ldr sp, [r3, r0, lsl #2]
时间: 2024-05-17 13:13:44 浏览: 116
这条指令是将一个带偏移的内存地址中的值加载到栈指针寄存器SP中。具体来说,它的操作数分别为r3、r0和#2,表示在r3寄存器的值基础上,加上r0寄存器的值左移2位(相当于乘以4),得到一个内存地址,然后从这个地址中加载一个32位的值到SP寄存器中。
这条指令通常用于初始化栈指针,以便在函数调用时正确地保存和恢复上下文。在ARM汇编中,栈通常是由高地址向低地址生长的,因此栈指针被初始化为最高地址的值。栈指针的值也可以通过其他方式进行初始化,例如使用MOV或ADD指令将一个常量加载到SP寄存器中。
需要注意的是,这条指令可能会引发内存访问异常,如果访问的内存地址无效或对应的内存页没有被映射。在实际编程中,需要确保访问的内存地址是合法的,并进行必要的异常处理。
希望这个回答能够帮到你,如果你还有其他问题,请继续提问。
相关问题
Use the following codes as the beginning of your program. Write a sort program to sort these ten numbers from largest to smallest, then save them to memory. For ARMSim#, the sorted number also need to be displayed in the console and LCD screen (use the Embest Board Plug‐In). MOV r0, #0x00002000 MOV r1, #18 STR r1, [r0],#4 MOV r1, #6 STR r1, [r0],#4 MOV r1, #2 STR r1, [r0],#4 MOV r1, #8 STR r1, [r0],#4 MOV r1, #16 STR r1, [r0],#4 MOV r1, #10 STR r1, [r0],#4 MOV r1, #14 STR r1, [r0],#4 MOV r1, #4 STR r1, [r0],#4 MOV r1, #20 STR r1, [r0],#4 MOV r1, #12 STR r1, [r0],#4
Sure, here is a possible solution in ARM assembly language:
```assembly
; Set up the constants and pointers
MOV r0, #0x00002000 ; Memory address to store the 10 numbers
MOV r1, #10 ; Number of elements to sort
LSL r2, r1, #2 ; Size of the array in bytes (10 * 4)
ADD r3, r0, r2 ; Pointer to the end of the array
; Copy the array to the stack for sorting
SUB sp, sp, r2 ; Allocate space on the stack
MOV r4, sp ; Pointer to the start of the stack
copy_loop:
LDR r5, [r0], #4 ; Load a number from the array
STR r5, [r4], #4 ; Store the number on the stack
CMP r0, r3 ; Check if we've reached the end of the array
BNE copy_loop ; If not, continue copying
; Sort the array using bubble sort
sort_loop:
MOV r4, sp ; Pointer to the start of the stack
inner_loop:
LDR r5, [r4], #4 ; Load the current number
LDR r6, [r4] ; Load the next number
CMP r5, r6 ; Compare the two numbers
BGE skip_swap ; If the current number is greater, skip the swap
STR r6, [r4, #-4]! ; Swap the numbers
STR r5, [r4], #4
MOV r7, #1 ; Set a flag to indicate that we swapped
skip_swap:
CMP r4, sp ; Check if we've reached the end of the array
BNE inner_loop ; If not, continue comparing
CMP r7, #1 ; Check if we swapped any numbers
BNE sort_done ; If not, the array is sorted and we're done
SUB r3, r3, #4 ; Otherwise, decrement the end pointer
B sort_loop ; and continue sorting
sort_done:
; Copy the sorted array back to memory
MOV r4, sp ; Pointer to the start of the stack
copy_back_loop:
LDR r5, [r4], #4 ; Load a number from the stack
STR r5, [r0], #4 ; Store the number in memory
CMP r0, r3 ; Check if we've reached the end of the array
BNE copy_back_loop ; If not, continue copying
; Display the sorted array in the console and LCD screen
MOV r0, #0x00002000 ; Memory address of the sorted array
MOV r1, #10 ; Number of elements to display
LSL r2, r1, #2 ; Size of the array in bytes (10 * 4)
MOV r3, #0x40000000 ; Base address of the Embest Board Plug-In
ADD r3, r3, #0x1000 ; Offset to the LCD screen buffer
ADD r4, r3, #0x80 ; Offset to the console output buffer
display_loop:
LDR r5, [r0], #4 ; Load a number from the sorted array
MOV r6, #0x30 ; ASCII code for '0'
ADD r6, r6, r5, LSL #1 ; Convert the number to a two-digit decimal string
STRB r6, [r4], #1 ; Display the string in the console
STRH r5, [r3], #2 ; Display the number in the LCD screen
CMP r0, r3 ; Check if we've reached the end of the array
BNE display_loop ; If not, continue displaying
; Clean up the stack and return from the program
ADD sp, sp, r2 ; Deallocate space on the stack
MOV pc, lr ; Return from the program
```
This program uses bubble sort to sort the 10 numbers in descending order, and then copies the sorted array back to memory. It also displays the sorted array in both the console and the LCD screen using the Embest Board Plug-In. Note that the LCD screen buffer is located at offset 0x1000 from the base address of the plug-in, and the console output buffer is located at offset 0x80. Also, the program assumes that the Embest Board Plug-In is connected and configured correctly.
上方要求使用汇编语言
如果你希望使用汇编语言来实现上述要求,你需要针对具体的微控制器架构编写代码。由于这里无法提供完整的汇编代码,我会给出一个通用的框架,以ARM Cortex- 在Cortex-M系列中,堆栈指针通常由处理器自动管理。但是为了明确起见,可以在`Reset_Handler`或`Vectors`表中设置初始堆栈指针。例如,在`Vectors.S`中:
```assembly
.thumb
.global Reset_Handler
Reset_Handler:
mov sp, #0x20006000
... // 跳转到其他初始化代码
```
2. **累加计算**:
- 创建一个`add_loop`函数,逐个累加数值,同时处理堆栈操作:
```assembly
add_loop:
ldr r1, [r0, #0x0] ; load next number
ldrb r2, [sp, #-1] ; pop from stack
adds r2, r1 ; add and update sum
cmp r1, #100 ; check loop condition
bne .add_loop ; branch if not done
str r2, [SUM1] ; store sum in SUM1
str r2, [SUM2] ; store sum in SUM2
ret
```
假设`r0`指向数组或寄存器,存储要累加的数字,而`sp`指向堆栈顶部。
3. **堆栈操作**:
- 对于堆栈操作,你可以使用`push`和`pop`指令,但在汇编中可能会更复杂,因为不是每个架构都支持直接访问堆栈:
```assembly
push_to_stack:
movs r3, #value ; 要压入的值
str r3, [stack, sp, lsl #2] ; 将值推入堆栈
subs sp, #1 ; 更新栈顶指针
bx lr ; 返回
```
4. **内存映射**:
- 分配好片内ROM和SRAM区域,并在代码中根据地址进行读写。这取决于你的芯片手册说明。
请注意,以上代码片段仅作示例,实际汇编代码会因处理器和工具链的不同而有所差异。使用汇编语言时,你还需要查阅相关的文档和头文件,如`syscalls.h`、`arch-microsemi.h`等。
阅读全文
相关推荐
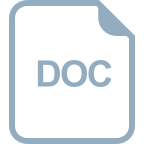
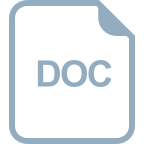
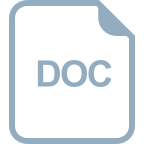
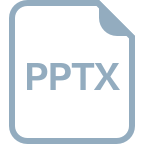
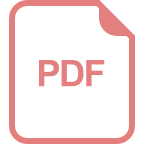
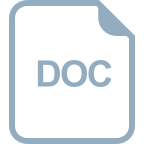
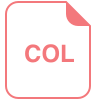



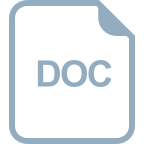
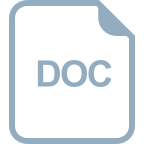
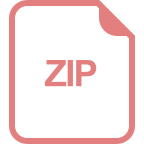
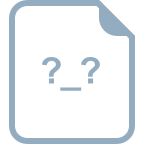
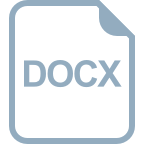
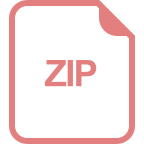
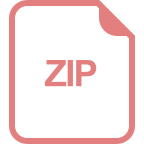