c#窗体应用程序实现软件开机自启,并可以通过路径控制ICT测试软件的打开和关闭。 良品和不良品条码可以在本地文件夹的txt文件中获取 。当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天ICT测试记录,如果存在,lable状态栏变绿并提示“以点检,请进行测试”就可以通过c#软件开启按钮打开ICT测试软件,进行别的条码的ICT测试。如果没有,点击开启ICT测试按钮时,label状态栏变红,并提示“请进行测试前点检”全部代码实现
时间: 2023-12-01 07:02:06 浏览: 367
以下是实现软件开机自启的代码:
1. 在项目中添加一个应用程序配置文件 app.config,添加一个 key 值为 AutoStartPath 的配置项,用于保存ICT测试软件的路径。
2. 在程序启动时,读取配置文件中的 AutoStartPath,判断是否存在该路径下的文件,如果存在,则打开该文件,否则不做任何操作。
```c#
private void Form1_Load(object sender, EventArgs e)
{
// 读取配置文件中的路径
string autoStartPath = ConfigurationManager.AppSettings["AutoStartPath"].ToString();
if (!string.IsNullOrEmpty(autoStartPath) && File.Exists(autoStartPath))
{
// 打开ICT测试软件
Process.Start(autoStartPath);
}
}
```
以下是实现通过路径控制ICT测试软件的打开和关闭的代码:
1. 在窗体中添加两个按钮 btnOpen 和 btnClose,用于打开和关闭ICT测试软件。
2. 在 btnOpen 的 Click 事件中,读取配置文件中的 AutoStartPath,判断是否存在该路径下的文件,如果存在,则打开该文件,否则提示用户选择文件路径。
```c#
private void btnOpen_Click(object sender, EventArgs e)
{
// 读取配置文件中的路径
string autoStartPath = ConfigurationManager.AppSettings["AutoStartPath"].ToString();
if (!string.IsNullOrEmpty(autoStartPath) && File.Exists(autoStartPath))
{
// 打开ICT测试软件
Process.Start(autoStartPath);
}
else
{
// 提示用户选择文件路径
OpenFileDialog openFileDialog = new OpenFileDialog();
openFileDialog.Filter = "Executable Files (*.exe)|*.exe";
openFileDialog.Title = "选择ICT测试软件";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
// 保存用户选择的路径
autoStartPath = openFileDialog.FileName;
Configuration config = ConfigurationManager.OpenExeConfiguration(ConfigurationUserLevel.None);
config.AppSettings.Settings["AutoStartPath"].Value = autoStartPath;
config.Save(ConfigurationSaveMode.Modified);
ConfigurationManager.RefreshSection("appSettings");
// 打开ICT测试软件
Process.Start(autoStartPath);
}
}
}
```
3. 在 btnClose 的 Click 事件中,通过 Process.GetProcessesByName 方法获取所有进程名为 ICTTest 的进程,遍历进程数组,逐个关闭进程。
```c#
private void btnClose_Click(object sender, EventArgs e)
{
Process[] processes = Process.GetProcessesByName("ICTTest");
foreach (Process process in processes)
{
process.Kill();
}
}
```
以下是实现定时器扫描数据库并提示用户的代码:
1. 在窗体中添加一个标签 label1,用于提示用户点检状态。
2. 在窗体中添加一个定时器 timer1,设置 Interval 为 5000(5 秒),启动定时器。
3. 在 timer1 的 Tick 事件中,查询数据库中是否存在当天的良品和不良品条码的ICT测试记录,如果存在,将 label1 的文本设置为“以点检,请进行测试”,并将 label1 的前景色设置为绿色,否则将 label1 的文本设置为“请进行测试前点检”,并将 label1 的前景色设置为红色。
```c#
private void timer1_Tick(object sender, EventArgs e)
{
string today = DateTime.Now.ToString("yyyy-MM-dd");
// 查询当天的良品和不良品条码的ICT测试记录
bool hasRecord = false; // 是否存在记录
// TODO: 查询数据库的代码
if (hasRecord)
{
label1.Text = "以点检,请进行测试";
label1.ForeColor = Color.Green;
}
else
{
label1.Text = "请进行测试前点检";
label1.ForeColor = Color.Red;
}
}
```
阅读全文
相关推荐
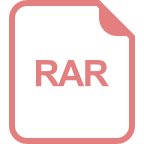
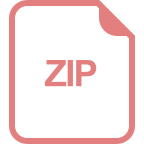
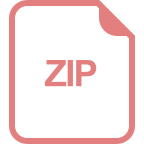





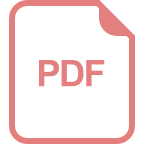
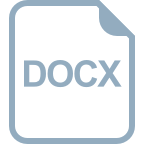
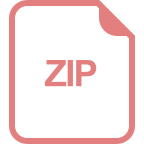
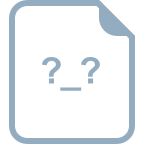
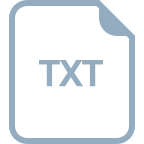
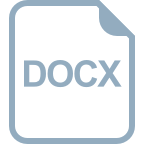
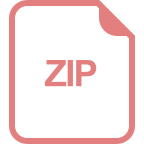