice和Bob生成各自的私钥a和b;
2. Alice和Bob分别使用自己的私钥计算出公钥A和B;
3. Alice和Bob交换公钥;
4. Alice使用自己的私钥和Bob的公钥计算出共享密钥;
5. Bob使用自己的私钥和Alice的公钥计算出共享密钥;
6. Alice和Bob比较计算出的共享密钥是否相同,如果相同则表示密钥交换成功。
在实际应用中,需要注意的是,为了保证安全性,需要选择足够大的素数P和生成元G,以及随机的私钥a和b。
阅读全文
相关推荐
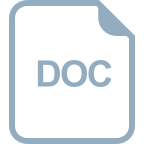
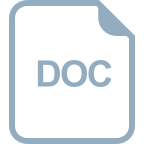
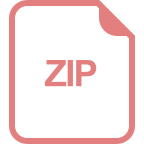
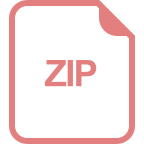
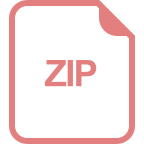
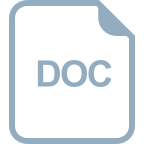
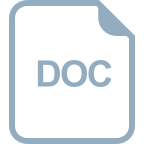
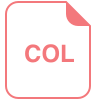
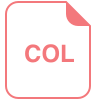




