C++一行字符(最多10个)以*号结尾(不包含空格),将这若干个字符按照asc码从小到大输出(不包括*号)。
时间: 2023-08-01 08:06:04 浏览: 182
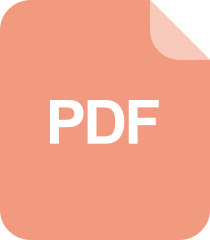
javascript将字符串中的多个空格替换为一个空格的正则实例
可以使用C++的string和algorithm库来实现这个功能。具体代码如下:
```
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
int main() {
string s;
cin >> s;
s.erase(s.end() - 1); // 去掉末尾的*
sort(s.begin(), s.end()); // 按照asc码从小到大排序
for (int i = 0; i < s.size(); i++) {
cout << s[i];
}
cout << endl;
return 0;
}
```
输入的字符串会被存在变量s中,然后使用erase函数去掉末尾的*号,再使用sort函数对字符串进行排序,最后按照顺序输出即可。
阅读全文
相关推荐
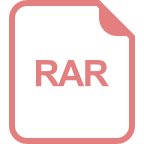















