我想读取“D:\点宽学院”下所有PDF内容,将所有读取到的内容合并成一个字符串,去掉其中所有空行与空格。然后用中文句号进行分割,一句话中含有一个句号,把分割出来的语句一一打印出来,请用python实现,不能用pypdf2和pdfminer库
时间: 2023-06-19 20:03:41 浏览: 209
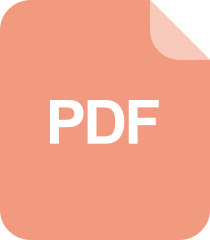
Python提取PDF内容的方法(文本、图像、线条等)

可以使用PyMuPDF库来实现这个功能。以下是代码实现:
```python
import fitz # PyMuPDF库
# 读取PDF文件的内容
def read_pdf(file_path):
doc = fitz.open(file_path)
content = ""
for page in doc:
content += page.getText()
doc.close()
return content
# 去除字符串中的空格和空行
def remove_spaces(text):
return "\n".join([line.strip() for line in text.split("\n") if line.strip()])
# 将字符串按中文句号分割成多个语句
def split_sentences(text):
sentences = []
for sentence in text.split("。"):
sentence = sentence.strip()
if sentence:
sentences.append(sentence + "。")
return sentences
# 读取指定目录下所有PDF文件的内容并合并成一个字符串
def read_all_pdfs(dir_path):
all_content = ""
for file_name in os.listdir(dir_path):
if file_name.endswith(".pdf"):
file_path = os.path.join(dir_path, file_name)
content = read_pdf(file_path)
content = remove_spaces(content)
all_content += content
return all_content
# 将字符串按中文句号分割成多个语句并打印出来
def print_sentences(text):
sentences = split_sentences(text)
for sentence in sentences:
print(sentence)
# 测试
dir_path = r"D:\点宽学院"
all_content = read_all_pdfs(dir_path)
print_sentences(all_content)
```
这段代码会读取指定目录下所有PDF文件的内容,并将它们合并成一个字符串。然后会去掉其中的空格和空行,并按中文句号分割成多个语句。最后将分割出来的语句逐一打印出来。
阅读全文
相关推荐
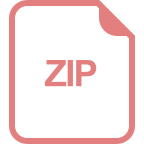


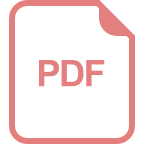
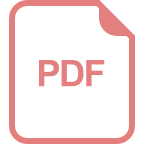
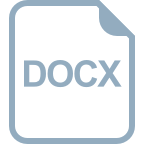
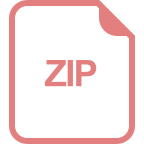
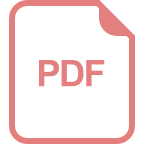
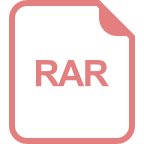
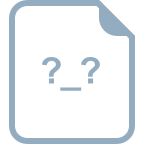
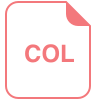
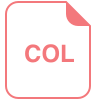
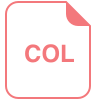
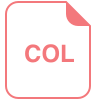
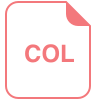
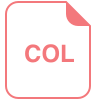
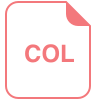