stm32读取TLE5012速度代码
时间: 2023-09-25 16:15:35 浏览: 198
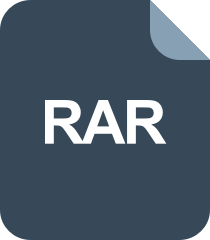
STM32编码器测速代码
以下是一个可能的STM32读取TLE5012速度的代码示例:
```c
#include "stm32f4xx.h"
#define TLE5012_SCK_PIN GPIO_Pin_5
#define TLE5012_SCK_PORT GPIOA
#define TLE5012_MISO_PIN GPIO_Pin_6
#define TLE5012_MISO_PORT GPIOA
#define TLE5012_CS_PIN GPIO_Pin_7
#define TLE5012_CS_PORT GPIOA
// TLE5012 command codes
#define TLE5012_READ_ANGLE 0x3FFF
#define TLE5012_READ_SPEED 0x3FFE
// TLE5012 data buffer
uint16_t TLE5012_data[2];
void TLE5012_init()
{
GPIO_InitTypeDef GPIO_InitStruct;
SPI_InitTypeDef SPI_InitStruct;
// Enable GPIOA and SPI1 clocks
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SPI1, ENABLE);
// Configure SCK and MISO pins as alternate function
GPIO_InitStruct.GPIO_Pin = TLE5012_SCK_PIN | TLE5012_MISO_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(TLE5012_SCK_PORT, &GPIO_InitStruct);
// Configure CS pin as output
GPIO_InitStruct.GPIO_Pin = TLE5012_CS_PIN;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(TLE5012_CS_PORT, &GPIO_InitStruct);
// Configure SPI1
SPI_InitStruct.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStruct.SPI_Mode = SPI_Mode_Master;
SPI_InitStruct.SPI_DataSize = SPI_DataSize_16b;
SPI_InitStruct.SPI_CPOL = SPI_CPOL_High;
SPI_InitStruct.SPI_CPHA = SPI_CPHA_2Edge;
SPI_InitStruct.SPI_NSS = SPI_NSS_Soft;
SPI_InitStruct.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_8;
SPI_InitStruct.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStruct.SPI_CRCPolynomial = 7;
SPI_Init(SPI1, &SPI_InitStruct);
// Enable SPI1
SPI_Cmd(SPI1, ENABLE);
}
void TLE5012_read_data()
{
// Select TLE5012 chip
GPIO_ResetBits(TLE5012_CS_PORT, TLE5012_CS_PIN);
// Send read angle command
SPI_I2S_SendData(SPI1, TLE5012_READ_ANGLE);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
TLE5012_data[0] = SPI_I2S_ReceiveData(SPI1);
// Send read speed command
SPI_I2S_SendData(SPI1, TLE5012_READ_SPEED);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_TXE) == RESET);
while (SPI_I2S_GetFlagStatus(SPI1, SPI_I2S_FLAG_RXNE) == RESET);
TLE5012_data[1] = SPI_I2S_ReceiveData(SPI1);
// Deselect TLE5012 chip
GPIO_SetBits(TLE5012_CS_PORT, TLE5012_CS_PIN);
}
int main(void)
{
// Initialize TLE5012
TLE5012_init();
while (1)
{
// Read TLE5012 data
TLE5012_read_data();
// Extract speed value
int16_t speed = (int16_t)(TLE5012_data[1] & 0x3FFF);
if ((TLE5012_data[1] & 0x4000) != 0) {
speed = -speed;
}
// Do something with speed value
// ...
// Delay for some time
for (int i = 0; i < 1000000; i++);
}
}
```
此代码示例包括以下步骤:
1. 定义TLE5012的引脚和命令代码。
2. 定义一个TLE5012数据缓冲区。
3. 初始化TLE5012,包括配置GPIO和SPI1。
4. 读取TLE5012数据,包括发送读取角度和速度的命令,等待SPI传输完成并接收数据,然后将数据存储到TLE5012数据缓冲区中。
5. 提取速度值,根据数据格式进行解码。
6. 对速度值进行处理,例如打印到串行端口或控制另一个设备。
7. 等待一段时间,然后重复步骤4到6。
这只是一个示例代码,具体实现可能因特定硬件和应用程序而有所不同。
阅读全文
相关推荐
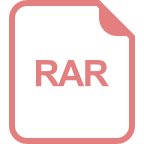
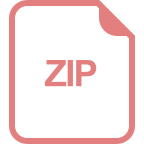
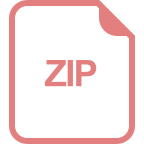
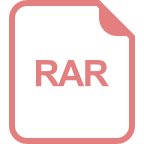
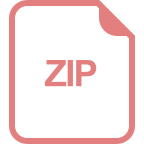
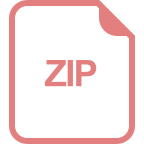
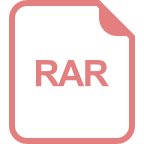
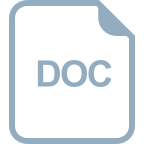
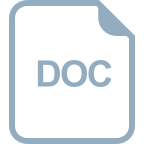
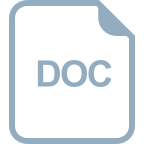
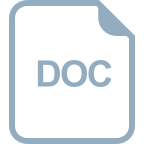
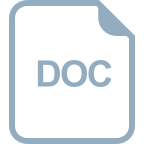
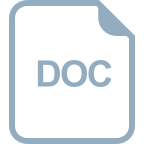


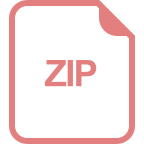