使用inotify获取usb设备插拔事件,并提取出设备类型,名字,pid vid 文件路径等信息,不使用libudev,请用C语言写出程序
时间: 2024-02-27 13:54:53 浏览: 200
好的,以下是使用inotify获取usb设备插拔事件并提取设备信息的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/inotify.h>
#include <string.h>
#define EVENT_SIZE (sizeof(struct inotify_event))
#define BUF_LEN (1024 * (EVENT_SIZE + 16))
int main(void)
{
int fd, wd;
char buf[BUF_LEN];
int len, i = 0;
struct inotify_event *event;
fd = inotify_init();
if (fd < 0) {
perror("inotify_init");
return EXIT_FAILURE;
}
wd = inotify_add_watch(fd, "/dev/", IN_CREATE | IN_DELETE);
if (wd < 0) {
perror("inotify_add_watch");
return EXIT_FAILURE;
}
while (1) {
len = read(fd, buf, BUF_LEN);
if (len < 0) {
perror("read");
return EXIT_FAILURE;
}
i = 0;
while (i < len) {
event = (struct inotify_event *)&buf[i];
if (event->mask & (IN_CREATE | IN_DELETE)) {
if (strstr(event->name, "usb")) {
char sys_path[256];
char vid[5], pid[5];
char *devname;
FILE *fp;
sprintf(sys_path, "/sys/class/%s/%s/device/uevent", event->name, event->name);
fp = fopen(sys_path, "r");
if (fp == NULL) {
perror("fopen");
return EXIT_FAILURE;
}
while (fgets(buf, sizeof(buf), fp)) {
if (strstr(buf, "PRODUCT=")) {
sscanf(buf, "PRODUCT=%4s/%4s/", vid, pid);
} else if (strstr(buf, "DEVNAME=")) {
buf[strlen(buf) - 1] = '\0';
devname = strrchr(buf, '/');
printf("Device %s %s (VID=%s PID=%s)\n", event->mask & IN_CREATE ? "added" : "removed", devname + 1, vid, pid);
}
}
fclose(fp);
}
}
i += EVENT_SIZE + event->len;
}
}
inotify_rm_watch(fd, wd);
close(fd);
return EXIT_SUCCESS;
}
```
程序中使用inotify监听/dev/目录下的IN_CREATE和IN_DELETE事件,并检查设备名是否带有“usb”字符串。如果是,则根据/sys/class/目录下的相应设备目录中的uevent文件提取出设备的VID、PID、设备名等信息并打印出来。
阅读全文
相关推荐
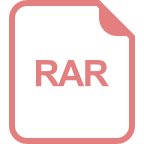




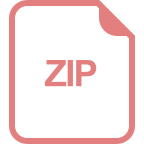
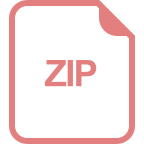
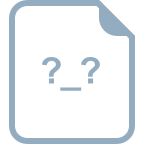
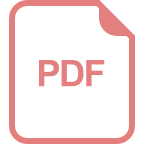
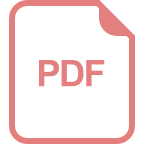
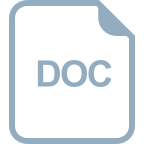
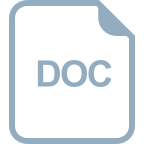
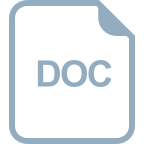
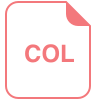


