java post请求构造请求参数
时间: 2023-04-04 18:01:55 浏览: 85
可以使用Java中的HttpURLConnection类来构造post请求,并使用OutputStream将请求参数写入请求体中。具体实现可以参考以下代码:
```
URL url = new URL("http://example.com/api");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");
conn.setDoOutput(true);
conn.setDoInput(true);
conn.setUseCaches(false);
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
String params = "param1=value1¶m2=value2";
OutputStream out = conn.getOutputStream();
out.write(params.getBytes());
out.flush();
out.close();
InputStream in = conn.getInputStream();
// 处理响应数据
```
其中,params为请求参数,可以根据实际情况进行修改。另外,需要注意设置请求头中的Content-Type为application/x-www-form-urlencoded,表示请求体中的参数为表单形式。
相关问题
java post请求代码
以下是使用Java发送POST请求的示例代码:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
public class PostRequest {
public static void main(String[] args) {
try {
// 创建URL对象
URL url = new URL("http://example.com/api");
// 创建HttpURLConnection对象
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 设置请求方法
conn.setRequestMethod("POST");
// 设置请求属性
conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
// 启用输出流
conn.setDoOutput(true);
// 构造POST请求参数
String postData = "param1=" + URLEncoder.encode("value1", "UTF-8") +
"¶m2=" + URLEncoder.encode("value2", "UTF-8");
// 获取输出流
conn.getOutputStream().write(postData.getBytes("UTF-8"));
// 获取服务器响应
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String response;
while ((response = in.readLine()) != null) {
System.out.println(response);
}
in.close();
// 关闭连接
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
请注意,此代码仅供参考,并且需要替换URL和请求参数以匹配您的实际情况。
axios post请求传参数 后端 java接收
好的,您可以参考以下代码示例:
前端使用 axios 发送 post 请求传递参数:
```javascript
axios.post('/api/user', {
name: 'John',
age: 30
})
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
```
后端使用 Java 接收参数:
```java
@RestController
@RequestMapping("/api")
public class UserController {
@PostMapping("/user")
public String createUser(@RequestBody User user) {
String name = user.getName();
int age = user.getAge();
// 处理逻辑...
return "success";
}
}
class User {
private String name;
private int age;
// 必须提供无参构造函数
public User() {}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
```
在上面的示例中,我们定义了一个 User 类作为请求参数的数据模型,使用 @RequestBody 注解将请求体映射到 User 对象中,在方法中就可以对 User 对象进行处理。
相关推荐
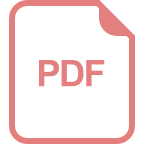












