springboot整合elasticsearch4.3.6
时间: 2023-09-17 10:14:59 浏览: 158
步骤如下:
1. 引入相关依赖
在 pom.xml 文件中引入 Elasticsearch 的相关依赖:
```xml
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.9.2</version>
</dependency>
<dependency>
<groupId>org.elasticsearch</groupId>
<artifactId>elasticsearch</artifactId>
<version>7.9.2</version>
</dependency>
```
2. 配置 Elasticsearch 连接信息
在 application.yml 文件中配置 Elasticsearch 的连接信息:
```yaml
elasticsearch:
host: localhost # Elasticsearch 的 IP 地址
port: 9200 # Elasticsearch 的端口号
```
3. 编写 Elasticsearch 配置类
在项目中创建 Elasticsearch 的配置类 ElasticsearchConfig,用于创建 Elasticsearch 的连接客户端 RestHighLevelClient。
```java
@Configuration
public class ElasticsearchConfig {
@Value("${elasticsearch.host}")
private String host;
@Value("${elasticsearch.port}")
private int port;
@Bean(destroyMethod = "close")
public RestHighLevelClient elasticsearchClient() {
RestClientBuilder builder = RestClient.builder(new HttpHost(host, port));
RestHighLevelClient client = new RestHighLevelClient(builder);
return client;
}
}
```
4. 编写 Elasticsearch 操作类
在项目中创建 Elasticsearch 的操作类 ElasticsearchService,用于实现 Elasticsearch 的相关操作,如创建索引、添加文档、更新文档、删除文档等。
```java
@Service
public class ElasticsearchService {
@Autowired
private RestHighLevelClient client;
/**
* 创建索引
*
* @param index 索引名称
* @throws IOException
*/
public void createIndex(String index) throws IOException {
CreateIndexRequest request = new CreateIndexRequest(index);
client.indices().create(request, RequestOptions.DEFAULT);
}
/**
* 添加文档
*
* @param index 索引名称
* @param id 文档id
* @param json 文档内容
* @throws IOException
*/
public void addDocument(String index, String id, String json) throws IOException {
IndexRequest request = new IndexRequest(index);
request.id(id);
request.source(json, XContentType.JSON);
client.index(request, RequestOptions.DEFAULT);
}
/**
* 更新文档
*
* @param index 索引名称
* @param id 文档id
* @param json 文档内容
* @throws IOException
*/
public void updateDocument(String index, String id, String json) throws IOException {
UpdateRequest request = new UpdateRequest(index, id);
request.doc(json, XContentType.JSON);
client.update(request, RequestOptions.DEFAULT);
}
/**
* 删除文档
*
* @param index 索引名称
* @param id 文档id
* @throws IOException
*/
public void deleteDocument(String index, String id) throws IOException {
DeleteRequest request = new DeleteRequest(index, id);
client.delete(request, RequestOptions.DEFAULT);
}
}
```
5. 测试 Elasticsearch 的相关操作
在测试类中编写 Elasticsearch 的相关操作,如创建索引、添加文档、更新文档、删除文档等。
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class ElasticsearchServiceTest {
@Autowired
private ElasticsearchService elasticsearchService;
@Test
public void testCreateIndex() throws IOException {
elasticsearchService.createIndex("test_index");
}
@Test
public void testAddDocument() throws IOException {
String json = "{\"name\":\"张三\",\"age\":18}";
elasticsearchService.addDocument("test_index", "1", json);
}
@Test
public void testUpdateDocument() throws IOException {
String json = "{\"name\":\"李四\",\"age\":20}";
elasticsearchService.updateDocument("test_index", "1", json);
}
@Test
public void testDeleteDocument() throws IOException {
elasticsearchService.deleteDocument("test_index", "1");
}
}
```
运行测试类,查看 Elasticsearch 的相关操作是否成功。
阅读全文
相关推荐
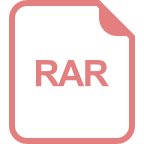
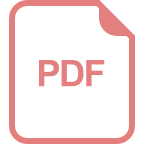
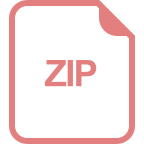
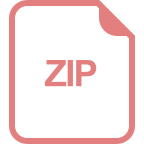
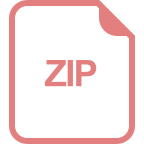





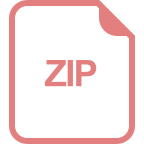
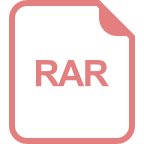
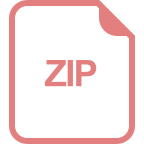
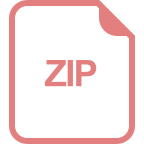