dense(torch.rand(2, 5))
时间: 2023-09-25 13:12:47 浏览: 144
这是一个 PyTorch 的函数,它将一个大小为 (2, 5) 的随机数张量作为输入,并将其传递到一个全连接层(也称为密集层),然后输出一个大小为 (2, ) 的张量。具体实现可能类似于以下代码:
```python
import torch.nn as nn
import torch
fc = nn.Linear(5, 1)
input_tensor = torch.rand(2, 5)
output_tensor = fc(input_tensor).squeeze()
```
其中 `nn.Linear(5, 1)` 表示输入大小为 5,输出大小为 1 的全连接层,`squeeze()` 函数用于将输出张量的维度为 1 的维度去掉。
相关问题
class MyLinear(nn.Module): def init(self, in_units, units): super().init() self.weight = nn.Parameter(torch.randn(in_units, units)) self.bias = nn.Parameter(torch.randn(units,)) def forward(self, X): linear = torch.matmul(X, self.weight.data) + self.bias.data return F.relu(linear) dense = MyLinear(5,3) dense.weight dense(torch.rand(2, 5))
这段代码的作用是打印出自定义全连接层 `dense` 的权重张量。由于权重张量是一个 `nn.Parameter` 对象,因此需要调用 `.data` 方法来获取其值。具体实现类似于以下代码:
```python
import torch.nn as nn
import torch.nn.functional as F
import torch
class MyLinear(nn.Module):
def __init__(self, in_units, units):
super().__init__()
self.weight = nn.Parameter(torch.randn(in_units, units))
self.bias = nn.Parameter(torch.randn(units,))
def forward(self, X):
linear = torch.matmul(X, self.weight.data) + self.bias.data
return F.relu(linear)
dense = MyLinear(5, 3)
print(dense.weight.data)
```
输出的结果为一个大小为 (5, 3) 的随机数张量。
class MyLinear(nn.Module): def init(self, in_units, units): super().init() self.weight = nn.Parameter(torch.randn(in_units, units)) self.bias = nn.Parameter(torch.randn(units,)) def forward(self, X): linear = torch.matmul(X, self.weight.data) + self.bias.data return F.relu(linear) dense = MyLinear(5,3) dense.weightdense(torch.rand(2, 5))
这是一个使用 PyTorch 实现的自定义全连接层,相较于 `nn.Linear`,在 `forward` 函数中增加了 ReLU 激活函数的操作。具体实现类似于以下代码:
```python
import torch.nn as nn
import torch.nn.functional as F
import torch
class MyLinear(nn.Module):
def __init__(self, in_units, units):
super().__init__()
self.weight = nn.Parameter(torch.randn(in_units, units))
self.bias = nn.Parameter(torch.randn(units,))
def forward(self, X):
linear = torch.matmul(X, self.weight.data) + self.bias.data
return F.relu(linear)
dense = MyLinear(5, 3)
input_tensor = torch.rand(2, 5)
output_tensor = dense(input_tensor)
```
其中 `nn.Parameter` 用于将张量转换为模型参数,`forward` 函数中的 `F.relu` 表示 ReLU 激活函数。
阅读全文
相关推荐
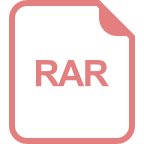
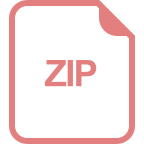

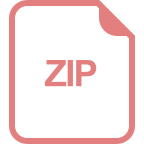
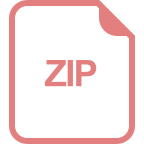
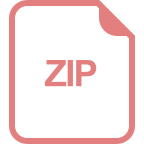
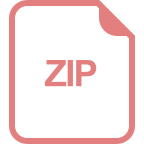
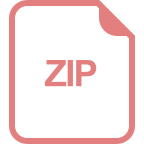
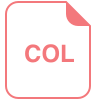
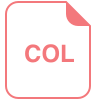
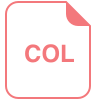
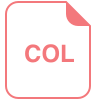
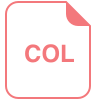
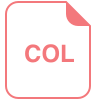
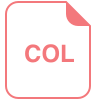
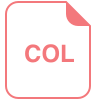