pycharm实现目标检并逐帧保存
时间: 2023-11-05 09:00:39 浏览: 41
以下是基本的步骤,可以实现在PyCharm中进行目标检测并逐帧保存:
1. 安装OpenCV和其他必要的库。
在PyCharm中创建一个新的Python项目,并确保安装了OpenCV和其他必要的库。您可以使用pip来安装它们,例如:
```
pip install opencv-python
pip install numpy
pip install imutils
```
2. 加载视频并设置输出路径。
使用OpenCV加载要检测的视频,然后设置输出路径以保存检测到的帧。例如,以下代码加载名为“video.mp4”的视频,并将检测到的帧保存在名为“output”目录中:
```
import cv2
import os
# Load the video
cap = cv2.VideoCapture('video.mp4')
# Create the output directory if it does not exist
if not os.path.exists('output'):
os.makedirs('output')
# Set the output path for each frame
output_path = os.path.join('output', 'frame_{}.jpg')
```
3. 定义目标检测函数。
定义一个函数来执行目标检测并返回检测到的帧。这里使用了Haar级联检测器,但您可以使用任何其他类型的检测器。以下是一个简单的函数,它将每个检测到的帧保存到输出路径中:
```
def detect_objects(frame):
# Convert the frame to grayscale
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# Load the Haar cascade classifier
detector = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
# Detect faces in the frame
faces = detector.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# Draw a rectangle around each face and save the frame
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imwrite(output_path.format(cap.get(cv2.CAP_PROP_POS_FRAMES)), frame)
# Return the frame
return frame
```
4. 逐帧处理视频。
使用一个while循环,逐帧读取视频并将每个帧传递给目标检测函数。以下是一个简单的代码示例:
```
while True:
# Read a frame from the video
ret, frame = cap.read()
# Break the loop if the video has ended
if not ret:
break
# Perform object detection on the frame
frame = detect_objects(frame)
# Display the frame
cv2.imshow('frame', frame)
# Press 'q' to quit
if cv2.waitKey(1) == ord('q'):
break
```
5. 清理并关闭所有窗口。
最后,记得在完成处理后清理和关闭所有窗口和对象:
```
# Release the video capture object and destroy all windows
cap.release()
cv2.destroyAllWindows()
```
这些是基本的步骤,可以实现在PyCharm中进行目标检测并逐帧保存。您可以根据需要进行更改和定制。
相关推荐
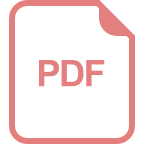














