c语言vector函数
时间: 2023-12-23 18:03:53 浏览: 85
在C语言中,没有内置的vector函数,但是可以通过自己实现来模拟vector的功能。下面是一个简单实现vector的例子:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int* data;
int size;
int capacity;
} vector;
void vector_init(vector* v) {
v->data = NULL;
v->size = 0;
v->capacity = 0;
}
void vector_push_back(vector* v, int element) {
if (v->size == v->capacity) {
int new_capacity = v->capacity == 0 ? 1 : v->capacity * 2;
int* new_data = (int*)realloc(v->data, new_capacity * sizeof(int));
if (new_data == NULL) {
printf("Error: out of memory\n");
exit(1);
}
v->data = new_data;
v->capacity = new_capacity;
}
v->data[v->size++] = element;
}
int vector_at(vector* v, int index) {
if (index < 0 || index >= v->size) {
printf("Error: index out of range\n");
exit(1);
}
return v->data[index];
}
void vector_clear(vector* v) {
free(v->data);
v->data = NULL;
v->size = 0;
v->capacity = 0;
}
int main() {
vector v;
vector_init(&v);
for (int i = 0; i < 10; i++) {
vector_push_back(&v, i);
}
for (int i = 0; i < v.size; i++) {
printf("%d ", vector_at(&v, i));
}
printf("\n");
vector_clear(&v);
return 0;
}
```
这个例子中,我们定义了一个结构体`vector`来保存vector的数据,包括指向数据的指针、当前数据的大小、当前数据的容量。然后我们实现了几个常用的vector函数,包括初始化、添加元素、访问元素、清空等。在`vector_push_back`函数中,我们使用`realloc`函数来动态分配内存,如果内存分配失败,则输出错误信息并退出程序。在`vector_at`函数中,我们检查给定的索引是否越界,如果越界,则输出错误信息并退出程序。在`vector_clear`函数中,我们使用`free`函数释放内存,并将指针和大小、容量都设置为0。
在主函数中,我们创建了一个vector,并添加了10个元素,然后遍历vector并输出所有元素。最后清空vector并返回0。
阅读全文
相关推荐





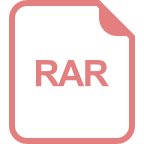








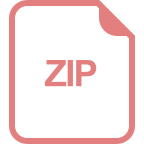

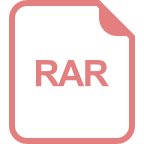
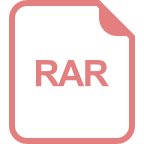
