如何中断js的foreach循环
时间: 2023-10-18 10:21:21 浏览: 46
在JavaScript中,forEach循环无法中断,但可以通过其他方式模拟中断循环的效果。以下是一些方法:
1. 使用some方法:some方法会在数组中找到第一个满足条件的元素后停止迭代,可以将forEach循环的回调函数改为some方法的回调函数,以达到中断循环的效果。
```javascript
let arr = [1, 2, 3, 4, 5];
let isBreak = false;
arr.some((item) => {
if (item === 3) {
isBreak = true;
return true;
}
console.log(item);
});
```
2. 使用for循环:使用传统的for循环可以通过break语句中断循环。
```javascript
let arr = [1, 2, 3, 4, 5];
for (let i = 0; i < arr.length; i++) {
if (arr[i] === 3) {
break;
}
console.log(arr[i]);
}
```
3. 使用try-catch语句:在循环中使用try-catch语句,当需要中断循环时,抛出一个异常并在catch语句中捕获。
```javascript
let arr = [1, 2, 3, 4, 5];
try {
arr.forEach((item) => {
if (item === 3) {
throw new Error('BreakException');
}
console.log(item);
});
} catch(e) {
if (e.message !== 'BreakException') throw e;
}
```
相关推荐
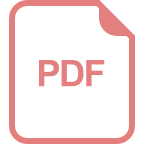
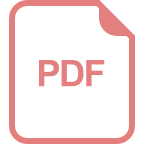














