#include<stdio.h> int main() { int n; int cnt; int max; int i=9; int m; printf("Input n:"); scanf("%d",&a); int a[n]={0}; int b[n]={0}; m=n; printf("Input %d numbers:"); for(cnt=0;cnt<n;cnt++) { scanf("%d",&a[cnt]); } max=a[0]; while(i) { for(cnt=0;cnt<n;cnt++) { if(a[cnt]>max) { b[m-1]=max; a[cnt]=0; } } i--; m--; } printf("Sorting results:"); for(cnt=0;cnt<n;cnt++) { printf("%4d",b[cnt]); } return 0; }检查一下是否有错误
时间: 2024-01-13 18:03:39 浏览: 38
代码中存在一些错误和潜在问题:
1. 在输入 n 之前,变量 n 没有被初始化,这可能会导致不可预期的行为。
2. 在输入 n 之后,定义了大小为 n 的数组 a 和 b,但是 n 的值是未知的,可能会导致数组访问越界。
3. 在输出 "Input n:" 时,应该使用 printf 函数而不是直接输出。
4. 在输出 "Input %d numbers:" 时,应该使用 printf 函数并将 n 作为参数传递,否则会输出不正确的信息。
5. 在循环中,将 a[cnt] 设置为 0 可能会导致数据丢失或排序错误。
6. 在循环中,如果 a[cnt] 等于 max,则不应该将 b[m-1] 设置为 max,因为此时应该保持排序的稳定性。
下面是修改后的代码:
```
#include <stdio.h>
int main() {
int n, cnt, i, m, max_idx;
printf("Input n:");
scanf("%d", &n);
int a[n], b[n];
printf("Input %d numbers:", n);
for(cnt = 0; cnt < n; cnt++) {
scanf("%d", &a[cnt]);
}
for (i = 0; i < n; i++) {
max_idx = 0;
for (cnt = 1; cnt < n; cnt++) {
if (a[cnt] > a[max_idx]) {
max_idx = cnt;
}
}
b[i] = a[max_idx];
a[max_idx] = -1; // 标记为已经处理过
}
printf("Sorting results:");
for (cnt = 0; cnt < n; cnt++) {
printf("%4d", b[cnt]);
}
return 0;
}
```
这个程序使用选择排序算法对输入的数字进行排序,时间复杂度为 O(n^2),不太适合处理大规模数据。如果需要处理大量数据,应该选择更高效的排序算法,例如快速排序或归并排序。
相关推荐
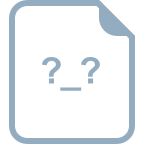
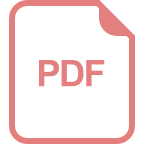















