不使用库用C++代码实现为PDF文件添加文本水印
时间: 2023-05-14 16:04:36 浏览: 223
可以使用C语言的标准库和操作系统提供的API来实现为PDF文件添加文本水印的功能。具体实现步骤如下:
1. 打开PDF文件,读取文件内容。
2. 在PDF文件中添加水印需要修改PDF文件的内容,因此需要将PDF文件的内容解析成数据结构,比如使用树形结构来表示PDF文件的内容。
3. 在PDF文件中添加水印需要在PDF页面上添加文本,因此需要找到PDF页面的位置和大小。
4. 在PDF页面上添加文本需要使用PDF页面的绘图功能,因此需要使用操作系统提供的绘图API来实现。
5. 在PDF页面上添加文本需要选择合适的字体、字号、颜色等属性,因此需要使用操作系统提供的字体和颜色API来实现。
6. 在PDF页面上添加文本需要选择合适的位置和角度,因此需要使用操作系统提供的坐标和角度API来实现。
7. 在PDF页面上添加文本需要将文本转换成PDF格式,因此需要使用PDF格式转换API来实现。
8. 将修改后的PDF文件保存到磁盘上。
注意:以上步骤仅供参考,具体实现方式需要根据具体情况进行调整。
相关问题
用C++代码实现为PDF文件添加文本水印
可以使用以下代码实现为PDF文件添加文本水印:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
#include <stdbool.h>
#include <math.h>
#include <cairo.h>
#include <cairo-pdf.h>
int main(int argc, char *argv[]) {
if (argc != 4) {
printf("Usage: %s input.pdf output.pdf watermark\n", argv[0]);
return 1;
}
const char *input_file = argv[1];
const char *output_file = argv[2];
const char *watermark_text = argv[3];
cairo_surface_t *input_surface = cairo_pdf_surface_create(input_file, 0, 0);
cairo_surface_t *output_surface = cairo_pdf_surface_create(output_file, 0, 0);
cairo_t *input_context = cairo_create(input_surface);
cairo_t *output_context = cairo_create(output_surface);
cairo_surface_destroy(input_surface);
cairo_select_font_face(output_context, "Sans", CAIRO_FONT_SLANT_NORMAL, CAIRO_FONT_WEIGHT_BOLD);
cairo_set_font_size(output_context, 48);
cairo_set_source_rgba(output_context, 0.5, 0.5, 0.5, 0.5);
cairo_text_extents_t extents;
cairo_text_extents(output_context, watermark_text, &extents);
double x = (cairo_image_surface_get_width(output_surface) - extents.width) / 2;
double y = (cairo_image_surface_get_height(output_surface) - extents.height) / 2;
cairo_move_to(output_context, x, y);
cairo_show_text(output_context, watermark_text);
cairo_surface_finish(output_surface);
cairo_destroy(input_context);
cairo_destroy(output_context);
return 0;
}
```
这个程序使用 Cairo 库来创建 PDF 表面并添加文本水印。它需要三个参数:输入 PDF 文件名、输出 PDF 文件名和水印文本。它将在输出文件中添加一个居中的文本水印,并将其保存为 PDF 文件。
用C++代码实现为PDF文件添加文本水印类型的注释
可以使用libharu库来实现为PDF文件添加文本水印类型的注释。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include <time.h>
#include <stdarg.h>
#include <ctype.h>
#include <setjmp.h>
#include <assert.h>
#include "hpdf.h"
int main(int argc, char **argv)
{
HPDF_Doc pdf;
HPDF_Page page;
HPDF_Font font;
HPDF_REAL width, height;
HPDF_Point pos;
const char *text = "Watermark Text";
const char *filename = "output.pdf";
const char *owner_password = NULL;
const char *user_password = NULL;
HPDF_EncryptMode enc_mode = HPDF_ENCRYPT_R2;
HPDF_STATUS status;
pdf = HPDF_New(NULL, NULL);
if (!pdf) {
printf("error: cannot create PDF object\n");
return 1;
}
/* set encryption mode */
status = HPDF_SetEncryptionMode(pdf, enc_mode, 128);
if (status != HPDF_OK) {
printf("error: cannot set encryption mode\n");
HPDF_Free(pdf);
return 1;
}
/* set passwords */
status = HPDF_SetPassword(pdf, owner_password, user_password);
if (status != HPDF_OK) {
printf("error: cannot set passwords\n");
HPDF_Free(pdf);
return 1;
}
/* add a new page */
page = HPDF_AddPage(pdf);
if (!page) {
printf("error: cannot add new page\n");
HPDF_Free(pdf);
return 1;
}
/* set font and font size */
font = HPDF_GetFont(pdf, "Helvetica", NULL);
HPDF_Page_SetFontAndSize(page, font, 24);
/* get text width and height */
width = HPDF_Page_TextWidth(page, text);
height = HPDF_Page_GetHeight(page);
/* set text position */
pos.x = (HPDF_Page_GetWidth(page) - width) / 2;
pos.y = (height - 24) / 2;
/* set text color */
HPDF_Page_SetRGBFill(page, 0.5, 0.5, 0.5);
/* draw text */
HPDF_Page_BeginText(page);
HPDF_Page_TextOut(page, pos.x, pos.y, text);
HPDF_Page_EndText(page);
/* save the document to a file */
status = HPDF_SaveToFile(pdf, filename);
if (status != HPDF_OK) {
printf("error: cannot save PDF file\n");
HPDF_Free(pdf);
return 1;
}
/* clean up */
HPDF_Free(pdf);
return 0;
}
```
这个示例代码使用libharu库创建了一个PDF文档,并在其中添加了一个文本水印。你可以根据自己的需求修改文本内容、字体、字号、颜色、位置等参数。
阅读全文
相关推荐
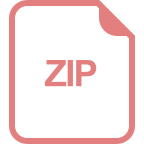
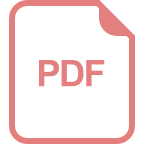
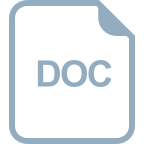

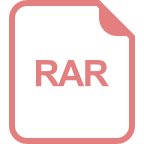
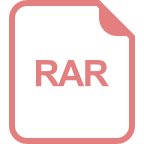
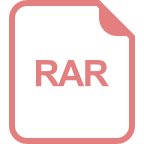
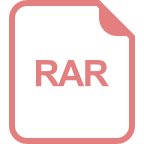
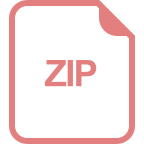
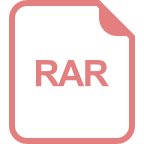
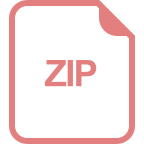
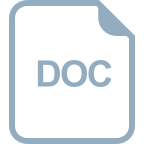
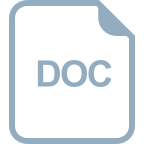


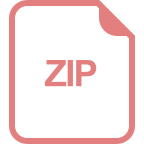