用python写一个免费的语音转文字的代码
时间: 2023-02-12 08:57:58 浏览: 167
您可以使用第三方库 SpeechRecognition 来实现语音转文字。首先需要安装该库,在命令行中输入:pip install SpeechRecognition。然后,您可以使用如下代码实现语音转文字:
```python
import speech_recognition as sr
def speech_to_text():
r = sr.Recognizer()
with sr.Microphone() as source:
audio = r.listen(source)
try:
text = r.recognize_google(audio, language='zh-CN')
print("你说的是: " + text)
except:
print("识别失败,请重试!")
speech_to_text()
```
请注意,这种方法使用的是 Google 的语音识别 API,如果您需要长时间使用,可能需要付费。
相关问题
使用python写一个语音实时转文字的代码
在Python中,可以使用` SpeechRecognition`库结合`pyaudio`或`gstreamer`来实现语音实时转文字的功能。以下是一个基本的示例,使用`SpeechRecognition`库配合Google Speech API:
```python
import speech_recognition as sr
# 创建一个Recognizer对象
r = sr.Recognizer()
# 如果你在本地运行,需要设置一个录音设备
mic_index = None # 更改为你系统的麦克风索引
with sr.Microphone(device_index=mic_index) as source:
print("启动语音识别...")
# 持续监听声音,直到有5秒的静默
r.pause_threshold = 0.5
audio_data = r.listen(source, timeout=5)
try:
# 将音频数据发送到Google Speech API进行转换
text = r.recognize_google(audio_data, language='zh-CN')
print(f"你说的是: {text}")
except sr.UnknownValueError:
print("无法识别语音")
except sr.RequestError as e:
print(f"请求错误; {e}")
# 更高级的使用还可以结合Google Cloud Speech-to-Text或其他API来处理
```
注意:
1. 这段代码需要网络连接才能访问Google的语音识别服务。
2. 需要先安装`SpeechRecognition`库 (`pip install SpeechRecognition`) 和可能的其他依赖。
3. Google Speech API可能会有一定的延迟,并不是实时的,这取决于网络速度。
用python写一个语音转文字的代码
这是一个使用 Python 的 SpeechRecognition 库将语音转换为文本的示例代码:
```python
import speech_recognition as sr
# 创建 Recognizer 对象
r = sr.Recognizer()
# 使用麦克风进行语音识别
with sr.Microphone() as source:
audio = r.listen(source)
# 识别语音
text = r.recognize_google(audio, language='zh-CN')
print(text)
```
需要注意的是,需要安装 speech_recognition 库和配置网络连接才能使用 Google 的语音识别API。
阅读全文
相关推荐
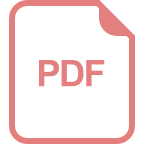
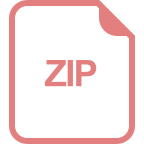













