Python递归之美详解:深入理解递归算法的数据结构应用
发布时间: 2024-09-12 11:15:00 阅读量: 133 订阅数: 51 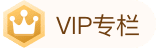
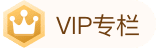
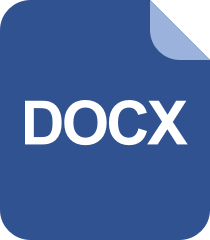
递归与Python Turtle分形树绘制详解(包含详细的完整的程序和数据)

# 1. Python递归概念与基础
## 1.1 递归的定义
递归是一种编程技术,它允许函数调用自身来解决问题。在Python中,递归是一种基本的编程范式,它适合于解决那些可以分解为相似子问题的问题。递归的基本思想是将问题分解为更小的相同问题,直到达到一个可以直接解决的简单情况,这个简单情况被称为基本情况。
## 1.2 递归函数的构建
递归函数由两部分组成:基本情况(Base Case)和递归步骤(Recursive Step)。基本情况用于终止递归,防止无限循环。递归步骤则将问题分解为更小的子问题,并调用自身来解决这些子问题。
```python
def recursive_function(parameters):
# 基本情况
if some_condition:
return base_value
# 递归步骤
else:
return recursive_function(modified_parameters)
```
在编写递归函数时,我们必须确保每次递归调用都使问题接近基本情况,否则将导致无限递归和栈溢出错误。
## 1.3 递归与Python的堆栈机制
Python使用调用堆栈来管理函数调用。每当我们调用一个函数时,一个新的栈帧就会被推送到堆栈上,包含函数的参数、局部变量和返回地址。递归函数利用堆栈的这一性质来保存每层递归的状态。理解堆栈的工作原理对于掌握递归至关重要,它有助于我们理解递归函数如何返回结果以及如何避免栈溢出问题。
在下一章,我们将深入了解递归的工作原理和理论基础,为解决更复杂的编程问题打下坚实的基础。
# 2. 递归算法的理论基础
## 2.1 递归的工作原理
### 2.1.1 递归函数的定义
递归是一种编程技术,它允许函数调用自身来解决问题。递归函数通过一系列重复的子问题来解决原问题,直到达到一个不再需要进一步递归的基本情况(base case)。
递归函数通常包含两个主要部分:
1. **基本情况(Base Case)**:这是递归的出口条件,它定义了当问题规模缩小到最小单元时应如何解决。如果缺乏基本案例,递归将无限进行下去,最终导致栈溢出错误。
2. **递归步骤(Recursive Step)**:这一步包含了函数调用自身的代码,通常伴随着问题规模的缩小。递归步骤是递归函数中实现问题解决逻辑的核心部分。
递归函数的伪代码结构如下:
```python
def recursive_function(parameters):
if base_condition(parameters):
return base_case_value
else:
result = recursive_function(modified_parameters)
# Post processing result if necessary
return result
```
让我们来看一个简单的递归函数例子——阶乘函数:
```python
def factorial(n):
if n == 0: # 基本情况
return 1
else:
return n * factorial(n - 1) # 递归步骤
```
阶乘函数`factorial`的递归逻辑是:`n`的阶乘等于`n`乘以`(n-1)`的阶乘,当`n`等于0时,返回1作为基本案例。
### 2.1.2 递归与迭代的对比
递归与迭代都是重复执行操作的方法,它们在某些情况下可以相互转换。然而,在理解递归时,它与迭代的主要区别在于:
- **递归**通过函数调用自身实现重复,涉及到函数调用栈。
- **迭代**通过循环语句(如`for`或`while`)重复执行代码块,仅需使用固定的内存空间。
在执行效率方面,迭代通常比递归更快,因为它避免了多次函数调用和相关的开销。然而,递归在实现某些算法时更为直观和简洁,特别是在涉及到自然递归结构的问题上。
递归的主要缺点是可能导致栈溢出错误,特别是在递归深度较大时。迭代由于只使用固定的内存空间,因此不太可能产生类似的错误。
## 2.2 递归算法的核心要素
### 2.2.1 基本情况与递归步骤
在任何递归函数中,都必须明确地定义基本情况和递归步骤。这两者构成了递归逻辑的核心,确保了递归能够正确并且安全地执行。
- **基本情况**是递归停止的条件,它定义了一个递归的“底部”,防止无限递归的发生。基本情况通常是对于最小输入集的直接解决方案,例如,对于阶乘函数,基本情况是当输入为0时返回1。
- **递归步骤**则描述了如何将问题分解为更小的问题,它涉及到修改输入参数以便函数可以处理更小规模的问题。在每次递归调用中,问题规模应该逐渐接近基本情况,以保证最终能够解决。
### 2.2.2 递归深度与效率分析
递归深度是指在一次递归调用中,函数自身被调用的最大次数。递归深度直接影响到程序的内存使用和性能。深度过大可能导致栈溢出错误,尤其是在有限的调用栈空间下。
- **递归深度的优化**:可以通过减少不必要的递归调用、使用尾递归(tail recursion)、或者在合适的情况下转用迭代方法来减少递归深度。
- **效率分析**:递归算法的效率通常取决于递归树的形状、每个递归调用的计算量以及基本情况的效率。复杂度高的递归算法可能导致指数级的时间复杂度,因此需要仔细设计递归结构以避免性能问题。
## 2.3 递归思想在数学中的应用
### 2.3.1 斐波那契数列的递归实现
斐波那契数列是一个经典的递归应用实例,其中每个数字是前两个数字的和。数学上定义如下:
```
F(0) = 0, F(1) = 1
F(n) = F(n-1) + F(n-2) for n > 1
```
递归实现斐波那契数列的代码如下:
```python
def fibonacci(n):
if n == 0: # 基本情况
return 0
elif n == 1: # 基本情况
return 1
else:
return fibonacci(n-1) + fibonacci(n-2) # 递归步骤
```
### 2.3.2 分形几何中的递归模式
分形几何是另一个递归思想广泛应用的领域。分形是一种自相似的几何结构,可以通过递归算法不断地细分来生成复杂的图案。
- **分形模式的递归生成**:使用递归算法可以创建如科赫雪花、曼德博集合、谢尔宾斯基地毯等复杂的分形图案。
- **递归与分形的关系**:递归算法在分形几何中的运用展示了如何通过简单的规则和递归步骤来构造出惊人的复杂结构。
分形几何通常要求递归函数能够以相同的模式在不同的尺度上重复自身,这在视觉上产生了无限重复的效果。因此,递归提供了一个自然的框架来描述和实现分形的生成过程。
# 3. 递归算法在数据结构中的应用
## 3.1 树结构的递归遍历
在计算机科学中,树结构是许多数据组织和算法的核心。树结构的递归遍历方法允许我们按照特定的顺序访问树中的每个节点。其中,二叉树是最常见的树结构,但递归遍历的概念可以扩展到N叉树。
### 3.1.1 二叉树的递归遍历方法
二叉树的递归遍历主要有三种方式:前序遍历(Pre-order)、中序遍历(In-order)和后序遍历(Post-order)。在每种遍历方法中,递归函数都会访问当前节点,然后递归地遍历左子树和右子树。
```python
# 定义二叉树节点类
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
# 前序遍历的递归实现
def preorder_traversal(root):
if root is None:
return
# 访问当前节点
print(root.value, end=' ')
# 递归遍历左子树
preorder_traversal(root.left)
# 递归遍历右子树
preorder_traversal(root.right)
# 中序遍历的递归实现
def inorder_traversal(root):
if root is None:
return
# 递归遍历左子树
inorder_traversal(root.left)
# 访问当前节点
print(root.valu
```
0
0
相关推荐
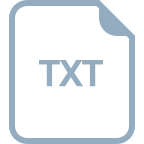
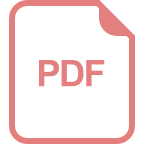
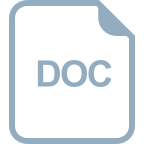
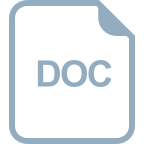
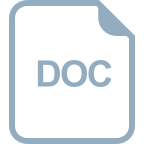
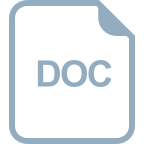
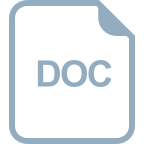
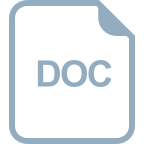