python如何获取excel数据所在的行和列_python 利用openpyxl读取Excel表格中指定的行或列教程...
时间: 2024-01-21 22:20:09 浏览: 87
使用openpyxl模块可以方便地读取和操作Excel文件。下面是获取Excel数据所在行和列的示例代码:
```python
import openpyxl
# 打开Excel文件
workbook = openpyxl.load_workbook('example.xlsx')
# 选择工作表
sheet = workbook['Sheet1']
# 获取第2行的数据
row_data = []
for cell in sheet[2]:
row_data.append(cell.value)
print(row_data)
# 获取第2列的数据
col_data = []
for cell in sheet['B']:
col_data.append(cell.value)
print(col_data)
# 获取单元格(2,3)的数据
cell_data = sheet.cell(row=2, column=3).value
print(cell_data)
```
以上代码中,我们首先打开Excel文件,然后选择要操作的工作表。接着,通过循环遍历行或列的所有单元格,获取对应的数据。最后,我们还可以通过指定单元格的行和列,获取该单元格的数据。
相关问题
修改代码,获取“绩效监测”表:ori_excel = openpyxl.load_workbook(ori_excel_path,data_only=True)#读取数据源表格 ori_sheet = ori_excel.active#获取第一个sheet
修改后的代码如下:
```python
ori_excel = openpyxl.load_workbook(ori_excel_path, data_only=True) # 读取数据源表格
ori_sheet = ori_excel["绩效监测"] # 获取“绩效监测”表
```
这样就可以直接获取到“绩效监测”表了。
规范代码:读取数据源表格 ori_excel = openpyxl.load_workbook(ori_excel_path) ori_sheet = ori_excel.active rows = ori_sheet.max_row cols = ori_sheet.max_column 加载公式解析器 from transformers import AutoTokenizer tokenizer = AutoTokenizer.from_pretrained("microsoft/CodeGPT-small-py") 遍历每一行 for r in range(2, rows + 1): info = {} # 定义一个字典用来存放数据 mould_excel = openpyxl.load_workbook(mould_excel_path) mould_sheet = mould_excel.active # 遍历每一列 for c in range(1, cols + 1): # 如果标题栏和单元格内有内容 ori_cell_value = ori_sheet.cell(1, c).value cell_value = ori_sheet.cell(r, c).value if ori_cell_value and cell_value: # 获取单元格数据类型和值 data_type = ori_sheet.cell(r, c).data_type value = cell_value # 如果单元格内是函数公式,则运算后写入 if data_type == 'f': # 公式解析 tokens = [str(token.value) if token.type == Token.NUMBER else repr(token.value) for token in tokenizer.parse(value)] # 运算 result = calculate(tokens) # 写入结果 mould_sheet.cell(row=r, column=c).value = result else: # 直接写入值 mould_sheet.cell(row=r, column=c).value = value # 将计算结果写入到模板表格 if data_type == 'f': mould_sheet.cell(row=r, column=c, value=result) else: mould_sheet.cell(row=r, column=c, value=value) else: # 如果单元格内不是函数公式,则直接写入 info[ori_cell_value] = cell_value mould_sheet.cell(row=r, column=c, value=cell_value)
下面是规范化后的代码:
```python
import openpyxl
from transformers import AutoTokenizer
# 读取数据源表格
ori_excel = openpyxl.load_workbook(ori_excel_path)
ori_sheet = ori_excel.active
rows = ori_sheet.max_row
cols = ori_sheet.max_column
# 加载公式解析器
tokenizer = AutoTokenizer.from_pretrained("microsoft/CodeGPT-small-py")
# 遍历每一行
for r in range(2, rows + 1):
info = {}
# 定义一个字典用来存放数据
mould_excel = openpyxl.load_workbook(mould_excel_path)
mould_sheet = mould_excel.active
# 遍历每一列
for c in range(1, cols + 1):
# 如果标题栏和单元格内有内容
ori_cell_value = ori_sheet.cell(1, c).value
cell_value = ori_sheet.cell(r, c).value
if ori_cell_value and cell_value:
# 获取单元格数据类型和值
data_type = ori_sheet.cell(r, c).data_type
value = cell_value
# 如果单元格内是函数公式,则运算后写入
if data_type == 'f':
# 公式解析
tokens = [str(token.value) if token.type == Token.NUMBER else repr(token.value) for token in tokenizer.parse(value)]
# 运算
result = calculate(tokens)
# 写入结果
mould_sheet.cell(row=r, column=c).value = result
else:
# 直接写入值
mould_sheet.cell(row=r, column=c).value = value
# 将计算结果写入到模板表格
if data_type == 'f':
mould_sheet.cell(row=r, column=c, value=result)
else:
mould_sheet.cell(row=r, column=c, value=value)
else:
# 如果单元格内不是函数公式,则直接写入
info[ori_cell_value] = cell_value
mould_sheet.cell(row=r, column=c, value=cell_value)
```
阅读全文
相关推荐
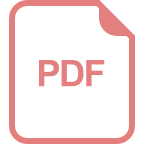
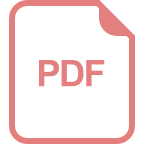
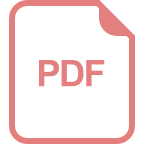
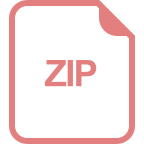
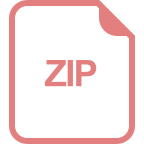
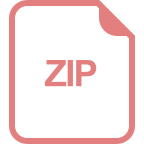
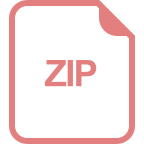
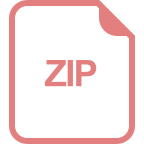
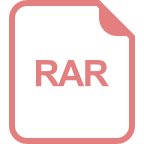
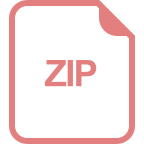
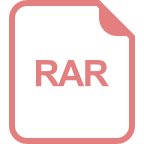
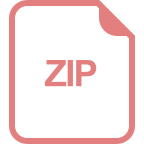
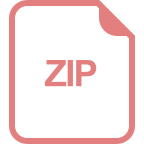
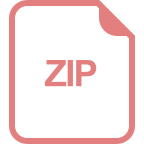

