3.请问作为特征提取器使用VGG和Resnet哪个更好?为什么?
时间: 2024-04-09 13:31:29 浏览: 158
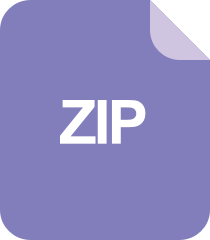
深度学习作业-基于resnet50和vgg16网络pytorch框架实现猫狗分类完整源码+代码注释+实验报告.zip
VGG和ResNet都是在计算机视觉领域中非常重要的深度学习模型,但在特征提取方面,ResNet通常更好,原因如下:
1. 模型深度: ResNet相对于VGG具有更深的网络结构。深层网络可以提取更丰富和复杂的特征表示。通过引入残差连接,ResNet能够有效地解决梯度消失和梯度爆炸的问题,使得更深的网络能够训练并保持较好的性能。
2. 参数效率: ResNet使用了残差块的结构,这种结构允许网络跳过一些层,只需要学习残差部分,而不是学习整个变换。这样可以减少了需要训练的参数数量,提高了参数的效率。
3. 性能表现: 大量的研究表明,在许多计算机视觉任务中,ResNet相对于VGG具有更好的性能。ResNet在ILSVRC 2015图像分类挑战赛中取得了第一名,证明了其在图像分类任务上的优越性能。
尽管ResNet在特征提取方面通常更好,但在某些特定任务或数据集上,VGG可能也能表现出色。因此,在选择模型时,最好根据具体任务和数据集的特点进行评估和选择。
阅读全文
相关推荐
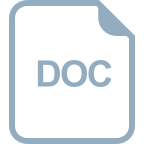
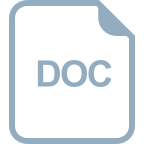
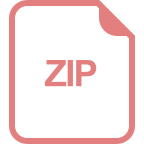
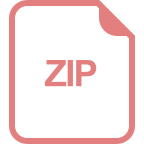
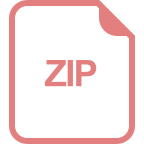
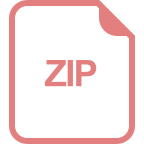
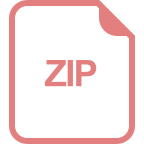
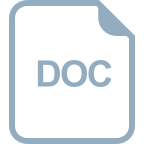
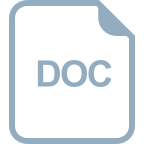
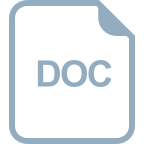
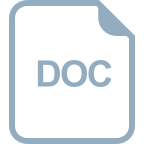
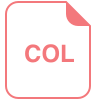





