用Resnet50模型对桥梁裂缝数据集中裂缝图像进行特征提取的具体实现代码python
时间: 2023-06-26 18:07:33 浏览: 117
以下是使用ResNet50模型对桥梁裂缝数据集进行特征提取的Python示例代码:
```python
# 导入必要的库
import tensorflow as tf
from tensorflow.keras.applications.resnet50 import ResNet50, preprocess_input
from tensorflow.keras.preprocessing.image import img_to_array, load_img
import numpy as np
import os
# 加载ResNet50模型
resnet_model = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3))
# 定义函数进行图像预处理和特征提取
def extract_features(image_path):
# 加载图像
img = load_img(image_path, target_size=(224, 224))
# 将图像转换为数组并进行预处理
img_array = img_to_array(img)
img_array = np.expand_dims(img_array, axis=0)
img_array = preprocess_input(img_array)
# 使用ResNet50模型提取特征
features = resnet_model.predict(img_array)
return features.flatten()
# 遍历数据集中的所有图像进行特征提取
data_dir = 'path/to/dataset'
features = []
labels = []
for label in os.listdir(data_dir):
label_dir = os.path.join(data_dir, label)
for image_file in os.listdir(label_dir):
image_path = os.path.join(label_dir, image_file)
# 提取特征并将其添加到特征列表中
feature = extract_features(image_path)
features.append(feature)
# 添加标签到标签列表中
labels.append(label)
# 将特征和标签转换为NumPy数组并保存到文件中
features = np.array(features)
labels = np.array(labels)
np.save('features.npy', features)
np.save('labels.npy', labels)
```
这段代码假设数据集中每个类别的图像都存储在一个单独的文件夹中,并且文件夹的名称就是类别的名称。代码会遍历整个数据集,对每个图像提取特征并将其添加到特征列表中,同时将图像的标签添加到标签列表中。最后,它将特征和标签转换为NumPy数组并保存到文件中。注意,这里使用了ResNet50模型进行特征提取,因此需要先安装TensorFlow和Keras库。
阅读全文
相关推荐
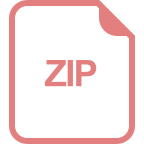
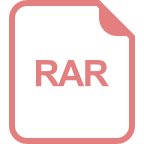
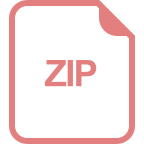















