selenium爬虫如何获取网页的js
时间: 2024-05-19 09:15:39 浏览: 201
代码执行结果?
对于这个问题,你可以回答:
可以使用Selenium中的execute_script()方法来执行页面的JS代码,然后获取结果。例如,可以通过以下方法获取页面中id为test的元素的文本内容:
driver.execute_script("return document.getElementById('test').textContent;")
相关问题
selenium爬虫获取微博评论
Selenium是一个用于自动化测试的工具,可以模拟用户的操作来获取和处理网页数据。要使用Selenium实现微博评论的爬取,首先需要安装Selenium并配置好相关的浏览器驱动。
1. 首先,在Python中安装Selenium库,可以使用以下命令完成安装:
```
pip install selenium
```
2. 接下来,下载对应的浏览器驱动,并将其配置到系统环境变量中。常用的浏览器驱动有 ChromeDriver 和 GeckoDriver(Firefox浏览器的驱动)。选择合适的驱动版本下载并解压。
3. 导入Selenium库并创建浏览器实例。
```python
from selenium import webdriver
driver = webdriver.Chrome() # 使用Chrome浏览器驱动,或者使用Firefox驱动:webdriver.Firefox()
```
4. 打开微博页面,并搜索相关内容。可以使用WebDriver提供的`get()`方法打开指定的URL,并使用`find_element_by_id()`、`find_element_by_xpath()`等方法来定位元素进行搜索。
```python
driver.get("https://weibo.com")
# 在搜索框输入关键词并提交搜索
search_box = driver.find_element_by_xpath('//*[@id="plc_top"]/div/div[1]/div[1]/div/input')
search_box.send_keys("关键词")
search_btn = driver.find_element_by_xpath('//*[@id="plc_top"]/div/div[1]/div[1]/div/div/button')
search_btn.click()
```
5. 定位并点击评论按钮。根据微博页面的HTML结构,使用`find_element_by_xpath()`等方法定位到评论按钮,并点击。
```python
comment_btn = driver.find_element_by_xpath('//*[@class="icon_comment_b"]')
comment_btn.click()
```
6. 模拟滚动加载评论。由于微博评论通常是动态加载的,需要模拟滚动来加载更多评论。可以使用`execute_script()`方法执行JavaScript代码,将页面滚动到合适的位置。
```python
driver.execute_script("window.scrollTo(0, document.body.scrollHeight)") # 滚动到页面底部
```
7. 获取评论数据。根据微博页面的HTML结构,使用`find_element_by_xpath()`等方法定位到评论元素,然后通过`text`属性获取评论内容。
```python
comment_element = driver.find_element_by_xpath('//*[@class="comment_list"]')
comment_text = comment_element.text
```
8. 处理并保存评论数据。将获取到的评论数据进行处理和保存,可以将其存储到数据库或者写入到文件中。
9. 关闭浏览器实例。最后需要关闭浏览器实例,释放资源。
```python
driver.quit()
```
以上就是使用Selenium获取微博评论的基本流程,可以根据具体需求和页面结构进行相应的定位和处理操作。
selenium爬虫如何获取js中的url
可以使用Selenium的execute_script()方法来执行JavaScript代码,然后获取其中的URL。具体的代码可以参考以下示例:
```python
from selenium import webdriver
driver = webdriver.Chrome()
# 打开网页
driver.get('https://example.com')
# 执行JavaScript代码并获取其中的URL
url = driver.execute_script("return document.getElementById('myLink').getAttribute('href')")
print(url)
driver.quit()
```
在上面的代码中,我们使用了execute_script()方法来执行JavaScript代码,并使用getAttribute()方法获取链接的URL。
阅读全文
相关推荐
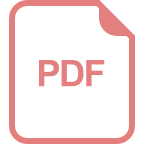
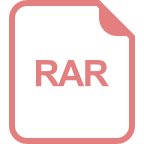
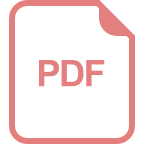













