用c语言写一个电子点餐系统
时间: 2023-11-23 09:06:53 浏览: 30
好的,电子点餐系统是一个比较复杂的系统,需要考虑到很多方面,比如菜品信息的存储、订单的生成和管理、支付和结账等等。以下是一个简单的电子点餐系统的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_DISHES 100
typedef struct dish {
char name[50];
float price;
int quantity;
} Dish;
Dish dishes[MAX_DISHES];
int num_dishes = 0;
void add_dish() {
Dish new_dish;
printf("Enter the name of the dish: ");
scanf("%s", new_dish.name);
printf("Enter the price of the dish: ");
scanf("%f", &new_dish.price);
printf("Enter the quantity of the dish: ");
scanf("%d", &new_dish.quantity);
dishes[num_dishes] = new_dish;
num_dishes++;
}
void view_dishes() {
printf("Dishes available:\n");
for (int i = 0; i < num_dishes; i++) {
printf("%s - $%.2f - %d available\n", dishes[i].name, dishes[i].price, dishes[i].quantity);
}
}
void place_order() {
int order[MAX_DISHES] = {0};
float total = 0;
printf("Enter the number of dishes you want to order: ");
int num_orders;
scanf("%d", &num_orders);
for (int i = 0; i < num_orders; i++) {
printf("Enter the name of dish #%d: ", i+1);
char dish_name[50];
scanf("%s", dish_name);
for (int j = 0; j < num_dishes; j++) {
if (strcmp(dishes[j].name, dish_name) == 0) {
printf("Enter the quantity of dish #%d: ", i+1);
int quantity;
scanf("%d", &quantity);
if (quantity > dishes[j].quantity) {
printf("Sorry, we don't have enough %s. Please choose a smaller quantity.\n", dishes[j].name);
} else {
order[j] = quantity;
total += quantity * dishes[j].price;
}
break;
}
}
}
printf("Your order:\n");
for (int i = 0; i < num_dishes; i++) {
if (order[i] > 0) {
printf("%dx %s - $%.2f\n", order[i], dishes[i].name, dishes[i].price);
}
}
printf("Total: $%.2f\n", total);
}
int main() {
int choice;
do {
printf("1. Add a new dish\n");
printf("2. View dishes available\n");
printf("3. Place an order\n");
printf("4. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_dish();
break;
case 2:
view_dishes();
break;
case 3:
place_order();
break;
case 4:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice!\n");
break;
}
} while (choice != 4);
return 0;
}
```
这个程序使用了结构体来存储菜品信息,包括名称、价格和数量。用户可以选择添加新菜品、查看现有菜品和下订单。在下订单的过程中,程序会提示用户输入想要点的菜品名称和数量,并且会检查库存是否充足。如果库存不够,程序会提示用户重新输入。最后,程序会输出订单的详细信息和总价。
相关推荐
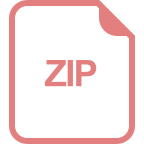
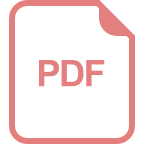
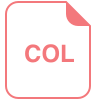
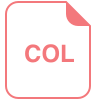
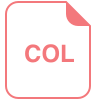
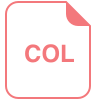
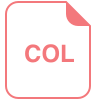









