eclipse连接mysql在控制台输入一个学号查找信息,不存在该学号时提示
时间: 2023-12-14 20:38:55 浏览: 54
要实现这个功能,需要使用Java编程语言和JDBC API来连接MySQL数据库,然后编写SQL语句来查询数据。以下是一个简单的示例代码,可以供参考:
```java
import java.sql.*;
public class StudentInfo {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt = null;
ResultSet rs = null;
try {
// 加载MySQL驱动程序
Class.forName("com.mysql.jdbc.Driver");
// 创建数据库连接
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
// 构造SQL语句
String sql = "SELECT * FROM student WHERE id=?";
// 创建PreparedStatement对象
stmt = conn.prepareStatement(sql);
// 设置参数
String id = "1001";
stmt.setString(1, id);
// 执行查询操作
rs = stmt.executeQuery();
// 处理查询结果
if (rs.next()) {
System.out.println("ID: " + rs.getString("id"));
System.out.println("Name: " + rs.getString("name"));
System.out.println("Age: " + rs.getInt("age"));
System.out.println("Gender: " + rs.getString("gender"));
} else {
System.out.println("No student found with ID " + id);
}
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (rs != null) rs.close();
if (stmt != null) stmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
在上述代码中,我们首先加载MySQL驱动程序,然后创建数据库连接。接着,我们构造SQL语句,使用PreparedStatement对象设置查询参数,并执行查询操作。最后,我们根据查询结果输出相应的信息。
如果数据库中不存在指定ID的学生,则会输出“No student found with ID xxx”。
相关推荐
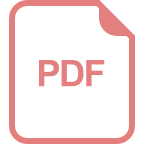
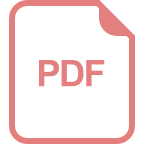
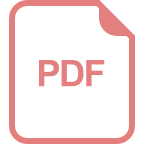














