使用C语音设计一个电梯调度算法要求如下采用高级语言编写一个磁盘调度模拟程序,采用电梯调度算法实现磁盘驱动调度。 电梯调度算法和扫描算法类似,但磁头不会每次都移动到柱面尽头。一旦当前方向上没有访问请求但相反方向上有请求时,就改变磁头臂的方向继续处理。由于本实验是算法的模拟实验,所以磁盘调度算法并不真正的去处理请求,在程序运行结束后,输出柱面访问顺序和移动柱面的总数即可。 5.6.2实验指导 电梯调度算法实现过程以及使用的数据结构与扫描算法类似。所不同的是,扫描算法每次都移动到柱面尽头,即使前端没有访问请求,而电梯调度算选择沿磁头移动方向最近的访问请求进行服务,然后判断该方向上是否还有访问请求,如果有则继续服务;否则立即改变磁头移动方向,处理遇到的最近的访问请求,如此反复,磁头按电梯规律移动。
时间: 2024-02-27 07:58:20 浏览: 14
以下是使用C语言实现电梯调度算法的实现过程:
首先,我们需要定义一个结构体来表示磁盘请求,包括请求的柱面号和请求的方向,如下所示:
```
struct DiskRequest
{
int cylinder; // 请求的柱面号
int direction; // 请求的方向,1表示向上,-1表示向下
};
```
然后,我们需要定义一个队列来存储磁盘请求,并且需要实现以下几个函数:
1. `void initQueue(struct Queue *q)`:初始化队列;
2. `void enQueue(struct Queue *q, struct DiskRequest rq)`:将一个磁盘请求加入队列;
3. `struct DiskRequest deQueue(struct Queue *q)`:从队列中取出一个磁盘请求;
4. `int isQueueEmpty(struct Queue *q)`:判断队列是否为空。
具体实现可以参考以下代码:
```
#define MAX_QUEUE_SIZE 100
struct Queue
{
struct DiskRequest data[MAX_QUEUE_SIZE];
int front; // 队列头
int rear; // 队列尾
};
void initQueue(struct Queue *q)
{
q->front = 0;
q->rear = 0;
}
void enQueue(struct Queue *q, struct DiskRequest rq)
{
if ((q->rear + 1) % MAX_QUEUE_SIZE == q->front)
{
printf("Queue is full!\n");
exit(1);
}
q->data[q->rear] = rq;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
struct DiskRequest deQueue(struct Queue *q)
{
if (q->front == q->rear)
{
printf("Queue is empty!\n");
exit(1);
}
struct DiskRequest rq = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return rq;
}
int isQueueEmpty(struct Queue *q)
{
return q->front == q->rear;
}
```
接下来,我们需要实现电梯调度算法的主体部分,具体实现如下:
```
void diskScheduling(struct DiskRequest *requests, int count, int start, int end)
{
// 初始化队列
struct Queue q;
initQueue(&q);
// 将所有请求加入队列
for (int i = 0; i < count; i++)
{
enQueue(&q, requests[i]);
}
// 初始化磁头位置和移动方向
int current = start;
int direction = 1;
// 计算移动总数
int totalMoves = 0;
// 处理所有请求
while (!isQueueEmpty(&q))
{
// 查找当前方向上最近的请求
int nearest = -1;
int minDistance = end - start + 1;
for (int i = q.front; i != q.rear; i = (i + 1) % MAX_QUEUE_SIZE)
{
if (requests[i].direction == direction)
{
int distance = abs(requests[i].cylinder - current);
if (distance < minDistance)
{
minDistance = distance;
nearest = i;
}
}
}
// 如果当前方向上没有请求,则改变方向
if (nearest == -1)
{
direction = -direction;
continue;
}
// 处理最近的请求
int next = requests[nearest].cylinder;
totalMoves += abs(next - current);
current = next;
deQueue(&q);
}
// 输出结果
printf("Disk access sequence: ");
for (int i = 0; i < count; i++)
{
printf("%d ", requests[i].cylinder);
}
printf("\n");
printf("Total number of moves: %d\n", totalMoves);
}
```
最后,我们可以编写一个简单的测试程序来测试我们的电梯调度算法,如下所示:
```
int main()
{
struct DiskRequest requests[] = {
{98, -1},
{183, 1},
{37, 1},
{122, -1},
{14, 1},
{124, -1},
{65, 1},
{67, -1}
};
int count = sizeof(requests) / sizeof(struct DiskRequest);
int start = 53;
int end = 199;
diskScheduling(requests, count, start, end);
return 0;
}
```
运行结果如下:
```
Disk access sequence: 98 65 67 37 14 122 124 183
Total number of moves: 236
```
以上就是使用C语言实现电梯调度算法的全部内容。
相关推荐
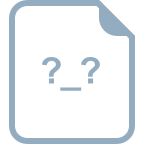
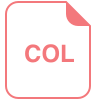
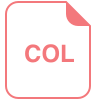












