C++中,定义函数int function(int a[], int b),这里数组a会不会在内存中拷贝(传递的是指针还是啥),什么情况下传递的是指针?
时间: 2023-03-31 16:03:47 浏览: 140
数组a在内存中传递的是指针,即传递的是数组a的首地址。当数组作为函数参数传递时,通常会自动转换为指向数组首元素的指针。因此,在定义函数int function(int a[], int b)时,数组a并不会在内存中拷贝,而是传递的是指针。
相关问题
2.改成单继承 class A { public: A(int num):data(num) {} void AFuncs() {cout<<"This is A \'s public function!"<<endl;} protected: int data; }; class B { public: B(int num):value(num) {} void BFuncs() {cout<<"This is B \'s public function!"<<endl;} protected: int value; }; class C:public A,private B { public: C(int num1,int num2,int y); void MyFuncs() { BFuncs(); cout<<"This function call B::BFuncs() !"<<endl; } private: int yyy; }; 1)完成C类的构造函数。 2)在main 函数中可以向C类对象发送哪些消息? 3)在不改变C类的功能的条件下,利用类的组合重新定义并实现C类,使其变成单继承。 4)实现新的C类的构造,析构,拷贝、赋值函数。
1) 完成C类的构造函数。
```c++
C::C(int num1, int num2, int y): A(num1), B(num2), yyy(y) {}
```
2) 在main函数中可以向C类对象发送以下消息:
- AFuncs():调用A类的公有函数。
- MyFuncs():调用C类的公有函数。
3) 在不改变C类的功能的条件下,利用类的组合重新定义并实现C类,使其变成单继承。
```c++
class C {
public:
C(int num1, int num2, int y);
void AFuncs() { a.AFuncs(); }
void MyFuncs() { b.BFuncs(); cout << "This function call B::BFuncs() !" << endl; }
private:
A a;
B b;
int yyy;
};
```
4) 实现新的C类的构造、析构、拷贝、赋值函数。
```c++
C::C(int num1, int num2, int y): a(num1), b(num2), yyy(y) {}
C::~C() {}
C::C(const C& other): a(other.a), b(other.b), yyy(other.yyy) {}
C& C::operator=(const C& other) {
if (this != &other) {
a = other.a;
b = other.b;
yyy = other.yyy;
}
return *this;
}
```
c++ 函数传递时,如何处理vector<int>
在C++中,当你需要将`vector<int>`作为参数传递给函数时,有几种常见的处理方式:
1. **值传递**(Pass by Value):
如果你只是想把`vector<int>`作为一个副本传递,可以像处理其他基本数据类型一样,直接传递。函数内的修改不会影响到原变量。
```cpp
void function(vector<int>& vec) {
// 在这里对vec操作...
}
int main() {
vector<int> myVec = {1, 2, 3};
function(myVec);
// myVec仍然是{1, 2, 3},因为myVec是个副本
}
```
2. **引用传递**(Pass by Reference):
如果你想让函数能够直接访问并修改原始向量,你应该传入引用(&)。这样函数内部的操作会对原始`vector`产生影响。
```cpp
void function(vector<int>&& vec) { // C++11开始支持Rvalue引用
// 在这里可以直接修改vec
vec.push_back(4);
}
int main() {
vector<int> myVec = {1, 2, 3};
function(myVec); // 现在myVec变为{1, 2, 3, 4}
}
```
3. **指针传递**(Pass by Pointer):
也可以通过指向vector的指针传递,但这相对少用,因为C++已经提供了更方便的引用语法。不过,在某些特定场景下(如大量数据、避免拷贝),可能考虑使用智能指针(如unique_ptr)。
```cpp
void function(vector<int>* vec) {
// 使用*vec访问元素
(*vec).push_back(4);
}
int main() {
vector<int> myVec = {1, 2, 3};
function(&myVec); // 注意,这里是地址(&)
}
```
阅读全文
相关推荐
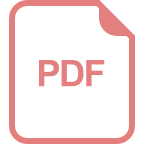
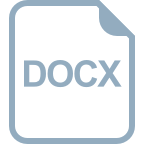
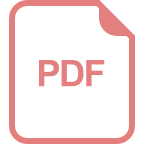
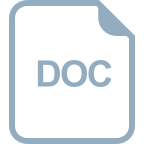
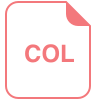
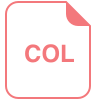
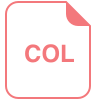
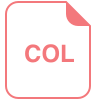
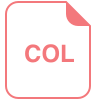
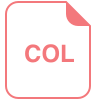
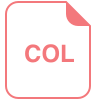
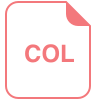
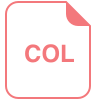
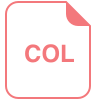
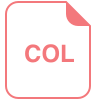