unordered_set
时间: 2023-08-31 10:08:44 浏览: 49
unordered_set 是 C++ STL 中的一个容器,它是一个不重复的元素集合,内部实现采用哈希表,因此支持快速的插入、查找、删除操作。它的元素是无序的,插入元素的顺序不影响元素在集合中的位置。使用 unordered_set 前需要包含头文件 <unordered_set>。你可以使用 insert()、find()、erase()、size() 等函数来操作 unordered_set。
相关问题
set unordered_set
unordered_set is a container in C++ Standard Template Library (STL) that stores a collection of unique elements. It is an associative container, meaning the elements are stored using a hash table implementation to provide constant-time average complexity for insertion, deletion, and search operations.
To use unordered_set in C++, you need to include the <unordered_set> header file and declare a variable of type unordered_set. Here's an example:
```cpp
#include <unordered_set>
int main() {
std::unordered_set<int> set;
// Insert elements
set.insert(10);
set.insert(20);
set.insert(30);
// Check if an element exists
if (set.find(20) != set.end()) {
std::cout << "Element found!" << std::endl;
}
// Remove an element
set.erase(10);
return 0;
}
```
In this example, we create an unordered_set called `set` to store integers. We insert three elements (10, 20, and 30) using the `insert` function. We then check if the element 20 exists using the `find` function and erase the element 10 using the `erase` function.
unordered_set和set
unordered_set和set都是C++ STL库中的容器,用于存储唯一的元素。它们之间的主要区别在于元素的存储和访问方式。
1. set:set是一个基于红黑树实现的有序容器。它会自动对元素进行排序,并且保证元素的唯一性。因此,当需要有序存储元素且不允许重复时,可以选择使用set。
2. unordered_set:unordered_set是一个基于哈希表实现的无序容器。它使用哈希函数对元素进行散列,从而实现快速的插入、查找和删除操作。由于元素是无序存储的,因此在某些场景下,unordered_set的性能可能会比set更好。
总结来说,如果对元素的顺序要求不高,且需要快速的查找和插入操作,可以选择使用unordered_set。如果需要有序存储元素,并且不允许重复,则可以选择使用set。
阅读全文
相关推荐
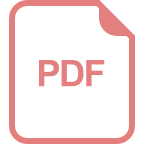
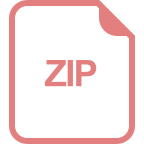









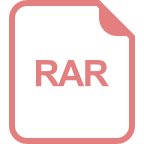
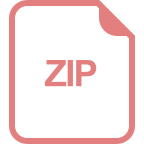
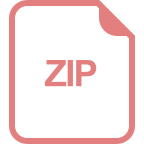