<script src="problem_categories.json"> var data = JSON.parse(problem_categories); var select = document.getElementById("problem_category"); for (var i = 0; i < data.length; i++) { var option = document.createElement("option"); option.value = data[i].value; option.text = data[i].text; select.appendChild(option); } </script> 这段代码有什么错误?
时间: 2023-05-24 10:05:15 浏览: 129
该代码有两个错误:
1. `<script>`标签中应该指定`src`属性来链接外部的JSON文件,而不是将JSON数据直接放在`<script>`标签内部。
2. 循环中的`i`变量没有自增,会导致无限循环。
正确的代码应该是这样的:
```
<script src="problem_categories.json"></script>
<script>
fetch('problem_categories.json')
.then(response => response.json())
.then(data => {
var select = document.getElementById("problem_category");
for (var i = 0; i < data.length; i++) {
var option = document.createElement("option");
option.value = data[i].value;
option.text = data[i].text;
select.appendChild(option);
}
});
</script>
```
相关问题
HTML的option 的ID 为problem_category动态加载problem_categories.json数据
可以使用JavaScript来实现这个功能。
首先,在HTML中定义一个select元素,并为其添加一个id属性,如下所示:
```html
<select id="problem_category"></select>
```
然后,在JavaScript中使用XMLHttpRequest对象获取problem_categories.json文件中的数据,并将其解析为一个JavaScript对象。接着,遍历该对象的属性,将每个属性的值添加为一个option元素的value和text属性。最后,将这些option元素添加到select元素中。
完整的代码如下所示:
```html
<select id="problem_category"></select>
<script>
const selectElement = document.getElementById('problem_category');
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
const problemCategories = JSON.parse(xhr.responseText);
for (const category in problemCategories) {
const option = document.createElement('option');
option.value = category;
option.text = problemCategories[category];
selectElement.add(option);
}
}
};
xhr.open('GET', 'problem_categories.json');
xhr.send();
</script>
```
注意:为了在本地测试时避免跨域问题,应该将problem_categories.json文件放在与HTML文件同一目录下。如果部署到服务器上,应该将该文件放在一个可以访问的静态资源目录下。
<select class="form-control" id="problem_category" name="problem_category"> <option disabled selected>请选择问题类型</option> <script> // 创建XMLHttpRequest对象 var xhttp = new XMLHttpRequest(); // 设置请求方式和地址 xhttp.open("GET", "problem_categories.json", true); // 发送请求 xhttp.send(); var problemCategories = JSON.parse(this.responseText); for (const problemCategory of problemCategories.problem_categories) { document.write(`<option value="${problemCategory.id}">${problemCategory.name}</option>`); }; </script> </select> 在段代码的错误在哪里?
这段代码缺少一个事件监听器来处理请求完成后的响应。应该为xhttp对象添加一个onload事件监听器,将解析JSON和循环选项的代码放到该监听器中。修改后的代码如下:
```
<select class="form-control" id="problem_category" name="problem_category">
<option disabled selected>请选择问题类型</option>
<script>
// 创建XMLHttpRequest对象
var xhttp = new XMLHttpRequest();
// 设置请求方式和地址
xhttp.open("GET", "problem_categories.json", true);
// 添加事件监听器
xhttp.onload = function() {
var problemCategories = JSON.parse(this.responseText);
for (const problemCategory of problemCategories.problem_categories) {
document.write(`<option value="${problemCategory.id}">${problemCategory.name}</option>`);
};
};
// 发送请求
xhttp.send();
</script>
</select>
```
阅读全文
相关推荐
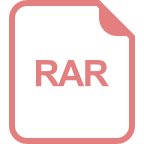
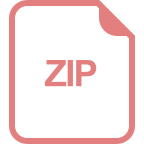














