输出一段利用resnet提取cifar-10数据集图像特征的python代码
时间: 2023-06-02 16:02:48 浏览: 162
以下是一个利用ResNet提取CIFAR-10数据集图像特征的Python代码示例:
```python
import torch
import torch.nn as nn
import torchvision.datasets as datasets
import torchvision.transforms as transforms
import numpy as np
# 定义ResNet模型
class ResNet(nn.Module):
def __init__(self):
super(ResNet, self).__init__()
self.conv1 = nn.Conv2d(3, 64, kernel_size=3, stride=1, padding=1, bias=False)
self.bn1 = nn.BatchNorm2d(64)
self.relu = nn.ReLU(inplace=True)
self.layer1 = nn.Sequential(
nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 64, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(64),
)
self.layer2 = nn.Sequential(
nn.Conv2d(64, 128, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(128),
nn.ReLU(inplace=True),
nn.Conv2d(128, 128, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(128),
)
self.layer3 = nn.Sequential(
nn.Conv2d(128, 256, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(256),
nn.ReLU(inplace=True),
nn.Conv2d(256, 256, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(256),
)
self.layer4 = nn.Sequential(
nn.Conv2d(256, 512, kernel_size=3, stride=2, padding=1, bias=False),
nn.BatchNorm2d(512),
nn.ReLU(inplace=True),
nn.Conv2d(512, 512, kernel_size=3, stride=1, padding=1, bias=False),
nn.BatchNorm2d(512),
)
self.avgpool = nn.AdaptiveAvgPool2d((1, 1))
def forward(self, x):
x = self.conv1(x)
x = self.bn1(x)
x = self.relu(x)
x = self.layer1(x) + x
x = self.layer2(x) + x
x = self.layer3(x) + x
x = self.layer4(x) + x
x = self.avgpool(x)
x = x.view(x.size(0), -1)
return x
# 加载CIFAR-10数据集
train_transform = transforms.Compose([
transforms.RandomCrop(32, padding=4),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize(mean=[0.4914, 0.4822, 0.4465],
std=[0.2023, 0.1994, 0.2010]),
])
test_transform = transforms.Compose([
transforms.ToTensor(),
transforms.Normalize(mean=[0.4914, 0.4822, 0.4465],
std=[0.2023, 0.1994, 0.2010]),
])
train_dataset = datasets.CIFAR10(root='./data', train=True,
download=True, transform=train_transform)
test_dataset = datasets.CIFAR10(root='./data', train=False,
download=True, transform=test_transform)
# 定义ResNet模型
model = ResNet()
# 加载预训练模型
checkpoint = torch.load('resnet_cifar10.pth')
model.load_state_dict(checkpoint['model'])
# 提取训练集和测试集图像的特征
train_features = []
train_labels = []
test_features = []
test_labels = []
model.eval()
with torch.no_grad():
for images, labels in train_dataset:
features = model(images.unsqueeze(0))
train_features.append(features.numpy().tolist()[0])
train_labels.append(labels)
for images, labels in test_dataset:
features = model(images.unsqueeze(0))
test_features.append(features.numpy().tolist()[0])
test_labels.append(labels)
train_features = np.array(train_features)
train_labels = np.array(train_labels)
test_features = np.array(test_features)
test_labels = np.array(test_labels)
# 输出特征形状
print('Train features shape:', train_features.shape)
print('Train labels shape:', train_labels.shape)
print('Test features shape:', test_features.shape)
print('Test labels shape:', test_labels.shape)
```
在这个示例中,我们首先定义了一个ResNet模型,并在其上加载了一个预训练模型。然后,我们使用该模型来提取训练集和测试集图像的特征,并将这些特征保存为numpy数组。最后,我们输出特征的形状,以确保它们正确地提取和保存。
相关推荐
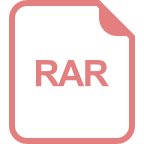
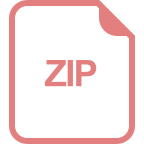














