怎么用PreparedStatement在Java中进行数据库的更新,数据库包含五列分别是int类型的id和Alexa,以及string类型的url,name,country,请以package Mysql.java; import java.sql.*; //mysqld --console class Conn { Connection con; public Connection getConnection() { try { Class.forName("com.mysql.jdbc.Driver"); System.out.println("数据库驱动加载成功"); } catch (ClassNotFoundException e) { e.printStackTrace(); } try { con = DriverManager.getConnection("jdbc:mysql://localhost:3306/test_demo", "root", "66666666"); System.out.println("数据库连接成功"); } catch (SQLException e) { e.printStackTrace(); } return con; } } public class JdbcTest { public static void main(String[] args) { Connection conn = null; Statement stmt = null; try { Conn c = new Conn(); conn = c.getConnection(); //添加 PreparedStatement的更新操作 conn.close(); } catch (SQLException se) { // 处理 JDBC 错误 se.printStackTrace(); } catch (Exception e) { // 处理 Class.forName 错误 e.printStackTrace(); } finally { // 关闭资源 try { if (stmt != null) stmt.close(); } catch (SQLException se2) { }// 什么都不做 try { if (conn != null) conn.close(); } catch (SQLException se) { se.printStackTrace(); } } } }为基础给出简洁的语段
时间: 2024-02-10 22:08:28 浏览: 21
可以使用以下代码示例在Java中使用PreparedStatement进行数据库的更新:
```java
String updateQuery = "UPDATE table_name SET Alexa = ?, url = ?, name = ?, country = ? WHERE id = ?";
PreparedStatement pstmt = conn.prepareStatement(updateQuery);
pstmt.setInt(1, alexa);
pstmt.setString(2, url);
pstmt.setString(3, name);
pstmt.setString(4, country);
pstmt.setInt(5, id);
int rowsAffected = pstmt.executeUpdate();
```
其中,updateQuery为更新语句,使用占位符(?)表示待填充的参数。通过调用PreparedStatement对象的set方法,将参数的值填充到对应位置。最后调用executeUpdate方法执行更新操作,并返回受影响的行数。需要注意的是,需要保证填充参数的顺序与updateQuery中占位符的顺序一致。
相关问题
怎么在Java中增删改查数据库的内容,我的数据库里有五列,两列的数据是int还有三列是string,请给我具体的代码
好的,以下是Java使用JDBC进行增删改查操作的示例代码:
```
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class DatabaseOperation {
// 数据库连接相关信息
private static final String URL = "jdbc:mysql://localhost:3306/test?useSSL=false&serverTimezone=UTC";
private static final String USERNAME = "root";
private static final String PASSWORD = "password";
// 插入数据
public static void insertData(int col1, String col2, int col3, String col4, String col5) throws SQLException {
Connection conn = null;
PreparedStatement pstmt = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
// 构造SQL语句,使用?占位符
String sql = "INSERT INTO tablename (col1, col2, col3, col4, col5) VALUES (?, ?, ?, ?, ?)";
// 创建PreparedStatement对象,预编译SQL语句
pstmt = conn.prepareStatement(sql);
// 设置占位符的值
pstmt.setInt(1, col1);
pstmt.setString(2, col2);
pstmt.setInt(3, col3);
pstmt.setString(4, col4);
pstmt.setString(5, col5);
// 执行SQL语句,返回受影响的行数
int rows = pstmt.executeUpdate();
System.out.println("插入了" + rows + "行数据");
} finally {
// 关闭连接和Statement对象
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
// 查询数据
public static void queryData(int id) throws SQLException {
Connection conn = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
// 构造SQL语句,使用?占位符
String sql = "SELECT * FROM tablename WHERE id = ?";
// 创建PreparedStatement对象,预编译SQL语句
pstmt = conn.prepareStatement(sql);
// 设置占位符的值
pstmt.setInt(1, id);
// 执行SQL语句,返回查询结果
rs = pstmt.executeQuery();
// 处理查询结果
while (rs.next()) {
int col1 = rs.getInt("col1");
String col2 = rs.getString("col2");
int col3 = rs.getInt("col3");
String col4 = rs.getString("col4");
String col5 = rs.getString("col5");
System.out.println(col1 + "\t" + col2 + "\t" + col3 + "\t" + col4 + "\t" + col5);
}
} finally {
// 关闭连接、Statement对象和ResultSet对象
if (rs != null) {
rs.close();
}
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
// 更新数据
public static void updateData(int id, int col1, String col2, int col3, String col4, String col5) throws SQLException {
Connection conn = null;
PreparedStatement pstmt = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
// 构造SQL语句,使用?占位符
String sql = "UPDATE tablename SET col1 = ?, col2 = ?, col3 = ?, col4 = ?, col5 = ? WHERE id = ?";
// 创建PreparedStatement对象,预编译SQL语句
pstmt = conn.prepareStatement(sql);
// 设置占位符的值
pstmt.setInt(1, col1);
pstmt.setString(2, col2);
pstmt.setInt(3, col3);
pstmt.setString(4, col4);
pstmt.setString(5, col5);
pstmt.setInt(6, id);
// 执行SQL语句,返回受影响的行数
int rows = pstmt.executeUpdate();
System.out.println("更新了" + rows + "行数据");
} finally {
// 关闭连接和Statement对象
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
// 删除数据
public static void deleteData(int id) throws SQLException {
Connection conn = null;
PreparedStatement pstmt = null;
try {
// 获取数据库连接
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
// 构造SQL语句,使用?占位符
String sql = "DELETE FROM tablename WHERE id = ?";
// 创建PreparedStatement对象,预编译SQL语句
pstmt = conn.prepareStatement(sql);
// 设置占位符的值
pstmt.setInt(1, id);
// 执行SQL语句,返回受影响的行数
int rows = pstmt.executeUpdate();
System.out.println("删除了" + rows + "行数据");
} finally {
// 关闭连接和Statement对象
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
}
}
}
```
使用时,只需要将其中的`tablename`替换为实际的表名,调用相应的方法即可。例如,插入一条数据:
```
DatabaseOperation.insertData(1, "hello", 2, "world", "!");
```
其中的数字和字符串是具体的列值。查询一条数据:
```
DatabaseOperation.queryData(1);
```
其中的数字是主键id的值。更新一条数据:
```
DatabaseOperation.updateData(1, 3, "hi", 4, "there", ".");
```
其中的数字和字符串是需要更新的列值,还需要指定主键id的值。删除一条数据:
```
DatabaseOperation.deleteData(1);
```
其中的数字是主键id的值。
数据库datatime类型在Java是什么类型
在 Java 中,与数据库中的 datetime 类型对应的是 java.sql.Timestamp 类型。Timestamp 类型可以用来表示日期和时间,包括年、月、日、时、分、秒以及纳秒。
你可以使用 java.sql.Timestamp 类型来处理数据库中的 datetime 类型数据。可以通过以下方式进行操作:
1. 从数据库中获取 datetime 类型的值时,可以使用 ResultSet 的 getTimestamp 方法获取 Timestamp 对象。例如:
```java
ResultSet resultSet = statement.executeQuery("SELECT my_datetime_column FROM my_table");
while (resultSet.next()) {
Timestamp timestamp = resultSet.getTimestamp("my_datetime_column");
// 进行你的操作
}
```
2. 在向数据库中插入或更新 datetime 类型的值时,可以使用 PreparedStatement 的 setTimestamp 方法设置 Timestamp 对象。例如:
```java
PreparedStatement preparedStatement = connection.prepareStatement("INSERT INTO my_table (my_datetime_column) VALUES (?)");
Timestamp timestamp = new Timestamp(System.currentTimeMillis()); // 使用当前时间作为示例
preparedStatement.setTimestamp(1, timestamp);
preparedStatement.executeUpdate();
```
请注意,java.sql.Timestamp 类型是 java.util.Date 类的子类,因此可以将 Timestamp 对象直接用于需要 java.util.Date 类型的地方。
需要注意的是,如果你只需要表示日期或时间部分而不需要纳秒精度,也可以使用 java.sql.Date 或 java.sql.Time 类型。这些类型分别用于表示日期和时间,但没有纳秒精度。
相关推荐
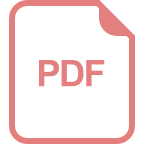
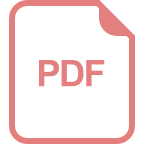
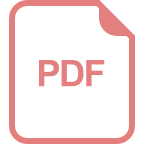












