Python字符串处理与优化技巧:成为文本数据处理高手
发布时间: 2024-09-12 11:25:36 阅读量: 236 订阅数: 52 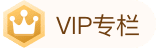
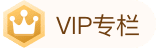
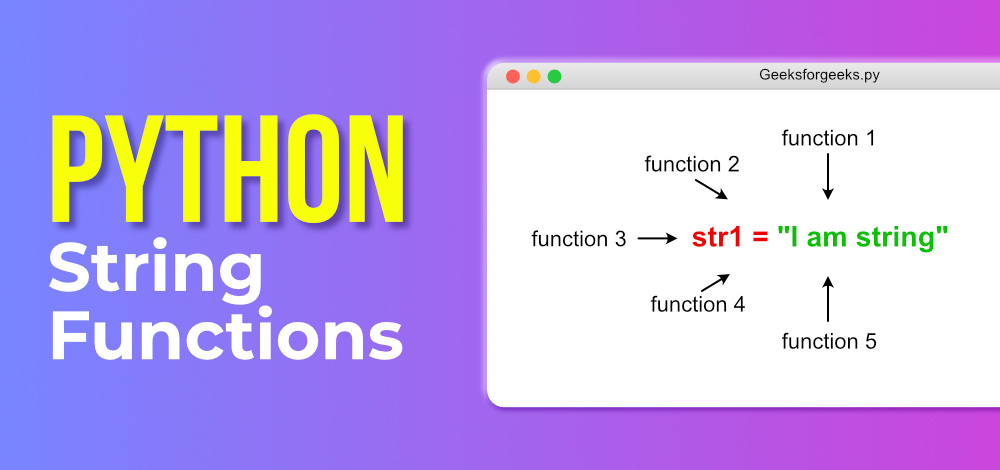
# 1. Python字符串处理基础
Python中的字符串是不可变的序列类型,它包含了对文本数据的各种处理方法。本章我们将从基础入手,了解如何在Python中创建和操作字符串。字符串字面量可以通过单引号('')、双引号("")或三引号('''或""")来定义,这为编写多行文本或包含特殊字符提供了极大的便利。
## 1.1 创建和表示字符串
创建字符串非常简单,只需将文本赋值给变量即可。
```python
greeting = "Hello, World!"
print(greeting)
```
## 1.2 字符串的基本操作
字符串支持多种基本操作,如连接、重复、索引和切片。
```python
# 字符串连接
concatenated = "Python" + "Programming"
# 字符串重复
repeated = "Code" * 3
# 字符串索引
first_char = greeting[0]
# 字符串切片
substring = greeting[0:5]
```
在后续章节中,我们将深入探讨字符串的高级操作与处理技术,本章为后续内容打下坚实基础。
# 2. 高级字符串处理技术
### 2.1 字符串的编码与解码
#### 2.1.1 Unicode和UTF-8的使用
Unicode提供了一个唯一的字符标识系统,它覆盖了世界上几乎所有的书面语言。Unicode不仅包括常见的字母和符号,还包括表情符号、历史文字等。在Python中处理文本时,理解Unicode及其变体UTF-8(一种经常用于文件和网络传输的编码格式)至关重要。
```python
# Python 3中的字符串默认为Unicode
text = 'hello, 世界'
# 将Unicode字符串编码为UTF-8
encoded_text = text.encode('utf-8')
# 将UTF-8编码的字节串解码回Unicode字符串
decoded_text = encoded_text.decode('utf-8')
print(f"Unicode字符串: {text}")
print(f"UTF-8编码的字节串: {encoded_text}")
print(f"解码回的Unicode字符串: {decoded_text}")
```
### 2.1.2 字符串的转换方法
在进行编码和解码时,我们可能会遇到不同的字符集,因此需要知道如何在不同的编码格式之间进行转换。Python提供了`encode`和`decode`方法用于此目的。
```python
# 将Unicode字符串转换为GBK编码
text_gbk_encoded = text.encode('gbk')
# 将GBK编码的字节串转换回Unicode字符串
text_gbk_decoded = text_gbk_encoded.decode('gbk')
print(f"GBK编码的字节串: {text_gbk_encoded}")
print(f"GBK解码回的Unicode字符串: {text_gbk_decoded}")
```
Unicode与UTF-8/GBK等编码方式之间的转换对于处理不同来源和目的的数据集尤为重要,尤其是在处理国际化文本和跨平台数据交换时。
### 2.2 格式化与模板化字符串
#### 2.2.1 传统的字符串格式化方法
在Python 3.6之前,字符串格式化通常使用`%`操作符或`str.format()`方法。使用`%`操作符进行格式化是一个传统的方式,但新式的f-string方法更简洁,因此通常更受欢迎。
```python
# 使用%操作符格式化字符串
formatted_text_percent = "Hello, %s!" % "world"
# 使用str.format()方法格式化字符串
formatted_text_format = "Hello, {}!".format("world")
print(formatted_text_percent)
print(formatted_text_format)
```
#### 2.2.2 新式的f-string格式化技术
从Python 3.6开始,f-string提供了一种更加直观和易读的方式来插入变量到字符串中。
```python
# 使用f-string进行字符串格式化
name = "Alice"
age = 30
f_string_formatted = f"My name is {name}, and I am {age} years old."
print(f_string_formatted)
```
#### 2.2.3 字符串模板的使用
除了f-string之外,`string.Template`类也可以用于字符串格式化,尤其是当需要从外部资源读取模板时。
```python
from string import Template
t = Template('Hello, $name!')
formatted_text_template = t.substitute(name='Bob')
print(formatted_text_template)
```
### 2.3 字符串操作的性能优化
#### 2.3.1 常见字符串操作的效率对比
字符串操作的效率取决于所用方法。Python支持多种字符串操作方法,了解它们的性能差异有助于编写高效的代码。
```python
import timeit
# 测试f-string格式化性能
f_string_performance = timeit.timeit('f"My name is {name}!"', globals=globals(), number=1000000)
# 测试%操作符格式化性能
percent_performance = timeit.timeit('"My name is %s!" % name', globals=globals(), number=1000000)
# 测试str.format()格式化性能
format_performance = timeit.timeit('"My name is {}!".format(name)', globals=globals(), number=1000000)
print(f"f-string formatting time: {f_string_performance}")
print(f"% formatting time: {percent_performance}")
print(f"str.format() formatting time: {format_performance}")
```
#### 2.3.2 使用内置函数提高处理速度
Python的内置字符串方法,比如`str.join()`, `str.split()`, `str.strip()`等,相比手动编写循环等方法通常更快速。
```python
# 测试使用str.join()合并字符串列表的性能
join_performance = timeit.timeit('" ".join(list)', globals=globals(), number=1000000)
# 测试使用for循环合并字符串列表的性能
loop_performance = timeit.timeit('"".join([x for x in list])', globals=globals(), number=1000000)
print(f"str.join() performance: {join_performance}")
print(f"Loop method performance: {loop_performance}")
```
#### 2.3.3 利用正则表达式优化复杂模式匹配
对于复杂的文本处理任务,如数据提取、文本分析等,正则表达式提供了一种强大的方式。正则表达式在Python中通过`re`模块实现,其性能经过优化,但使用不当可能造成性能下降。
```python
import re
# 测试正则表达式搜索性能
regex_performance = timeit.timeit('re.search(pattern, string)', setup='from __main__ import re, pattern, string', number=1000000)
print(f"Regular expression search performance: {regex_performance}")
```
### 2.4 字符串编码与解码的实战应用
在真实世界的应用中,编码与解码的需求无处不在。例如,处理来自网页或不同操作系统下的文本文件,通常需要使用Unicode字符串进行正确的编码和解码操作。
```python
# 示例:处理不同编码的文本数据
try:
# 尝试以GBK编码读取文本文件
with open('example_gbk.txt', 'r', encoding='gbk') as ***
***
***"GBK编码文件内容: {content}")
except UnicodeDecodeError:
print("读取GBK编码文件时发生错误")
try:
# 尝试以UTF-8编码读取文本文件
with open('example_utf8.txt', 'r', encoding='utf-8') as ***
***
***"UTF-8编码文件内容: {content}")
except UnicodeDecodeError:
print("读取UTF-8编码文件时发生错误")
```
在上述代码中,我们使用`try-except`块来处理可能出现的`UnicodeDecodeError`,这在处理从不同编码的文本数据时非常有用,特别是在处理用户上传的文件或网络爬取的数据时。
### 2.5 格式化和模板化在生产中的运用
在实际生产环境中,格式化和模板化字符串是创建报告、构建用户界面元素、生成数据输出等任务不可或缺的一部分。它们不仅帮助提升代码的可读性,还可以简化复杂文本处理的实现。
### 2.6 字符串处理的性能优化实践
在本章节的实践中,我们了解了如何利用Python内置方法和正则表达式来优化字符串处理性能。这些技术在处理大量文本数据时尤其重要,比如在构建搜索引擎、数据分析或日志处理系统时。
# 3. 字符串处理实战应用
## 3.1 文本清洗与预处理
在处理实际文本数据时,首先需要进行的步骤往往是文本的清洗与预处理。文本数据通常包含许多不必要的部分,如多余的空白字符、特殊字符、格式错误等,这些都会对后续的处理步骤造成影响。以下是一些常见的预处理步骤及其应用。
### 3.1.1 去除空白字符和特殊字符
在文本数据中,空白字符(包括空格、制表符、换行符等)和特殊字符可能会影响数据的准确性或分析的精确度。在Python中,我们可以使用`str.strip()`、`str.replace()`和`str.translate()`等方法来移除这些字符。
例如,下面的代码展示了如何移除字符串两端的空白字符:
```python
text = " Hello, world! "
clean_text = text.strip()
print(clean_text) # 输出: Hello, world!
```
要移除特定的特殊字符,可以使用`str.replace()`方法:
```python
text = "Hello, world!#"
clean_text = text.replace("#", "")
print(clean_text) # 输出: Hello, world!
```
使用`str.translate()`和`str.maketrans()`可以移除或替换多个字符:
```python
import string
translator = str.maketrans('', '', string.punctuation) # 移除所有标点符号
text = "Hello, world!"
clean_text = text.translate(translator)
print(clean_text) # 输出: Hello world
```
### 3.1.2 文本数据的标准化处理
标准化处理文本数据,主要是为了统一数
0
0
相关推荐
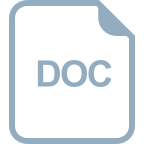
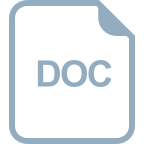
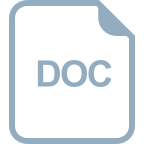
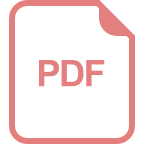
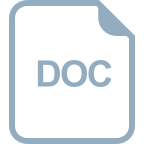
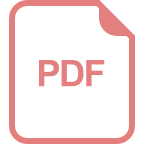
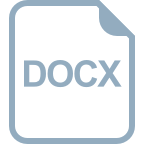
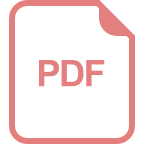
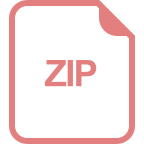