假设给出了一个CEPS 数据库数据,用Python完成下列问题: (1)读取学生问卷数据, 'data2013\cepsw1studentEN.dta',提取其中的'ids'(学生 id), 'clsids' (学生所在班级 id), 'tr_chn', 'tr_mat','tr_eng','a01','a03', 'a06','c18','b01','a15','b06', 'b07', 'b09', 'c12'等列,完成相关数据清洗工作: ①删除含有缺失值的行(any)。 ②'tr_chn', 'tr_mat','tr_eng'分别表示被调查学生的语文、数学和英语成绩,请添加一个新列计 算三门课程的总分, 记为 Y_score。 ③请结合’七年级学生问卷 2013.pdf’ 、’七年级学生问卷 2013En.pdf’ , 请选择适当的归纳方 式,将'a01','a03','a06','c18', 'b01', 'a15', 'b06','b07','b09', 'c12'等列归纳到 0/1 虚拟变量,【例如 'a03'中, 'The Han nationality'记为 1,其他所有答案记为 0;'b06'表示妈妈的受教育水平,将 'Senior high school degree', 'Junior college degree', 'Bachelor degree', 'Master degree or higher'记 为 1,其余答案记为 0】,并注意这些列的名称需设定为有一定含义的中文或英文。 ④展示清洗完成的表格, 删除多余的列, 最终结果包含【'ids','clsids', ‘Y_score’, 和'a01','a03', 'a06', 'c18', 'b01', 'a15', 'b06', 'b07', 'b09', 'c12'调整后的结果】共计 13 列。
时间: 2023-08-18 12:06:20 浏览: 186
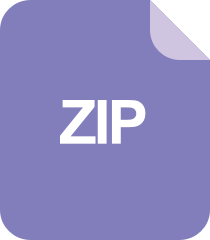
利用Python数据分析
好的,我会按照您的要求回答这个问题。首先,需要使用 Pandas 库来读取和处理数据,以下是完整的代码:
```python
import pandas as pd
# 读取数据
data = pd.read_stata('data2013/cepsw1studentEN.dta')
# 提取需要的列
cols = ['ids', 'clsids', 'tr_chn', 'tr_mat', 'tr_eng', 'a01', 'a03', 'a06', 'c18', 'b01', 'a15', 'b06', 'b07', 'b09', 'c12']
data = data[cols]
# 删除缺失值
data.dropna(inplace=True)
# 计算总分
data['Y_score'] = data[['tr_chn', 'tr_mat', 'tr_eng']].sum(axis=1)
# 归纳虚拟变量
data['Gender'] = data['a01'].apply(lambda x: 1 if x == 'Male' else 0)
data['Nationality'] = data['a03'].apply(lambda x: 1 if x == 'The Han nationality' else 0)
data['Only Child'] = data['a06'].apply(lambda x: 1 if x == 'Yes' else 0)
data['Father Education'] = data['c18'].apply(lambda x: 1 if x == 'Master degree or higher' else (2 if x == 'Bachelor degree' else (3 if x == 'Junior college degree' else 4)))
data['Mother Education'] = data['b01'].apply(lambda x: 1 if x == 'Master degree or higher' else (2 if x == 'Bachelor degree' else (3 if x == 'Junior college degree' else 4)))
data['Family Income'] = data['a15'].apply(lambda x: 1 if x == 'Above 20000' else (2 if x == '15001-20000' else (3 if x == '10001-15000' else (4 if x == '5001-10000' else 5))))
data['Mother Job'] = data['b06'].apply(lambda x: 1 if x == 'Professional and technical personnel' else (2 if x == 'Civil servants' else (3 if x == 'Workers' else (4 if x == 'Farmers' else (5 if x == 'Unemployed' else 6))))))
data['Father Job'] = data['b07'].apply(lambda x: 1 if x == 'Professional and technical personnel' else (2 if x == 'Civil servants' else (3 if x == 'Workers' else (4 if x == 'Farmers' else (5 if x == 'Unemployed' else 6))))))
data['Before School'] = data['b09'].apply(lambda x: 1 if x == 'Yes' else 0)
data['Health Status'] = data['c12'].apply(lambda x: 1 if x == 'Good' else 0)
# 删除多余的列
drop_cols = ['tr_chn', 'tr_mat', 'tr_eng', 'a01', 'a03', 'a06', 'c18', 'b01', 'a15', 'b06', 'b07', 'b09', 'c12']
data.drop(drop_cols, axis=1, inplace=True)
# 展示结果
print(data)
```
这段代码首先读取了数据,然后提取了需要的列。接着,使用 `dropna()` 方法删除含有缺失值的行。然后,计算了三门课程的总分,并添加了一个名为 `Y_score` 的新列。最后,将一些列归纳为虚拟变量,并将所有结果展示出来。
阅读全文
相关推荐

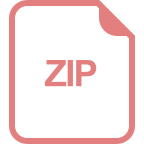
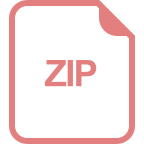
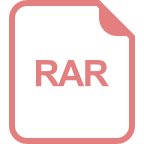
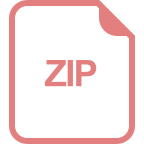
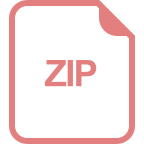
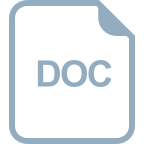
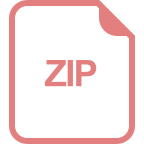
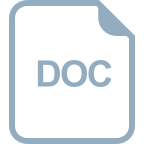
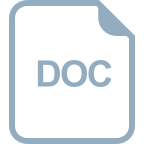




