mybatis-plus自动填充时间
时间: 2023-05-31 17:20:22 浏览: 163
### 回答1:
Mybatis-plus 支持自动填充功能,其中包括填充时间。
你可以通过在实体类的字段上使用注解 @TableField(fill = FieldFill.INSERT) 来实现自动填充创建时间,使用注解 @TableField(fill = FieldFill.UPDATE) 来实现自动填充更新时间。
示例代码:
```
@TableField(fill = FieldFill.INSERT)
private LocalDateTime createTime;
@TableField(fill = FieldFill.UPDATE)
private LocalDateTime updateTime;
```
### 回答2:
Mybatis-Plus是基于Mybatis的增强工具包,该工具包提供了方便快捷的开发方式,其中一个重要功能就是自动填充时间。
自动填充时间功能可以在数据库表中创建日期和时间字段时,以特定方式填充或更新这些字段。在Mybatis-Plus中,自动填充时间可以通过以下几个步骤实现。
第一步,创建实体类
在实体类中,需要添加需要自动填充的字段和注解。如下所示:
```
@Data
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
private Integer age;
@TableField(fill = FieldFill.INSERT)
private Date createTime;
@TableField(fill = FieldFill.INSERT_UPDATE)
private Date updateTime;
}
```
其中,@TableField注解中fill属性用于指定自动填充的方式,FieldFill.INSERT表示新增时自动填充,FieldFill.INSERT_UPDATE表示新增和修改时都自动填充。
第二步,创建处理器
处理器用于在特定条件下填充时间。可以通过实现MetaObjectHandler接口来创建处理器。如下所示:
```
@Component
public class MyMetaObjectHandler implements MetaObjectHandler {
@Override
public void insertFill(MetaObject metaObject) {
this.strictInsertFill(metaObject, "createTime", Date.class, new Date());
this.strictInsertFill(metaObject, "updateTime", Date.class, new Date());
}
@Override
public void updateFill(MetaObject metaObject) {
this.strictUpdateFill(metaObject, "updateTime", Date.class, new Date());
}
}
```
其中,insertFill方法表示新增时填充,updateFill方法表示更新时填充。通过strictInsertFill和strictUpdateFill方法实现填充。
第三步,配置Mybatis-Plus
在Mybatis-Plus的配置文件中,需要添加自动填充的处理器。
```
@Configuration
public class MybatisPlusConfig {
@Autowired
private MyMetaObjectHandler myMetaObjectHandler;
@Bean
public MybatisPlusInterceptor mybatisPlusInterceptor() {
MybatisPlusInterceptor interceptor = new MybatisPlusInterceptor();
interceptor.addInnerInterceptor(new PaginationInnerInterceptor(DbType.MYSQL));
return interceptor;
}
@Bean
public GlobalConfig globalConfig() {
GlobalConfig config = new GlobalConfig();
config.setMetaObjectHandler(myMetaObjectHandler);
return config;
}
}
```
其中,globalConfig方法用于配置自动填充处理器。在配置中,setMetaObjectHandler用于指定处理器。
以上就是Mybatis-Plus自动填充时间的实现过程。通过注解指定需要填充的字段,实现处理器填充,最后在配置文件中配置自动填充处理器。自动填充时间功能可以方便地处理数据表中的时间字段,提高了开发效率。
### 回答3:
Mybatis-Plus是Mybatis的增强工具,提供了很多方便开发的功能,其中包括自动填充时间。
自动填充时间是指在插入或更新记录时,自动将时间字段填充上当前时间。这在开发中非常常见,比如我们需要在创建记录时记录下创建时间,或者在更新记录时记录下更新时间。
Mybatis-Plus提供了两种方式来实现自动填充时间:注解和接口实现。
1.注解方式
使用注解方式需要在实体类中添加字段注解使用@DateTimeFormat和@TableField,如下所示:
```
public class User {
@TableId(type = IdType.AUTO)
private Long id;
private String name;
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@TableField(fill = FieldFill.INSERT)
private Date createTime;
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss")
@TableField(fill = FieldFill.INSERT_UPDATE)
private Date updateTime;
}
```
在上述代码中,我们为createTime和updateTime字段添加了注解,@TableField中的fill属性指定了在插入和更新时自动填充时间。在使用自动填充时,需要在Mybatis-Plus的配置文件中开启自动填充功能,配置如下:
```
mybatis-plus:
global-config:
db-config:
logic-delete-value: 1
logic-not-delete-value: 0
id-type: auto
field-strategy: not_empty
insert-strategy: not_null
update-strategy: not_null
table-prefix: mp_
logic-delete-field: deleted
auto-fill: true
```
其中,auto-fill属性表示是否开启自动填充功能。开启后,在数据插入或更新时,自动将createTime和updateTime字段填充上当前时间。
2. 接口实现方式
除了使用注解方式,我们还可以使用接口实现方式,这种方式更加灵活。需要创建一个自动填充处理器实现MetaObjectHandler接口,如下所示:
```
@Component
public class MyMetaObjectHandler implements MetaObjectHandler {
@Override
public void insertFill(MetaObject metaObject) {
this.strictInsertFill(metaObject, "createTime", Date.class, new Date());
}
@Override
public void updateFill(MetaObject metaObject) {
this.strictUpdateFill(metaObject, "updateTime", Date.class, new Date());
}
}
```
在上述代码中,我们为createTime和updateTime字段添加了自动填充,通过调用strictInsertFill和strictUpdateFill方法实现。在Mybatis-Plus的配置文件中,需要注册这个自动填充处理器,配置如下:
```
mybatis-plus:
global-config:
db-config:
logic-delete-value: 1
logic-not-delete-value: 0
id-type: auto
field-strategy: not_empty
insert-strategy: not_null
update-strategy: not_null
table-prefix: mp_
logic-delete-field: deleted
meta-object-handler: com.example.mydemo.config.MyMetaObjectHandler
```
在开启了自动填充功能之后,无论是使用注解方式还是接口实现方式,都可以轻松实现自动填充时间。这可以大大减少开发中重复的时间填充操作,提高开发效率。
阅读全文
相关推荐
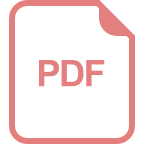
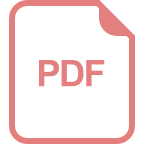
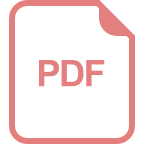

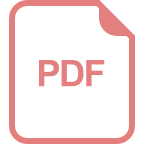



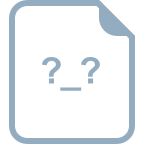
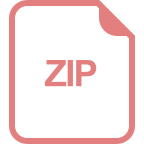
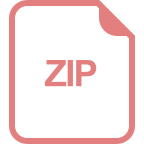
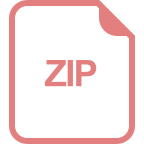
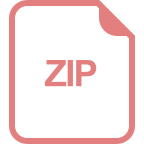
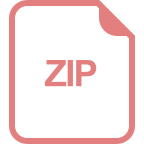
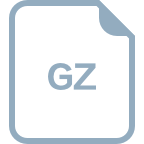



