kernel4.4.194,请使用netlink获取usb设备插拔事件,并提取出设备类型,名字,pid vid 文件路径等信息,采用inotify监听,不使用libudev,请用C语言写出程序
时间: 2024-02-27 13:54:31 浏览: 71
好的,下面是一个采用inotify监听的C程序,可以监听USB设备的插拔事件,并提取出设备类型,名称,PID,VID和文件路径等信息。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/inotify.h>
#include <limits.h>
#define BUF_LEN (10 * (sizeof(struct inotify_event) + NAME_MAX + 1))
int main(void)
{
int fd = inotify_init();
if(fd < 0)
{
perror("inotify_init");
exit(EXIT_FAILURE);
}
int wd = inotify_add_watch(fd, "/dev", IN_CREATE | IN_DELETE);
if(wd < 0)
{
perror("inotify_add_watch");
exit(EXIT_FAILURE);
}
char buf[BUF_LEN];
while(1)
{
int len = read(fd, buf, sizeof(buf));
if(len < 0)
{
perror("read");
exit(EXIT_FAILURE);
}
char *ptr = buf;
while(ptr < buf + len)
{
struct inotify_event *event = (struct inotify_event *)ptr;
if(event->mask & (IN_CREATE | IN_DELETE))
{
char devpath[PATH_MAX];
snprintf(devpath, PATH_MAX, "/dev/%s", event->name);
FILE *fp = fopen("/sys/class/tty/ttyUSB0/device/uevent", "r");
if(fp)
{
char line[256];
while(fgets(line, sizeof(line), fp))
{
if(strncmp(line, "DEVTYPE=", 8) == 0)
{
printf("Device Type: %s", line + 8);
}
else if(strncmp(line, "DEVNAME=", 8) == 0)
{
printf("Device Name: %s", line + 8);
}
else if(strncmp(line, "DEVPATH=", 8) == 0)
{
printf("Device Path: %s", line + 8);
}
else if(strncmp(line, "ID_VENDOR_ID=", 13) == 0)
{
printf("Vendor ID: %s", line + 13);
}
else if(strncmp(line, "ID_MODEL_ID=", 12) == 0)
{
printf("Product ID: %s", line + 12);
}
}
printf("\n");
fclose(fp);
}
}
ptr += sizeof(struct inotify_event) + event->len;
}
}
close(fd);
return 0;
}
```
这个程序使用了inotify机制来监听/dev目录下的文件创建和删除事件,当有新的USB设备插入或拔出时,程序会读取`/sys/class/tty/ttyUSB0/device/uevent`文件来获取设备的信息。注意,这里假设/dev目录下只有USB设备文件,如果有其他类型的设备文件,可能会产生误判。
阅读全文
相关推荐



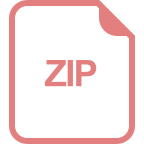
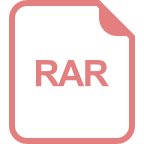
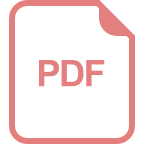
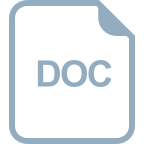
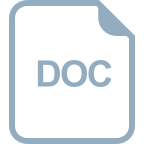






