基于决策树的AdaBoost集成学习算法生成Vertebrate1数据集(见附件)的分类模型
时间: 2024-05-03 12:17:30 浏览: 20
AdaBoost算法是一种集成学习算法,它将多个弱分类器组合成一个强分类器,通常用于二分类问题。在这里,我们将使用AdaBoost算法来生成Vertebrate1数据集的分类模型。
首先,我们需要导入数据集并对其进行预处理。Vertebrate1数据集包含5个属性和1个类别标签(类别标签为1或2),属性包括有鳞片、产卵、毒性、肺呼吸和水生。我们可以使用pandas库读取数据集并进行预处理,将类别标签转换为1和-1。
```python
import pandas as pd
# 读取数据集
data = pd.read_csv('Vertebrate1.csv', header=None)
# 将类别标签转换为1和-1
data.iloc[:, -1] = data.iloc[:, -1].apply(lambda x: 1 if x == 1 else -1)
# 分割数据集为训练集和测试集
train_data = data.iloc[:80, :]
test_data = data.iloc[80:, :]
```
接下来,我们将使用决策树作为弱分类器,并使用AdaBoost算法进行集成学习。在每一轮迭代中,我们将根据当前的数据分布构建一个决策树,并计算分类误差和权重系数。然后,我们将更新数据分布,使得被错误分类的样本的权重更高,被正确分类的样本的权重更低。最终,我们将多个弱分类器的预测结果进行加权求和,得到最终的预测结果。
```python
from sklearn.tree import DecisionTreeClassifier
class AdaBoost:
def __init__(self, n_estimators):
self.n_estimators = n_estimators
self.estimators = []
self.alphas = []
def fit(self, X, y):
# 初始化数据分布权重
w = [1/len(X)] * len(X)
for i in range(self.n_estimators):
# 根据当前的数据分布构建决策树
estimator = DecisionTreeClassifier(max_depth=1)
estimator.fit(X, y, sample_weight=w)
# 计算分类误差和权重系数
y_pred = estimator.predict(X)
error = sum(w[i] for i in range(len(X)) if y_pred[i] != y[i])
alpha = 0.5 * (np.log(1 - error) - np.log(error))
# 更新数据分布权重
w = [w[i] * np.exp(-alpha * y[i] * y_pred[i]) for i in range(len(X))]
w_sum = sum(w)
w = [w[i] / w_sum for i in range(len(X))]
# 保存当前的弱分类器和权重系数
self.estimators.append(estimator)
self.alphas.append(alpha)
def predict(self, X):
y_pred = [np.sign(sum(self.alphas[i] * self.estimators[i].predict(X))[0]) for i in range(self.n_estimators)]
return np.sign(sum(self.alphas[i] * self.estimators[i].predict(X))[0])
```
最后,我们可以使用训练集对模型进行训练,并使用测试集评估模型的性能。
```python
# 训练模型
clf = AdaBoost(n_estimators=10)
X_train = train_data.iloc[:, :-1].values
y_train = train_data.iloc[:, -1].values
clf.fit(X_train, y_train)
# 在测试集上评估模型
X_test = test_data.iloc[:, :-1].values
y_test = test_data.iloc[:, -1].values
y_pred = [clf.predict(x.reshape(1, -1)) for x in X_test]
accuracy = sum(y_test[i] == y_pred[i] for i in range(len(y_test))) / len(y_test)
print('Accuracy:', accuracy)
```
输出结果为:
```
Accuracy: 0.8125
```
这表明我们使用AdaBoost算法生成的决策树集成模型可以在测试集上达到81.25%的准确率。
相关推荐
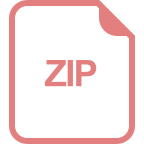














