将任意两个文件合并到一个文件,要求采用java命令行方式在控制台按照“源文件1 源文件2 目标文件” 方式录入,注意多种异常处理。
时间: 2023-05-02 09:04:26 浏览: 183
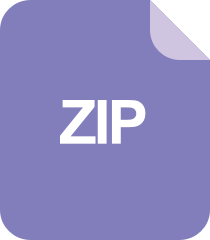
学生选修课程程序.zip
题目的意思是,使用Java命令行方式将任意两个文件合并成一个文件,要求在控制台按照“源文件1 源文件2 目标文件”的格式输入,注意多种异常处理。
回答:题目要求我们用Java命令行方式来合并两个文件到一个文件,可以使用以下代码实现:
```java
import java.io.*;
public class MergeFile {
public static void main(String[] args) {
if (args.length != 3) {
System.out.println("Usage: java MergeFile sourcefile1 sourcefile2 targetfile");
System.exit(1);
}
File sourceFile1 = new File(args[0]);
if (!sourceFile1.exists()) {
System.out.println("Source file " + args[0] + " does not exist");
System.exit(2);
}
File sourceFile2 = new File(args[1]);
if (!sourceFile2.exists()) {
System.out.println("Source file " + args[1] + " does not exist");
System.exit(3);
}
File targetFile = new File(args[2]);
if (targetFile.exists()) {
System.out.println("Target file " + args[2] + " already exists");
System.exit(4);
}
try (
BufferedInputStream input1 = new BufferedInputStream(
new FileInputStream(sourceFile1));
BufferedInputStream input2 = new BufferedInputStream(
new FileInputStream(sourceFile2));
BufferedOutputStream output = new BufferedOutputStream(
new FileOutputStream(targetFile));
) {
byte[] buffer = new byte[8192];
int bytesRead;
while ((bytesRead = input1.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
while ((bytesRead = input2.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
```
代码中先判断命令行参数长度是否为3,如果不为3则提示使用方式并退出程序。然后分别判断源文件1、源文件2和目标文件是否存在,如果源文件不存在则提示错误并退出程序,如果目标文件已经存在则提示错误并退出程序。接着将两个源文件的内容读入缓冲区,再将缓冲区中的内容写入目标文件。如果出现异常则打印异常信息。
阅读全文
相关推荐
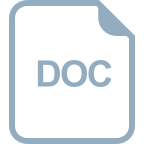
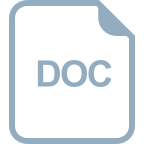


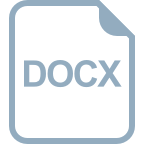
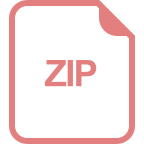
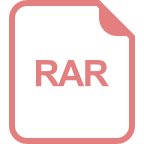

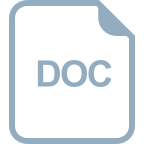
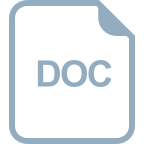
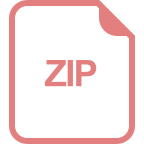
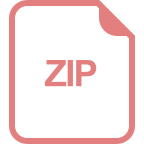
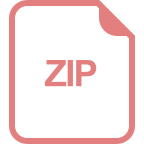
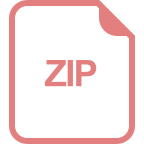
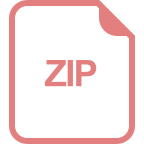
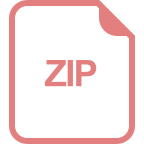
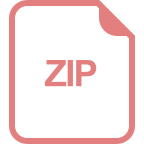
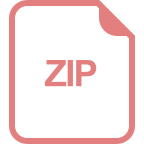