牛顿插值法c++并绘图
时间: 2023-10-18 09:56:46 浏览: 62
好的,下面是牛顿插值法的C++代码实现和绘图,代码中使用了STL库中的vector和iostream,可以使用gnuplot进行绘图。
```cpp
#include <iostream>
#include <vector>
using namespace std;
// 计算阶乘
int factorial(int n){
int res = 1;
for(int i = 1; i <= n; i++){
res *= i;
}
return res;
}
// 计算差商
double diff_quot(vector<double>& x, vector<double>& y, int n, int i, int j){
if(j == 0){
return y[i];
}
else{
return (diff_quot(x, y, n, i + 1, j - 1) - diff_quot(x, y, n, i, j - 1)) / (x[i + j] - x[i]);
}
}
// 牛顿插值法
double newton_interpolation(vector<double>& x, vector<double>& y, double xi){
int n = x.size();
double res = 0.0;
for(int i = 0; i < n; i++){
double term = diff_quot(x, y, n, 0, i);
for(int j = 0; j < i; j++){
term *= (xi - x[j]);
}
res += term;
}
return res;
}
int main(){
vector<double> x = {1, 2, 4, 5}; // x坐标
vector<double> y = {-1, 0, 2, 1}; // y坐标
double xi = 3.5; // 待插值的位置
double yi = newton_interpolation(x, y, xi); // 插值结果
cout << "插值结果为:" << yi << endl;
// 绘制插值曲线
FILE* gp = popen("gnuplot -persist", "w");
fprintf(gp, "plot '-' with lines title 'Interpolation'\n");
for(double x_val = 0.5; x_val <= 5.5; x_val += 0.1){
fprintf(gp, "%lf %lf\n", x_val, newton_interpolation(x, y, x_val));
}
pclose(gp);
return 0;
}
```
这里绘制了插值曲线,插值曲线是一条光滑的曲线,它经过给定的数据点。可以使用gnuplot进行绘图,将插值曲线绘制出来。

相关推荐
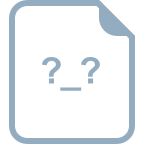














