给出用resnet-18进行图像特征提取并在原图上用热力图的形式展示该过程中哪个特征重要的python代码
时间: 2024-03-21 14:41:13 浏览: 16
以下是使用ResNet-18进行图像特征提取并在原图上用热力图形式展示该过程中哪个特征重要的Python代码:
```python
import torch
import torch.nn as nn
from torchvision import models, transforms
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# Load pre-trained ResNet-18 model
model = models.resnet18(pretrained=True)
# Remove the fully connected layer from the model
model = nn.Sequential(*list(model.children())[:-1])
# Set model to evaluation mode
model.eval()
# Define image transformation to match the pre-processing used during training
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])
])
# Load sample image
img = Image.open('sample_image.jpg')
# Apply transformation and convert to tensor
img_tensor = transform(img).unsqueeze(0)
# Pass image tensor through ResNet-18 to get feature maps
with torch.no_grad():
features = model(img_tensor)
# Convert features to numpy array
features = features.squeeze().numpy()
# Calculate global average pooling of feature maps
pooled_features = np.mean(features, axis=(1,2))
# Reshape pooled features to match spatial dimensions of feature maps
pooled_features = np.repeat(pooled_features, features.shape[1]*features.shape[2]).reshape(features.shape)
# Calculate importance of each feature map by comparing it to the global average
heatmap = np.abs(features - pooled_features)
# Normalize heatmap to range between 0 and 1
heatmap = (heatmap - np.min(heatmap)) / (np.max(heatmap) - np.min(heatmap))
# Plot heatmap on top of original image
plt.imshow(img)
plt.imshow(heatmap.sum(axis=0), alpha=0.5, cmap='jet')
plt.axis('off')
plt.show()
```
该代码加载了预训练的ResNet-18模型,并使用其提取输入图像的特征。然后,代码计算每个特征图在整个图像中的重要性,并使用热力图的形式将其叠加在原始图像上展示。
相关推荐
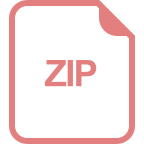
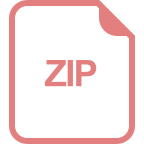
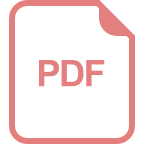














