可以提供一个比较简短简单的C语言OpenGL编写的双人贪吃蛇代码吗
时间: 2023-07-29 13:14:42 浏览: 48
好的,以下是一个简单的C语言OpenGL编写的双人贪吃蛇游戏代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <GL/glut.h>
#define WIDTH 400
#define HEIGHT 400
#define INIT_X 10
#define INIT_Y 10
#define INIT_LEN 3
int snake1_x[100], snake1_y[100], snake2_x[100], snake2_y[100];
int snake1_len = INIT_LEN, snake2_len = INIT_LEN;
int dir1 = 1, dir2 = 1;
int food_x, food_y;
void init() {
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(0, WIDTH, 0, HEIGHT);
snake1_x[0] = INIT_X;
snake1_y[0] = INIT_Y;
snake2_x[0] = WIDTH - INIT_X;
snake2_y[0] = HEIGHT - INIT_Y;
for (int i = 1; i < INIT_LEN; i++) {
snake1_x[i] = snake1_x[i-1] + 10;
snake1_y[i] = snake1_y[i-1];
snake2_x[i] = snake2_x[i-1] - 10;
snake2_y[i] = snake2_y[i-1];
}
food_x = rand() % (WIDTH/10) * 10;
food_y = rand() % (HEIGHT/10) * 10;
}
void drawFood() {
glColor3f(1.0, 0.0, 0.0);
glRectf(food_x, food_y, food_x+10, food_y+10);
}
void drawSnake(int x[], int y[], int len) {
glColor3f(0.0, 1.0, 0.0);
for (int i = 0; i < len; i++) {
glRectf(x[i], y[i], x[i]+10, y[i]+10);
}
}
void display() {
glClear(GL_COLOR_BUFFER_BIT);
drawFood();
drawSnake(snake1_x, snake1_y, snake1_len);
drawSnake(snake2_x, snake2_y, snake2_len);
glutSwapBuffers();
}
void moveSnake(int x[], int y[], int len, int dir) {
for (int i = len-1; i > 0; i--) {
x[i] = x[i-1];
y[i] = y[i-1];
}
switch(dir) {
case 1: // right
x[0] += 10;
break;
case 2: // up
y[0] += 10;
break;
case 3: // left
x[0] -= 10;
break;
case 4: // down
y[0] -= 10;
break;
}
}
void checkFood(int x[], int y[], int len) {
if (x[0] == food_x && y[0] == food_y) {
len++;
x[len-1] = x[len-2] + 10;
y[len-1] = y[len-2];
food_x = rand() % (WIDTH/10) * 10;
food_y = rand() % (HEIGHT/10) * 10;
}
}
void checkCollision(int x[], int y[], int len) {
if (x[0] < 0 || x[0] >= WIDTH || y[0] < 0 || y[0] >= HEIGHT) {
printf("Game Over!\n");
exit(0);
}
for (int i = 1; i < len; i++) {
if (x[0] == x[i] && y[0] == y[i]) {
printf("Game Over!\n");
exit(0);
}
}
}
void keyboard(unsigned char key, int x, int y) {
switch(key) {
case 'w':
if (dir2 != 4) dir2 = 2;
break;
case 'a':
if (dir2 != 1) dir2 = 3;
break;
case 's':
if (dir2 != 2) dir2 = 4;
break;
case 'd':
if (dir2 != 3) dir2 = 1;
break;
case 'i':
if (dir1 != 4) dir1 = 2;
break;
case 'j':
if (dir1 != 1) dir1 = 3;
break;
case 'k':
if (dir1 != 2) dir1 = 4;
break;
case 'l':
if (dir1 != 3) dir1 = 1;
break;
}
}
void timer(int value) {
moveSnake(snake1_x, snake1_y, snake1_len, dir1);
moveSnake(snake2_x, snake2_y, snake2_len, dir2);
checkFood(snake1_x, snake1_y, snake1_len);
checkFood(snake2_x, snake2_y, snake2_len);
checkCollision(snake1_x, snake1_y, snake1_len);
checkCollision(snake2_x, snake2_y, snake2_len);
glutPostRedisplay();
glutTimerFunc(100, timer, 0);
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB);
glutInitWindowSize(WIDTH, HEIGHT);
glutCreateWindow("Snake Game");
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glutTimerFunc(0, timer, 0);
init();
glutMainLoop();
return 0;
}
```
这是一个比较简单的双人贪吃蛇游戏实现,使用了OpenGL来绘制游戏界面,基本的操作和规则与一般的贪吃蛇游戏相同。注意这个代码只是提供一个示例,实际的游戏可能需要更多的功能和细节处理。
相关推荐
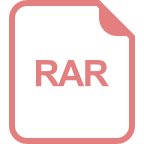
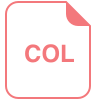
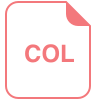
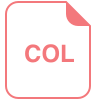
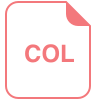
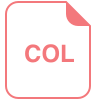









