C# Winform简单模拟水果店结账系统
时间: 2023-07-12 08:02:00 浏览: 90
好的,我可以为您提供一些简单的实现方式。首先,您需要创建一个 Winform 窗体应用程序,然后在窗体上放置一些控件,例如文本框、标签、按钮等。
接下来,您可以创建一个水果类,该类表示水果的名称、单价和数量。然后,您可以在窗体上添加一个 ListBox 控件,用于显示所有添加到购物车的水果。当用户选择一个水果并点击“添加到购物车”按钮时,您可以将该水果添加到 ListBox 控件中。
在购物车中,您需要计算所选水果的总价。为此,您可以在窗体上添加一个标签,用于显示购物车中所选水果的总价。当用户点击“结账”按钮时,您可以将购物车中的所有水果的单价相加,并将结果显示在标签中。
下面是一些基本的代码实现:
```csharp
public class Fruit
{
public string Name { get; set; }
public double Price { get; set; }
public int Quantity { get; set; }
}
public partial class MainForm : Form
{
private List<Fruit> fruits = new List<Fruit>();
public MainForm()
{
InitializeComponent();
}
private void MainForm_Load(object sender, EventArgs e)
{
// 初始化水果列表
fruits.Add(new Fruit() { Name = "苹果", Price = 3.5 });
fruits.Add(new Fruit() { Name = "香蕉", Price = 2.5 });
fruits.Add(new Fruit() { Name = "橙子", Price = 4.0 });
// 绑定水果列表到 ListBox 控件
fruitListBox.DataSource = fruits;
fruitListBox.DisplayMember = "Name";
}
private void addButton_Click(object sender, EventArgs e)
{
// 获取所选水果
Fruit selectedFruit = (Fruit)fruitListBox.SelectedItem;
// 更新水果数量
selectedFruit.Quantity += (int)quantityNumericUpDown.Value;
// 添加到购物车
cartListBox.Items.Add(selectedFruit.Name);
// 更新总价
double total = GetTotalPrice();
totalPriceLabel.Text = total.ToString("C");
}
private void checkoutButton_Click(object sender, EventArgs e)
{
// 显示结账信息
double total = GetTotalPrice();
MessageBox.Show($"总价:{total.ToString("C")}", "结账信息", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
private double GetTotalPrice()
{
double total = 0.0;
// 计算总价
foreach (var fruit in fruits)
{
total += fruit.Price * fruit.Quantity;
}
return total;
}
}
```
以上代码仅提供一种简单的思路,您可以根据实际需求进行修改和扩展。
相关推荐
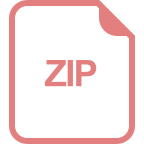
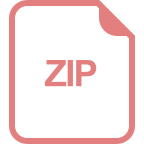














