import fitz # PyMuPDF库 import os # 读取PDF文件的内容 def read_pdf(file_path): doc = fitz.open(file_path) content = "" for page in doc: content += page.getText() doc.close() return content # 去除字符串中的空格和空行 def remove_spaces(text): return "\n".join([line.strip() for line in text.split("\n") if line.strip()]) # 将字符串按中文句号分割成多个语句 def split_sentences(text): sentences = [] for sentence in text.split("。"): sentence = sentence.strip() if sentence: sentences.append(sentence + "。") return sentences # 读取指定目录下所有PDF文件的内容并合并成一个字符串 def read_all_pdfs(dir_path): all_content = "" for file_name in os.listdir(dir_path): if file_name.endswith(".pdf"): file_path = os.path.join(dir_path, file_name) content = read_pdf(file_path) content = remove_spaces(content) all_content += content return all_content # 将字符串按中文句号分割成多个语句并打印出来 def print_sentences(text): sentences = split_sentences(text) for sentence in sentences: print(sentence) # 测试 dir_path = r"D:\点宽学院" all_content = read_all_pdfs(dir_path) print_sentences(all_content)这是我的代码,显示AttributeError: 'Page' object has no attribute 'getText',我该如何修改
时间: 2023-06-19 16:03:32 浏览: 272
这个错误可能是因为fitz库升级后Page对象没有getText属性了。你可以尝试使用PageText方法来获取文本内容。修改后的代码如下:
```python
import fitz # PyMuPDF库
import os # 读取PDF文件的内容
def read_pdf(file_path):
doc = fitz.open(file_path)
content = ""
for page in doc:
content += page.getText("text")
doc.close()
return content
# 去除字符串中的空格和空行
def remove_spaces(text):
return "\n".join([line.strip() for line in text.split("\n") if line.strip()])
# 将字符串按中文句号分割成多个语句
def split_sentences(text):
sentences = []
for sentence in text.split("。"):
sentence = sentence.strip()
if sentence:
sentences.append(sentence + "。")
return sentences
# 读取指定目录下所有PDF文件的内容并合并成一个字符串
def read_all_pdfs(dir_path):
all_content = ""
for file_name in os.listdir(dir_path):
if file_name.endswith(".pdf"):
file_path = os.path.join(dir_path, file_name)
content = read_pdf(file_path)
content = remove_spaces(content)
all_content += content
return all_content
# 将字符串按中文句号分割成多个语句并打印出来
def print_sentences(text):
sentences = split_sentences(text)
for sentence in sentences:
print(sentence)
# 测试
dir_path = r"D:\点宽学院"
all_content = read_all_pdfs(dir_path)
print_sentences(all_content)
```
你可以尝试运行一下看是否可以正常工作。
阅读全文
相关推荐
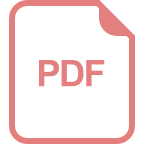
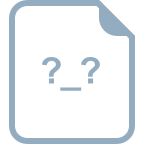














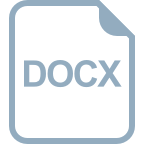