spring boot打印接口入参,出参,其中入参中可能会包含MultipartFile、,入参也有可能是个实体类,实体类中也有可能包含MultipartFile集合的具体代码
时间: 2023-08-26 22:08:30 浏览: 220
### 回答1:
您可以通过使用AOP(面向切面编程)来实现对Spring Boot接口的入参和出参进行打印。
首先,定义一个切面类,在该类中编写打印代码:
```
@Aspect
@Component
public class LogAspect {
private static final Logger LOGGER = LoggerFactory.getLogger(LogAspect.class);
@Pointcut("execution(public * com.example.controller.*.*(..))")
public void log() {}
@Before("log()")
public void doBefore(JoinPoint joinPoint) throws Throwable {
ServletRequestAttributes attributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = attributes.getRequest();
LOGGER.info("请求URL : {}", request.getRequestURL().toString());
LOGGER.info("请求方法 : {}", request.getMethod());
LOGGER.info("请求IP : {}", request.getRemoteAddr());
LOGGER.info("请求入参 : {}", Arrays.toString(joinPoint.getArgs()));
}
@AfterReturning(returning = "ret", pointcut = "log()")
public void doAfterReturning(Object ret) throws Throwable {
LOGGER.info("请求出参 : {}", ret);
}
}
```
其中,使用@Pointcut注解定义切入点,在本例中为所有在com.example.controller包下的方法。
使用@Before注解定义在切入点之前执行的操作,在本例中为打印请求URL、请求方法、请求IP和请求入参。
使用@AfterReturning注解定义在切入点之后执行的操作,在本例中为打印请求出参。
对于入参中包含MultipartFile类型的情况,您可以使用instanceof进行判断,并对MultipartFile进行特殊处理。例如:
```
for (Object arg : joinPoint.getArgs()) {
if (arg instanceof MultipartFile) {
### 回答2:
在Spring Boot中,可以通过一些方式来打印接口的入参和出参。
首先,我们可以在方法的参数中使用`@RequestBody`注解来接收请求的入参,该注解可以将请求的JSON数据转换成对应的实体类对象。如果实体类中包含了`MultipartFile`类型的属性,可以通过以下代码处理:
```java
@PostMapping("/upload")
public void uploadFile(@RequestParam("file") MultipartFile file) throws IOException {
// 打印入参
System.out.println("入参:");
System.out.println("文件名:" + file.getOriginalFilename());
System.out.println("文件类型:" + file.getContentType());
System.out.println("文件大小:" + file.getSize());
// 具体的文件处理逻辑
// ...
}
```
上述代码中,`@RequestParam("file")`用于获取请求参数中名为`file`的`MultipartFile`类型对象,并打印相关的属性信息。
另外,对于入参是实体类的情况,同样可以通过`@RequestBody`注解来接收请求的JSON数据并转换成对应的实体类对象。如果实体类中包含了`MultipartFile`类型的集合属性,可以通过以下代码处理:
```java
@PostMapping("/upload")
public void uploadFiles(@RequestBody Entity entity) {
// 打印入参
System.out.println("入参:");
System.out.println("属性1:" + entity.getProperty1());
System.out.println("属性2:" + entity.getProperty2());
// 打印MultipartFile集合中的属性信息
List<MultipartFile> files = entity.getFiles();
for (MultipartFile file : files) {
System.out.println("文件名:" + file.getOriginalFilename());
System.out.println("文件类型:" + file.getContentType());
System.out.println("文件大小:" + file.getSize());
// 具体的文件处理逻辑
// ...
}
}
```
上述代码中,`Entity`即为实体类,其中包含了名为`files`的`MultipartFile`集合属性。通过遍历集合中的每个`MultipartFile`对象,可以打印相关的属性信息。
综上所述,通过上述代码可以在Spring Boot中打印接口的入参和出参,包括处理包含`MultipartFile`和实体类的情况。
### 回答3:
在Spring Boot中打印接口入参和出参可以通过切面编程来实现。首先需要创建一个切面类,用于处理日志的打印。
```java
@Aspect
@Component
public class LogAspect {
private Logger logger = LoggerFactory.getLogger(LogAspect.class);
@Pointcut("execution(* com.example.controller.*.*(..))")
public void controllerPointcut() {}
@Before("controllerPointcut()")
public void logRequest(JoinPoint joinPoint) {
// 打印接口入参
logger.info("接口入参: {}", Arrays.toString(joinPoint.getArgs()));
}
@AfterReturning(returning = "result", pointcut = "controllerPointcut()")
public void logResponse(Object result) {
// 打印接口出参
logger.info("接口出参: {}", result);
}
}
```
在切面类中,通过`@Before`注解的方式,在方法执行前打印接口的入参;通过`@AfterReturning`注解的方式,在方法返回后打印接口的出参。
如果接口入参中包含`MultipartFile`,可以在切面类中获取其相关信息并打印出来。
实体类中包含`MultipartFile`集合的情况,可以通过增加一段逻辑来判断并处理打印。例如:
```java
if (arg instanceof MultipartFile) {
MultipartFile file = (MultipartFile) arg;
logger.info("文件名: {}", file.getOriginalFilename());
}
```
以上代码判断入参是否为`MultipartFile`类型,如果是的话,则打印文件名。
需要注意的是,切面类需要添加`@Aspect`和`@Component`注解,用于声明该类为切面类,并将其纳入Spring容器管理。
另外,需要在Spring Boot的配置类中添加`@EnableAspectJAutoProxy`注解,启用切面的自动代理功能。
以上是一个简单示例,可以根据实际需求进行扩展和调整。希望对你有所帮助!
阅读全文
相关推荐
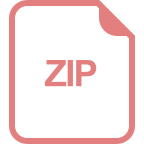
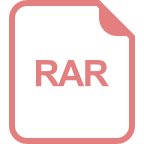
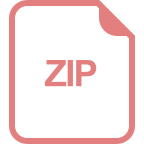

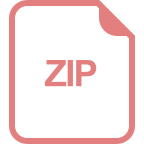
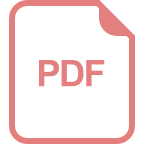
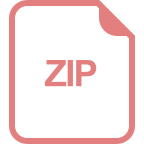
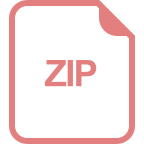
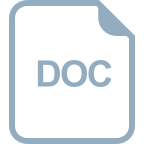
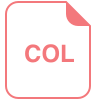
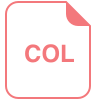
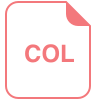
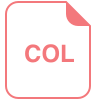





