count = Integer.parseInt(cookie.getValue());的意思
时间: 2024-05-31 13:15:15 浏览: 91
这行代码的意思是将一个字符串类型的cookie值转换为整数类型。具体来说,它使用Integer类的静态方法parseInt(),将cookie.getValue()返回的字符串转换为int类型,并将结果赋值给变量count。如果字符串不能被解析为整数,则会抛出NumberFormatException异常。
相关问题
在Javaweb中用cookie和session购物车代码
可以使用以下代码实现基于cookie和session的购物车功能:
1. 创建一个名为ShoppingCart的Java类,用于存储购物车中的商品信息。
public class ShoppingCart {
private Map<Integer, Integer> items;
public ShoppingCart() {
items = new HashMap<Integer, Integer>();
}
public void addItem(int productId, int quantity) {
if (items.containsKey(productId)) {
int currentQuantity = items.get(productId);
items.put(productId, currentQuantity + quantity);
} else {
items.put(productId, quantity);
}
}
public void removeItem(int productId) {
items.remove(productId);
}
public Map<Integer, Integer> getItems() {
return items;
}
public int getItemCount() {
int count = 0;
for (int quantity : items.values()) {
count += quantity;
}
return count;
}
public double getTotalPrice() {
double totalPrice = 0;
for (Map.Entry<Integer, Integer> entry : items.entrySet()) {
int productId = entry.getKey();
int quantity = entry.getValue();
double price = getProductPrice(productId);
totalPrice += price * quantity;
}
return totalPrice;
}
private double getProductPrice(int productId) {
// TODO: 根据商品ID获取商品价格
return 0;
}
}
2. 在Servlet中使用ShoppingCart类来实现购物车功能。
public class ShoppingCartServlet extends HttpServlet {
private static final String CART_ATTRIBUTE_NAME = "cart";
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession();
ShoppingCart cart = (ShoppingCart) session.getAttribute(CART_ATTRIBUTE_NAME);
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute(CART_ATTRIBUTE_NAME, cart);
}
String action = request.getParameter("action");
if (action != null) {
switch (action) {
case "add":
int productId = Integer.parseInt(request.getParameter("productId"));
int quantity = Integer.parseInt(request.getParameter("quantity"));
cart.addItem(productId, quantity);
break;
case "remove":
int productId = Integer.parseInt(request.getParameter("productId"));
cart.removeItem(productId);
break;
}
}
request.setAttribute("cart", cart);
request.getRequestDispatcher("/shoppingCart.jsp").forward(request, response);
}
}
3. 在JSP页面中显示购物车信息。
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品ID</th>
<th>商品名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach var="item" items="${cart.items}">
<tr>
<td>${item.key}</td>
<td>${getProductName(item.key)}</td>
<td>${getProductPrice(item.key)}</td>
<td>${item.value}</td>
<td>${getProductPrice(item.key) * item.value}</td>
<td><a href="?action=remove&productId=${item.key}">删除</a></td>
</tr>
</c:forEach>
</tbody>
<tfoot>
<tr>
<td colspan="4">总计:</td>
<td>${cart.totalPrice}</td>
<td></td>
</tr>
</tfoot>
</table>
</body>
</html>
注意:在实际开发中,需要根据具体的业务需求来完善购物车功能,例如:添加商品时需要判断库存是否充足,结算时需要进行支付等操作。
jsp写 每次用户访问网页cookie加一
可以通过以下步骤来实现:
1. 在 JSP 页面中获取 cookie 的值,判断是否存在。如果不存在,则创建一个新的 cookie,并将值设置为 1。
```
<%
Cookie[] cookies = request.getCookies();
int count = 0;
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals("visitCount")) {
count = Integer.parseInt(cookie.getValue());
break;
}
}
}
count++;
Cookie cookie = new Cookie("visitCount", String.valueOf(count));
response.addCookie(cookie);
%>
```
2. 在 JSP 页面中显示 cookie 的值。
```
<%
out.print("您是第 " + count + " 次访问本站。");
%>
```
这样,每次用户访问网页时都会将 cookie 的值加一。
阅读全文
相关推荐
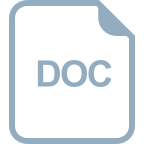
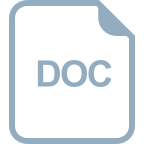
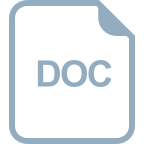
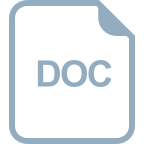
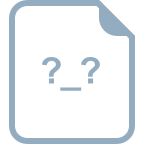







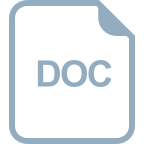
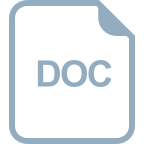