在javaweb中用cookie和session实现一个购物车的代码
时间: 2023-05-10 13:55:29 浏览: 203
以下是一个简单的 JavaWeb 购物车代码,使用了 Cookie 和 Session:
1. 创建一个名为 ShoppingCart 的 Java 类,用于存储购物车中的商品信息:
public class ShoppingCart {
private Map<String, Integer> items = new HashMap<String, Integer>();
public void addItem(String itemId, int quantity) {
if (items.containsKey(itemId)) {
int currentQuantity = items.get(itemId);
items.put(itemId, currentQuantity + quantity);
} else {
items.put(itemId, quantity);
}
}
public void removeItem(String itemId) {
items.remove(itemId);
}
public Map<String, Integer> getItems() {
return items;
}
public int getItemCount() {
int count = 0;
for (int quantity : items.values()) {
count += quantity;
}
return count;
}
public double getTotalPrice() {
double totalPrice = 0;
for (Map.Entry<String, Integer> entry : items.entrySet()) {
String itemId = entry.getKey();
int quantity = entry.getValue();
double price = getItemPrice(itemId);
totalPrice += price * quantity;
}
return totalPrice;
}
private double getItemPrice(String itemId) {
// 根据 itemId 查询商品价格
return 0.0;
}
}
2. 在 Servlet 中使用 Cookie 和 Session 来实现购物车功能:
public class ShoppingCartServlet extends HttpServlet {
private static final String CART_COOKIE_NAME = "cart";
private static final int CART_COOKIE_MAX_AGE = 60 * 60 * 24 * 7; // 一周
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String itemId = request.getParameter("itemId");
int quantity = Integer.parseInt(request.getParameter("quantity"));
// 从 Session 中获取购物车对象
HttpSession session = request.getSession();
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
// 将商品添加到购物车中
cart.addItem(itemId, quantity);
// 将购物车信息保存到 Cookie 中
String cartJson = toJson(cart);
Cookie cartCookie = new Cookie(CART_COOKIE_NAME, cartJson);
cartCookie.setMaxAge(CART_COOKIE_MAX_AGE);
response.addCookie(cartCookie);
// 跳转到购物车页面
response.sendRedirect("cart.jsp");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 从 Cookie 中获取购物车信息
String cartJson = null;
Cookie[] cookies = request.getCookies();
if (cookies != null) {
for (Cookie cookie : cookies) {
if (cookie.getName().equals(CART_COOKIE_NAME)) {
cartJson = cookie.getValue();
break;
}
}
}
// 从 Session 中获取购物车对象
HttpSession session = request.getSession();
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart == null) {
cart = new ShoppingCart();
session.setAttribute("cart", cart);
}
// 如果 Cookie 中有购物车信息,则将其合并到 Session 中的购物车对象中
if (cartJson != null) {
ShoppingCart cookieCart = fromJson(cartJson);
for (Map.Entry<String, Integer> entry : cookieCart.getItems().entrySet()) {
String itemId = entry.getKey();
int quantity = entry.getValue();
cart.addItem(itemId, quantity);
}
}
// 将购物车信息保存到 Cookie 中
String cartJson = toJson(cart);
Cookie cartCookie = new Cookie(CART_COOKIE_NAME, cartJson);
cartCookie.setMaxAge(CART_COOKIE_MAX_AGE);
response.addCookie(cartCookie);
// 跳转到购物车页面
response.sendRedirect("cart.jsp");
}
private String toJson(ShoppingCart cart) {
Gson gson = new Gson();
return gson.toJson(cart);
}
private ShoppingCart fromJson(String json) {
Gson gson = new Gson();
return gson.fromJson(json, ShoppingCart.class);
}
}
3. 在 JSP 页面中显示购物车信息:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品编号</th>
<th>商品名称</th>
<th>单价</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach var="item" items="${cart.items}">
<tr>
<td>${item.key}</td>
<td>${getItemName(item.key)}</td>
<td>${getItemPrice(item.key)}</td>
<td>${item.value}</td>
<td>${getItemPrice(item.key) * item.value}</td>
<td><a href="removeItem?id=${item.key}">删除</a></td>
</tr>
</c:forEach>
</tbody>
<tfoot>
<tr>
<td colspan="4">总计:</td>
<td>${cart.totalPrice}</td>
<td></td>
</tr>
</tfoot>
</table>
</body>
</html>
4. 在 Servlet 中实现删除商品的功能:
public class RemoveItemServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String itemId = request.getParameter("id");
// 从 Session 中获取购物车对象
HttpSession session = request.getSession();
ShoppingCart cart = (ShoppingCart) session.getAttribute("cart");
if (cart != null) {
cart.removeItem(itemId);
}
// 将购物车信息保存到 Cookie 中
String cartJson = toJson(cart);
Cookie cartCookie = new Cookie(CART_COOKIE_NAME, cartJson);
cartCookie.setMaxAge(CART_COOKIE_MAX_AGE);
response.addCookie(cartCookie);
// 跳转到购物车页面
response.sendRedirect("cart.jsp");
}
private String toJson(ShoppingCart cart) {
Gson gson = new Gson();
return gson.toJson(cart);
}
}
注意:以上代码仅供参考,实际应用中需要根据具体需求进行修改和完善。
阅读全文
相关推荐
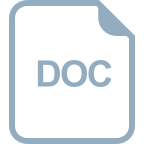
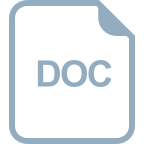
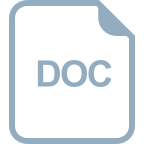

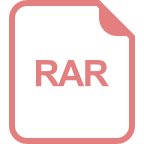
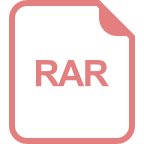
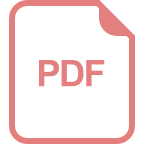
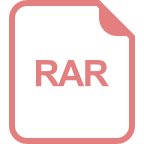
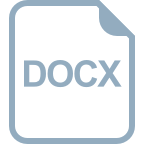
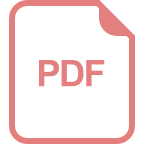
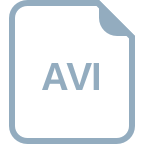