54321的倒计时前端
时间: 2023-10-18 11:14:55 浏览: 91
这是一个简单的倒计时前端实现,使用 HTML、CSS 和 JavaScript 编写。
HTML 代码:
```
<!DOCTYPE html>
<html>
<head>
<title>倒计时</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="countdown">
<div class="days">
<span class="number" id="days"></span>
<span class="label">天</span>
</div>
<div class="hours">
<span class="number" id="hours"></span>
<span class="label">小时</span>
</div>
<div class="minutes">
<span class="number" id="minutes"></span>
<span class="label">分钟</span>
</div>
<div class="seconds">
<span class="number" id="seconds"></span>
<span class="label">秒</span>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
```
CSS 代码:
```
body {
font-family: Arial, sans-serif;
text-align: center;
}
.countdown {
display: flex;
justify-content: center;
align-items: center;
margin-top: 50px;
}
.number {
display: block;
font-size: 60px;
font-weight: bold;
margin-bottom: 10px;
}
.label {
font-size: 20px;
font-weight: bold;
}
.days {
margin-right: 50px;
}
.hours,
.minutes,
.seconds {
margin-right: 30px;
}
```
JavaScript 代码:
```
// 目标日期,格式为 "年-月-日 时:分:秒"
const targetDate = "2022-01-01 00:00:00";
// 获取倒计时元素
const daysElement = document.getElementById("days");
const hoursElement = document.getElementById("hours");
const minutesElement = document.getElementById("minutes");
const secondsElement = document.getElementById("seconds");
// 更新倒计时
function updateCountdown() {
// 计算目标日期和当前日期之间的时间差
const timeDiff = new Date(targetDate) - new Date();
// 如果目标日期已过,则停止倒计时
if (timeDiff < 0) {
clearInterval(countdownInterval);
return;
}
// 计算天数、小时数、分钟数和秒数
const days = Math.floor(timeDiff / (1000 * 60 * 60 * 24));
const hours = Math.floor((timeDiff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((timeDiff % (1000 * 60 * 60)) / (1000 * 60));
const seconds = Math.floor((timeDiff % (1000 * 60)) / 1000);
// 更新倒计时元素的内容
daysElement.textContent = days;
hoursElement.textContent = hours.toString().padStart(2, "0");
minutesElement.textContent = minutes.toString().padStart(2, "0");
secondsElement.textContent = seconds.toString().padStart(2, "0");
}
// 首次更新倒计时
updateCountdown();
// 每秒更新倒计时
const countdownInterval = setInterval(updateCountdown, 1000);
```
代码解释:
1. HTML 中包含一个倒计时容器(`.countdown`),其中包含四个子元素,分别对应天数、小时数、分钟数和秒数的显示。
2. CSS 中对倒计时容器和子元素进行样式设置。
3. JavaScript 中定义了目标日期、倒计时元素和更新倒计时的函数。
4. `updateCountdown` 函数首先计算目标日期和当前日期之间的时间差,然后根据时间差计算天数、小时数、分钟数和秒数,最后将这些值更新到倒计时元素中。
5. 首次更新倒计时需要调用一次 `updateCountdown` 函数。
6. 每秒更新倒计时需要使用 `setInterval` 函数来定时调用 `updateCountdown` 函数,并将返回值保存在 `countdownInterval` 变量中,以便在目标日期已过时停止倒计时。
阅读全文
相关推荐
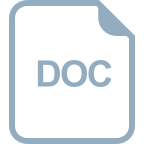
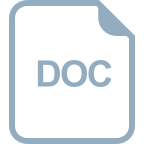
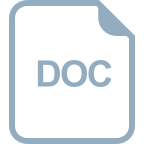
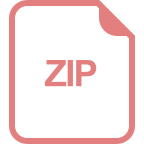
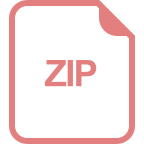
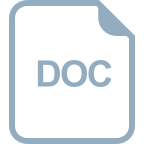
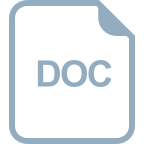
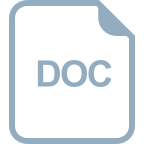
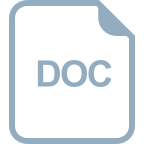
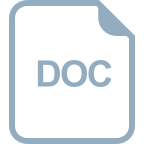
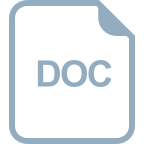
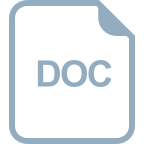




