spring data mongodb多表分组
时间: 2023-08-07 20:07:48 浏览: 56
在 Spring Data MongoDB 中,可以使用 Aggregation Framework 来进行多表分组。下面是一个示例:
假设我们有两个集合,一个是 orders,另一个是 customers,orders 集合中有一个字段 customer_id,表示订单所属的客户。现在我们想要按照客户性别统计他们的订单数量。可以使用以下代码实现:
```java
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.data.mongodb.core.aggregation.Aggregation;
import org.springframework.data.mongodb.core.aggregation.Fields;
import org.springframework.data.mongodb.core.aggregation.LookupOperation;
import org.springframework.data.mongodb.core.query.Criteria;
import org.springframework.stereotype.Repository;
import java.util.List;
@Repository
public class OrderRepositoryImpl implements OrderRepositoryCustom {
private final MongoTemplate mongoTemplate;
public OrderRepositoryImpl(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
@Override
public List<OrderCountByGender> countOrdersByGender() {
LookupOperation lookupOperation = LookupOperation.newLookup()
.from("customers")
.localField("customer_id")
.foreignField("_id")
.as("customer");
Aggregation aggregation = Aggregation.newAggregation(
lookupOperation,
Aggregation.project("customer.gender"),
Aggregation.group("customer.gender").count().as("count"),
Aggregation.project(Fields.fields("count")).and("gender").previousOperation()
);
return mongoTemplate.aggregate(aggregation, "orders", OrderCountByGender.class).getMappedResults();
}
}
```
其中 OrderCountByGender 是一个 POJO 类,用于存储按照性别统计的订单数量:
```java
public class OrderCountByGender {
private String gender;
private Long count;
// getters and setters
}
```
在上面的代码中,我们使用 LookupOperation 将 orders 集合中的 customer_id 与 customers 集合中的 _id 关联起来。然后,使用 Aggregation 进行分组统计,最后使用 mongoTemplate.aggregate 方法执行聚合操作,并将结果映射到 OrderCountByGender 类型的对象列表中返回。
相关推荐
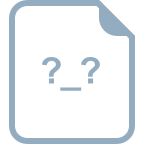
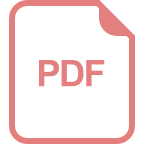














