用Java编写将任意两个文件合并到一个文件,要求采用java命令行方式在控制台按照“源文件1 源文件2 目标文件” 方式录入,注意多种异常处理。
时间: 2024-03-07 11:50:47 浏览: 89
好的,下面是一个简单的 Java 程序,用于将任意两个文件合并到一个文件:
```java
import java.io.*;
public class FileMerge {
public static void main(String[] args) {
if (args.length != 3) {
System.out.println("Usage: java FileMerge source1 source2 target");
return;
}
String sourceFile1 = args[0];
String sourceFile2 = args[1];
String targetFile = args[2];
try (BufferedInputStream in1 = new BufferedInputStream(new FileInputStream(sourceFile1));
BufferedInputStream in2 = new BufferedInputStream(new FileInputStream(sourceFile2));
BufferedOutputStream out = new BufferedOutputStream(new FileOutputStream(targetFile))) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = in1.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
while ((bytesRead = in2.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
System.out.println("File merge completed.");
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
} catch (IOException e) {
System.err.println("IOException: " + e.getMessage());
}
}
}
```
在上面的代码中,我们使用命令行参数 `args` 获取源文件 1、源文件 2 和目标文件的路径。然后,我们使用 `BufferedInputStream` 和 `BufferedOutputStream` 分别读取和写入文件内容。最后,我们捕获可能抛出的 `FileNotFoundException` 和 `IOException` 异常,并在控制台上显示错误消息。
你可以使用以下命令在控制台上运行这个程序:
```
java FileMerge source1.txt source2.txt target.txt
```
假设 `source1.txt` 和 `source2.txt` 是要合并的两个源文件,`target.txt` 是合并后的目标文件。如果一切正常,程序将在控制台上输出 `File merge completed.`。如果出现错误,程序将在控制台上显示相应的错误消息。
希望这可以帮助你编写一个 Java 程序,用于将任意两个文件合并到一个文件。
阅读全文
相关推荐




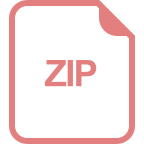







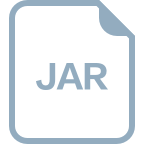
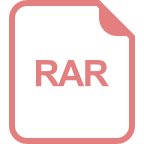

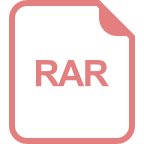